Introduction to WebRTC Peer-to-Peer Video Call
WebRTC (Web Real-Time Communication) has revolutionized real-time communication by enabling web browsers and mobile applications to engage in audio and video communication without the need for plugins or native applications. This technology is the backbone of many modern video conferencing platforms and real-time collaboration tools. In this comprehensive guide, we will explore the intricacies of building a WebRTC peer-to-peer video call application, delving into its architecture, core concepts, and practical implementation.
What is WebRTC?
WebRTC is an open-source project providing web browsers and mobile applications with real-time communication (RTC) capabilities via simple APIs. It enables audio and video communication directly between browsers and devices, without requiring intermediaries. This opens the doors for creating engaging and interactive web applications.
The Power of Peer-to-Peer
The peer-to-peer (P2P) nature of WebRTC offers several advantages. P2P connections reduce latency, as data travels directly between users rather than through a central server. This direct communication model also enhances privacy and reduces bandwidth costs for the application provider.
Why Choose WebRTC for P2P Video Calls?
WebRTC provides a robust, standardized, and readily available solution for building peer-to-peer video call applications. It eliminates the complexities of managing media servers and proprietary protocols, allowing developers to focus on creating innovative user experiences. Furthermore, WebRTC's wide browser compatibility ensures that your application can reach a broad audience.
Understanding WebRTC Architecture
WebRTC architecture comprises several key components that work together to establish and maintain peer-to-peer connections. Understanding these components is crucial for building reliable and scalable video call applications.
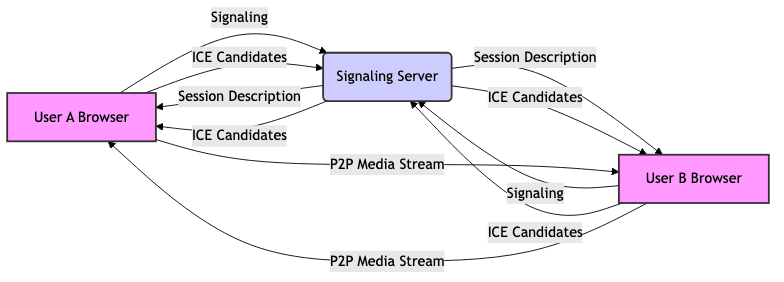
Signaling Servers: The Bridge Between Peers
WebRTC uses a signaling server to coordinate the initial handshake between peers. This server facilitates the exchange of metadata, such as session descriptions (SDP) and ICE candidates, enabling peers to establish a direct connection. The signaling server itself doesn't handle the actual media stream; its primary role is to facilitate the connection setup.
STUN, TURN, and ICE: Navigating Network Complexities
STUN (Session Traversal Utilities for NAT), TURN (Traversal Using Relays around NAT), and ICE (Interactive Connectivity Establishment) are protocols that help WebRTC navigate network address translators (NATs) and firewalls. ICE is a framework that uses STUN and TURN servers to find the best possible path for establishing a peer-to-peer connection. STUN allows peers to discover their public IP address, while TURN acts as a relay server when direct connections are not possible.
JavaScript
1const iceServers = [
2 { urls: 'stun:stun.l.google.com:19302' },
3 { urls: 'stun:stun1.l.google.com:19302' },
4 { urls: 'turn:your-turn-server.com:3478', username: 'your-username', credential: 'your-password' }
5];
6
Media Streams and Peer Connections
Once the signaling process is complete and ICE has established a suitable connection, media streams can flow directly between peers. The
RTCPeerConnection
API is the core of WebRTC, providing the methods and events needed to establish and manage these connections. Media streams, represented by MediaStream
objects, carry the audio and video data.Building a Simple WebRTC Peer-to-Peer Video Call
Let's walk through the process of building a basic WebRTC peer-to-peer video call application.
Setting up the Development Environment
To start, you'll need a text editor, a web browser that supports WebRTC (most modern browsers do), and a simple web server to host your HTML, CSS, and JavaScript files. You can use tools like Node.js with
http-server
or Python's built-in http.server
module.Obtaining Media Access
The first step is to obtain access to the user's camera and microphone using the
getUserMedia()
API. This function prompts the user for permission to access their media devices.JavaScript
1navigator.mediaDevices.getUserMedia({ video: true, audio: true })
2 .then(stream => {
3 // Handle the stream
4 const localVideo = document.getElementById('localVideo');
5 localVideo.srcObject = stream;
6 })
7 .catch(error => {
8 console.error('Error accessing media devices:', error);
9 });
10
Establishing a Peer Connection
Next, you'll need to create an
RTCPeerConnection
object. This object is responsible for managing the peer-to-peer connection and handling the exchange of media streams.JavaScript
1const peerConnection = new RTCPeerConnection({
2 iceServers: iceServers
3});
4
5stream.getTracks().forEach(track => {
6 peerConnection.addTrack(track, stream);
7});
8
9peerConnection.ontrack = event => {
10 const remoteVideo = document.getElementById('remoteVideo');
11 remoteVideo.srcObject = event.streams[0];
12};
13
Handling Signaling
Signaling is crucial for coordinating the connection between peers. You'll need a signaling server to facilitate the exchange of SDP offers, answers, and ICE candidates. This can be implemented using WebSockets or any other real-time communication technology. After a peer connection is initiated an offer needs to be generated and the other end returns an answer. Both parties gather ICE candidates for NAT traversal.
Advanced WebRTC Concepts
To build production-ready WebRTC applications, you need to understand some advanced concepts.
Handling ICE Candidates
ICE candidates are potential network paths that can be used to establish a peer-to-peer connection. The
RTCPeerConnection
object emits icecandidate
events as it discovers these candidates. You need to send these candidates to the other peer via the signaling server.Optimizing for Performance and Scalability
Optimizing WebRTC applications for performance and scalability involves techniques such as using simulcast (sending multiple video streams at different resolutions), adjusting bandwidth constraints, and implementing efficient signaling mechanisms.
Addressing Security Concerns
WebRTC incorporates several security features, including encryption and authentication. However, it's important to follow best practices, such as using secure signaling channels (HTTPS/WSS) and validating user identities, to prevent security vulnerabilities. It is also important to use DTLS for encryption.
Error Handling and Robustness
Implement comprehensive error handling to gracefully handle network errors, media device failures, and other unexpected issues. Provide informative error messages to the user and implement retry mechanisms when appropriate.
WebRTC and its Applications
WebRTC has a wide range of applications beyond simple video calls.
Video Conferencing and Collaboration
WebRTC is the foundation of many modern video conferencing platforms, enabling seamless collaboration between remote teams. Applications include screen sharing, whiteboarding, and real-time document editing.
Live Streaming and Broadcasting
WebRTC can be used for live streaming and broadcasting applications, allowing users to stream video content to a large audience in real-time. Examples include live webinars, online gaming, and virtual events.
Other Use Cases
Other use cases for WebRTC include remote support, telehealth, augmented reality applications, and IoT devices.
Choosing the Right WebRTC Framework or Library
Several WebRTC frameworks and libraries can simplify the development process.
Evaluating Popular Options
Popular WebRTC frameworks and libraries include Janus, Kurento, Jitsi Videobridge, and simple-peer. Each option has its own strengths and weaknesses, so it's important to evaluate them based on your specific needs.
Factors to Consider
Factors to consider when choosing a WebRTC framework or library include its features, performance, scalability, ease of use, and community support.
Recommendations based on project needs
For simple peer-to-peer applications,
simple-peer
might be sufficient. For more complex applications requiring advanced features like media server integration, Janus or Kurento may be more appropriate. Jitsi Videobridge is a good option for building scalable video conferencing platforms.The Future of WebRTC
WebRTC continues to evolve, with new features and capabilities being added regularly.
Emerging Trends and Advancements
Emerging trends in WebRTC include support for new codecs (like AV1), improved scalability, and integration with emerging technologies like artificial intelligence and machine learning.
Potential Challenges and Solutions
Potential challenges for WebRTC include maintaining browser compatibility, addressing security concerns, and optimizing for low-bandwidth environments. Solutions include using feature detection, implementing robust security measures, and utilizing adaptive bitrate streaming.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ