WebRTC (Web Real-Time Communication) has revolutionized the way we build real-time communication applications. This guide provides a comprehensive overview of WebRTC application development, covering everything from the fundamentals to advanced concepts.
Introduction to WebRTC Application Development
WebRTC empowers developers to create rich, real-time communication experiences directly within web browsers and mobile applications. It eliminates the need for plugins or native downloads, streamlining the user experience and simplifying development.
What is WebRTC?
WebRTC is a free, open-source project providing web browsers and mobile applications with real-time communication (RTC) capabilities via simple APIs. It allows audio and video communication to work inside web pages by allowing direct peer-to-peer communication, eliminating the need for installing plugins or downloading native apps. WebRTC uses protocols like Session Description Protocol (SDP), Interactive Connectivity Establishment (ICE), and others to establish and maintain real-time connections.
Benefits of Using WebRTC
WebRTC offers several key advantages:
- No Plugins Required: Operates directly within the browser, avoiding plugin installations.
- Open Source and Free: Reduces development costs and fosters community contributions.
- Real-Time Communication: Enables low-latency audio and video transmission.
- Cross-Platform Compatibility: Works across various browsers and operating systems.
- Security: Employs encryption to protect communication data.
- Peer-to-Peer: Allows for direct communication between browsers and devices reducing bandwidth costs and latency.
WebRTC Architecture Overview
WebRTC architecture consists of several key components working together to establish and maintain real-time communication channels. Here's a simplified overview:
- Signaling: Exchanging metadata to coordinate communication (e.g., session descriptions, ICE candidates). This is not part of the WebRTC standard itself but crucial for setting up connections.
- PeerConnection: The core component for establishing peer-to-peer connections, handling media streams, and managing network traversal.
- MediaStream: Represents the audio and video data streams.
- NAT Traversal (ICE, STUN, TURN): Overcoming network address translation (NAT) challenges to establish direct peer-to-peer connections.
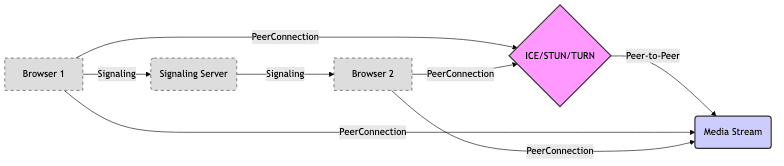
Core Components of WebRTC Applications
Let's delve deeper into the essential components of WebRTC applications.
Signaling Servers
Signaling is the process of exchanging metadata between peers to initiate and coordinate a WebRTC session. This metadata includes session descriptions (SDP) and ICE candidates. Signaling is typically implemented using a separate server and a protocol like WebSockets or Socket.IO.
javascript
1// Example using WebSockets for signaling
2const WebSocket = require('ws');
3
4const wss = new WebSocket.Server({ port: 8080 });
5
6wss.on('connection', ws => {
7 console.log('Client connected');
8
9 ws.on('message', message => {
10 console.log(`Received: ${message}`);
11
12 // Broadcast the message to all connected clients
13 wss.clients.forEach(client => {
14 if (client !== ws && client.readyState === WebSocket.OPEN) {
15 client.send(message);
16 }
17 });
18 });
19
20 ws.on('close', () => {
21 console.log('Client disconnected');
22 });
23});
24
25console.log('WebSocket server started on port 8080');
26
Peer-to-Peer Connections
The
RTCPeerConnection
interface is the core of WebRTC. It handles the establishment, maintenance, and termination of peer-to-peer connections. It also manages media streams, security, and NAT traversal.javascript
1// Establishing a PeerConnection
2const pc = new RTCPeerConnection({
3 iceServers: [
4 { urls: 'stun:stun.l.google.com:19302' }
5 ]
6});
7
8pc.onicecandidate = e => {
9 if (e.candidate) {
10 console.log('ICE candidate:', e.candidate);
11 // Send the candidate to the other peer via signaling
12 }
13};
14
15pc.ontrack = e => {
16 console.log('Track received', e.streams[0]);
17 // Handle the received media stream
18};
19
20// Create an offer
21pc.createOffer()
22 .then(offer => pc.setLocalDescription(offer))
23 .then(() => {
24 console.log('Offer created:', pc.localDescription);
25 // Send the offer to the other peer via signaling
26 });
27
Media Streams
MediaStream
represents a stream of audio and/or video data. You can access media streams from the user's microphone and camera using the getUserMedia
API. The tracks of the media stream can be added to the RTCPeerConnection
to be sent to the remote peer.javascript
1// Accessing user's microphone and camera
2navigator.mediaDevices.getUserMedia({ audio: true, video: true })
3 .then(stream => {
4 console.log('Got MediaStream:', stream);
5 // Add the stream to the RTCPeerConnection
6 stream.getTracks().forEach(track => pc.addTrack(track, stream));
7 })
8 .catch(error => {
9 console.error('Error accessing media devices.', error);
10 });
11
ICE Candidates and NAT Traversal
ICE (Interactive Connectivity Establishment) is a framework used by WebRTC to overcome the challenges of NAT (Network Address Translation) and firewalls. ICE gathers potential connection candidates (IP addresses and ports) and attempts to establish a direct peer-to-peer connection. STUN (Session Traversal Utilities for NAT) and TURN (Traversal Using Relays around NAT) servers are used to assist in this process. STUN allows a client to discover its public IP address. If a direct connection is not possible due to symmetric NAT or firewall restrictions, TURN acts as a relay server, forwarding traffic between the peers.
Developing a Simple WebRTC Chat Application
Let's walk through building a basic WebRTC chat application.
Setting up the Development Environment
- Install Node.js and npm: Required for backend development and package management.
- Create Project Directory: Set up a directory for your project.
- Initialize npm:
npm init -y
- Install Dependencies: Install necessary libraries (e.g.,
ws
for WebSockets).
Frontend Development
Create the HTML structure and JavaScript code for the user interface and WebRTC logic.
html
1<!DOCTYPE html>
2<html>
3<head>
4 <title>WebRTC Chat</title>
5</head>
6<body>
7 <h1>WebRTC Chat</h1>
8 <video id="localVideo" autoplay muted></video>
9 <video id="remoteVideo" autoplay></video>
10 <script src="script.js"></script>
11</body>
12</html>
13
javascript
1// JavaScript code for handling peer connections
2const localVideo = document.getElementById('localVideo');
3const remoteVideo = document.getElementById('remoteVideo');
4
5// ... (WebRTC code from previous examples, adapted for the chat application)
6
7//Example using WebSockets for signaling
8const ws = new WebSocket('ws://localhost:8080');
9
10ws.onopen = () => {
11 console.log('Connected to signaling server');
12};
13
14ws.onmessage = (event) => {
15 console.log('Received message:', event.data);
16
17 // Handle signaling messages (offer, answer, ICE candidates)
18 const message = JSON.parse(event.data);
19 if (message.type === 'offer') {
20 // Handle offer
21 } else if (message.type === 'answer') {
22 // Handle answer
23 } else if (message.type === 'iceCandidate') {
24 // Handle ICE candidate
25 }
26};
27
28ws.onerror = (error) => {
29 console.error('WebSocket error:', error);
30};
31
Backend Development (Optional)
Implement a simple Node.js server for signaling.
javascript
1// Simple Node.js server for signaling (using ws library)
2const WebSocket = require('ws');
3
4const wss = new WebSocket.Server({ port: 8080 });
5
6wss.on('connection', ws => {
7 console.log('Client connected');
8
9 ws.on('message', message => {
10 console.log(`Received: ${message}`);
11
12 // Broadcast the message to all connected clients
13 wss.clients.forEach(client => {
14 if (client !== ws && client.readyState === WebSocket.OPEN) {
15 client.send(message);
16 }
17 });
18 });
19
20 ws.on('close', () => {
21 console.log('Client disconnected');
22 });
23});
24
25console.log('WebSocket server started on port 8080');
26
Testing and Deployment
Test your application locally using two browser windows or devices. For deployment, consider using a cloud hosting platform and configuring appropriate STUN/TURN servers.
Advanced WebRTC Concepts
Explore these advanced topics to enhance your WebRTC applications.
Handling Multiple Peers
For group video calls or conferencing, you'll need to manage multiple
RTCPeerConnection
instances. Techniques like Selective Forwarding Units (SFUs) can optimize bandwidth usage in multi-party scenarios.WebRTC Security Considerations
- Encryption: WebRTC mandates encryption for all media streams.
- Signaling Security: Secure your signaling channel using HTTPS and authentication.
- Data Channel Security: Consider security implications when using data channels.
- Authentication and Authorization: Implement authentication and authorization mechanisms to control access to your application and protect against unauthorized users.
Scaling WebRTC Applications
- SFUs (Selective Forwarding Units): Forward only relevant media streams to each participant.
- MCUs (Multipoint Control Units): Mix media streams into a single stream.
- Load Balancing: Distribute signaling and media processing across multiple servers.
Integrating with Other Services
WebRTC can be integrated with other services like:
- Cloud Recording: Record WebRTC sessions to cloud storage.
- Transcription Services: Transcribe audio from WebRTC streams in real time.
- AI/ML Integration: Integrate AI/ML models for features like background removal or noise cancellation.
WebRTC Libraries and Frameworks
Leverage these libraries and frameworks to simplify WebRTC development.
Popular WebRTC Libraries
- adapter.js: Handles browser compatibility issues.
- SimpleWebRTC: Simplifies the process of creating a WebRTC application by providing a higher-level API.
- Kurento: A comprehensive media server framework for advanced WebRTC applications.
Choosing the Right Framework
Consider factors like:
- Complexity: How much abstraction do you need?
- Features: What features are included out-of-the-box?
- Community Support: Is there a strong community for support and resources?
- Cost: Is the framework free or commercial?
Comparison of Popular Options
Framework | Pros | Cons |
---|---|---|
SimpleWebRTC | Easy to use, good for simple applications | Limited flexibility, not suitable for complex scenarios |
Kurento | Powerful, feature-rich, suitable for complex applications | More complex to set up and use, requires a dedicated server |
Jitsi Meet | Open-source, complete video conferencing solution | Can be resource-intensive, requires significant infrastructure setup |
Real-World WebRTC Application Examples
Explore these diverse WebRTC use cases.
Video Conferencing
Applications like Zoom, Google Meet, and Jitsi Meet leverage WebRTC for real-time video conferencing.
Live Streaming
Platforms like Twitch and YouTube Live utilize WebRTC for low-latency live streaming.
Collaborative Tools
WebRTC powers collaborative tools like shared whiteboards and co-editing applications.
Other Use Cases
- Telemedicine
- Remote Education
- Gaming
- Customer Support
Troubleshooting Common WebRTC Issues
Address these common challenges when developing WebRTC applications.
Connection Problems
- Firewall Issues: Ensure firewalls are not blocking WebRTC traffic.
- NAT Traversal Problems: Verify STUN/TURN server configuration.
- Signaling Issues: Debug signaling messages for errors.
Media Issues
- Audio/Video Not Working: Check browser permissions and device settings.
- Poor Media Quality: Optimize media encoding and network conditions.
- Codec Incompatibility: Ensure peers support compatible codecs.
Browser Compatibility
Use adapter.js to handle browser-specific differences.
Other Common Problems
- ICE Gathering Timeout: Increase ICE gathering timeout.
- Signaling Server Issues: Ensure that the signaling server is working correctly.
- Bandwidth Limitations: Adjust media stream quality to accommodate bandwidth constraints.
Conclusion
WebRTC application development opens a world of possibilities for creating real-time communication experiences. By understanding the core components, leveraging available libraries and frameworks, and addressing potential challenges, you can build innovative and engaging applications. As WebRTC technology continues to evolve, staying updated with the latest advancements and best practices will be crucial for success in this dynamic field.
Further Resources:
- Learn more about WebRTC:
Official WebRTC website
- WebRTC best practices:
Mozilla WebRTC documentation
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ