Why Socket.IO? A Deep Dive into Real-Time Communication
The Need for Real-Time Communication
In today's fast-paced digital world, users expect instant updates and seamless interactions. Traditional request-response models fall short when delivering real-time experiences, making technologies like Socket.IO crucial.
What is Socket.IO?
Socket.IO is a JavaScript library that enables real-time, bidirectional, and event-based communication between web clients and servers. It simplifies the process of building real-time applications by providing a higher-level abstraction over WebSockets while gracefully falling back to other techniques when WebSockets are not available. Socket.IO is a popular solution for building chat apps, real-time dashboards, online games, and collaborative tools.
Socket.IO's Core Advantages
Socket.IO offers several key advantages that make it a preferred choice for real-time web application development.
Bidirectional Communication: Pushing Data Efficiently
Unlike traditional HTTP requests, Socket.IO allows both the client and server to send data to each other at any time. This bidirectional communication is essential for real-time applications where updates need to be pushed to the client without waiting for a request.
1// Client-side: Emitting an event
2socket.emit('new message', 'Hello, server!');
3
1// Server-side: Receiving and responding to the event
2io.on('connection', (socket) => {
3 socket.on('new message', (message) => {
4 console.log('Received message:', message);
5 socket.emit('message received', 'Message received successfully!');
6 });
7});
8
Event-Driven Architecture: Handling Real-time Updates
Socket.IO uses an event-driven architecture, making it easy to manage and respond to real-time events. Developers can define custom events and associate them with specific actions on both the client and server sides. This simplifies the process of handling real-time updates and interactions.
Seamless Fallback Mechanisms: Ensuring Connectivity
One of Socket.IO's strengths is its ability to seamlessly fall back to other communication methods when WebSockets are not available. This ensures that applications can function even in environments where WebSockets are blocked or unsupported. It provides a reliable experience across diverse network conditions and browsers, ensuring near universal support.
Scalability and Performance: Handling Many Concurrent Connections
Socket.IO is designed to handle a large number of concurrent connections, making it suitable for applications with many users. It is optimized for performance and can be scaled horizontally to accommodate growing traffic. Proper architecture is important for high scalability, including considerations for load balancing, message queues, and database interactions.
Socket.IO vs. WebSockets: Understanding the Differences
While Socket.IO uses WebSockets as its primary transport mechanism, it provides additional features and benefits that WebSockets alone do not offer. Understanding the differences between these technologies is crucial for choosing the right tool for your real-time application.
WebSockets: The Foundation of Real-Time Communication
WebSockets is a communication protocol that provides full-duplex communication channels over a single TCP connection. It enables persistent connections between clients and servers, allowing for real-time data exchange. However, WebSockets can be complex to implement directly, especially when dealing with different browser implementations and network environments.
Socket.IO: A Higher-Level Abstraction
Socket.IO builds on top of WebSockets by adding features like automatic reconnection, fallback to other transports (e.g., HTTP long-polling), and a simplified API for sending and receiving events. It handles many of the complexities of WebSockets, allowing developers to focus on building the application logic.
When to Choose Socket.IO over WebSockets
Choose Socket.IO over raw WebSockets when you need:
- Automatic reconnection: Socket.IO automatically reconnects clients after a disconnection.
- Fallback mechanisms: Socket.IO ensures connectivity even when WebSockets are not available.
- A simplified API: Socket.IO provides a more intuitive and easier-to-use API for handling real-time events.
- Namespaces and rooms: Socket.IO offers built-in support for organizing connections into namespaces and rooms.
- Broader browser support: Socket.IO has wider support for older browsers thanks to fallbacks.
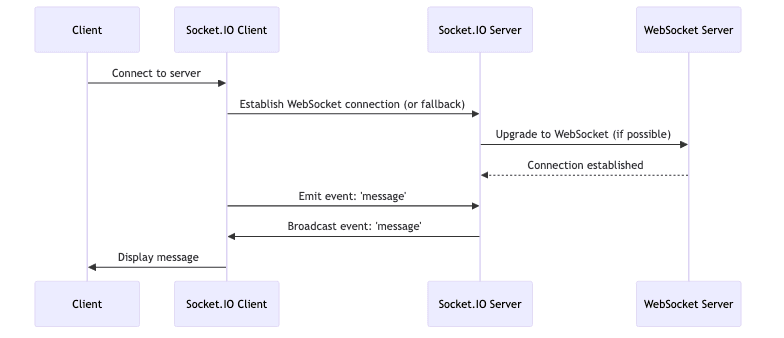
Practical Use Cases for Socket.IO
Socket.IO is a versatile library that can be used in a wide range of real-time applications.
Building Interactive Chat Applications
Chat applications are a classic use case for Socket.IO. The library's bidirectional communication and event-driven architecture make it easy to send and receive messages in real-time, creating a seamless and engaging user experience. Features such as typing indicators, online presence, and message history can be easily implemented.
Real-time Collaboration Tools
Socket.IO can be used to build real-time collaboration tools such as shared document editors, project management platforms, and virtual whiteboards. The ability to instantly synchronize changes across multiple clients enables users to work together seamlessly in real-time.
Live Data Streaming and Monitoring
Socket.IO is ideal for streaming live data from servers to clients, such as stock prices, sensor data, or social media feeds. The library's high performance and scalability make it suitable for handling large volumes of data in real-time. It can also be used to build real-time dashboards and monitoring systems that provide users with up-to-the-minute information.
Gaming and Interactive Entertainment
Socket.IO is a popular choice for building online games and interactive entertainment applications. The library's low latency and bidirectional communication enable developers to create responsive and engaging gaming experiences. Real-time multiplayer games, virtual worlds, and interactive simulations can all be built using Socket.IO.
Implementing Socket.IO: A Step-by-Step Guide
Implementing Socket.IO involves setting up both the server-side (typically using Node.js) and the client-side (using JavaScript). The following steps provide a basic overview of the process.
Setting up the Server-Side (Node.js)
First, you need to install the Socket.IO library using npm:
1npm install socket.io
2
Then, you can set up a basic Socket.IO server in Node.js:
1// server.js
2const { Server } = require("socket.io");
3
4const io = new Server({ /* options */ });
5
6io.on("connection", (socket) => {
7 console.log('A user connected');
8
9 socket.on('disconnect', () => {
10 console.log('User disconnected');
11 });
12
13 socket.on('chat message', (msg) => {
14 console.log('message: ' + msg);
15 io.emit('chat message', msg);
16 });
17
18});
19
20io.listen(3000, () => {
21 console.log('server running at http://localhost:3000');
22});
23
Connecting the Client-Side (JavaScript)
On the client-side, you need to include the Socket.IO client library and connect to the server:
1<!DOCTYPE html>
2<html>
3<head>
4 <title>Socket.IO Chat</title>
5 <script src="/socket.io/socket.io.js"></script>
6 <script>
7 const socket = io();
8
9 socket.emit('chat message', 'Hello from the client!');
10
11 socket.on('chat message', function(msg) {
12 console.log('Message from server: ' + msg);
13 });
14 </script>
15</head>
16<body>
17 <h1>Socket.IO Chat</h1>
18</body>
19</html>
20
Handling Events and Data
Once the client and server are connected, you can start emitting and handling events. Use the
socket.emit()
method to send data to the server, and the socket.on()
method to listen for events from the server. The io.emit
method sends events to all connected clients.Advanced Socket.IO Features
Socket.IO offers several advanced features that can be used to enhance real-time applications.
Namespaces and Rooms: Organizing Connections
Namespaces allow you to create separate communication channels within the same Socket.IO server. Rooms allow you to group clients together and send messages to specific groups of users. These features are useful for organizing connections and managing complex real-time applications.
Authentication and Authorization
Socket.IO provides mechanisms for authenticating and authorizing users before allowing them to connect to the server. This ensures that only authorized users can access sensitive data and functionality.
Error Handling and Robustness
Proper error handling is crucial for building robust and reliable real-time applications. Socket.IO provides mechanisms for detecting and handling errors, such as connection errors and data validation errors. Implementing comprehensive error handling can prevent application crashes and ensure a smooth user experience.
Conclusion: Socket.IO's Place in Modern Web Development
Socket.IO is a powerful and versatile library that simplifies the process of building real-time web applications. Its bidirectional communication, event-driven architecture, and seamless fallback mechanisms make it a preferred choice for developers who want to create engaging and interactive user experiences. As the demand for real-time applications continues to grow, Socket.IO will remain an important tool in the modern web developer's arsenal.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ