Building Your Angular Chat App: A Comprehensive Guide
Introduction: Why Choose Angular for Your Chat App?
Angular, a powerful and versatile JavaScript framework, is an excellent choice for building modern, real-time chat applications. Its component-based architecture, robust data binding, and extensive ecosystem make it ideal for creating scalable and maintainable chat solutions.
The Power of Angular
Angular offers several advantages for chat app development. Its two-way data binding simplifies UI updates, while its modular structure promotes code reusability. Typescript support makes the app more robust and scalable.
Choosing the Right Backend
While Angular handles the front-end, a reliable backend is crucial for real-time communication. Options include Node.js with Socket.IO, Firebase Realtime Database, or PubNub. The backend handles message persistence, user authentication, and real-time updates.
Setting Up Your Development Environment
Before diving into coding, let's set up your development environment.
Prerequisites
- A code editor (e.g., VS Code, Sublime Text)
- Basic knowledge of HTML, CSS, and JavaScript/TypeScript
- A modern web browser (e.g., Chrome, Firefox)
Installing Node.js and npm
Angular requires Node.js and npm (Node Package Manager). Download and install the latest LTS version of Node.js from the official website:
https://nodejs.org/
. npm comes bundled with Node.js.Verify the installation by running the following commands in your terminal:
1node -v
2npm -v
3
Setting up Angular CLI
The Angular CLI (Command Line Interface) simplifies project creation, component generation, and deployment. Install it globally using npm:
1npm install -g @angular/cli
2
Verify the installation:
1ng version
2
Project Structure and Key Components
Now, let's create the Angular project and explore its structure.
Project Setup with Angular CLI
Use the Angular CLI to generate a new project:
1ng new angular-chat-app
2cd angular-chat-app
3
Choose the following options during project setup:
- Would you like to add Angular routing? Yes
- Which stylesheet format would you like to use? CSS (or your preferred format)
This will create a new Angular project with a basic structure, including modules, components, and routing.
Core Components: Chat Service, Message Component, User Component
Our chat app will consist of the following core components:
- Chat Service: Handles real-time communication logic (e.g., connecting to Socket.IO, sending/receiving messages).
- Message Component: Displays individual chat messages, including sender and timestamp.
- User Component: Manages user authentication, profile information, and online status.
Create these components using the Angular CLI:
1ng generate service chat
2ng generate component message
3ng generate component user
4
These commands will generate the necessary files (e.g.,
chat.service.ts
, message.component.ts
, user.component.ts
) in your project.Implementing Real-time Communication
Real-time communication is the heart of any chat application. We'll explore two popular options: Socket.IO and Firebase.
Choosing a Real-time Communication Technology
- Socket.IO: A library that enables real-time, bidirectional and event-based communication between web clients and servers. Requires a dedicated server.
- Pros: Flexible, high performance, suitable for complex chat features.
- Cons: Requires server setup and maintenance.
- Firebase Realtime Database: A cloud-hosted NoSQL database that allows you to store and synchronize data between users in real-time.
- Pros: Easy to set up, serverless, scalable.
- Cons: Limited query capabilities, can be expensive for high usage.
- PubNub: A real-time communication platform that provides APIs for building chat, streaming, and other real-time applications.
- Pros: Reliable, scalable, provides a wide range of features.
- Cons: Can be more expensive than other options.
Integrating Socket.IO
First, install the Socket.IO client library:
1npm install socket.io-client
2
In your
chat.service.ts
, add the following code to connect to the Socket.IO server:Typescript
1import { Injectable } from '@angular/core';
2import { io } from 'socket.io-client';
3import { Observable } from 'rxjs';
4
5@Injectable({
6 providedIn: 'root'
7})
8export class ChatService {
9 private socket = io('http://localhost:3000'); // Replace with your server URL
10
11 joinRoom(data:any):
12 void{
13 this.socket.emit('join', data);
14 }
15
16 sendMessage(data:any):
17 void{
18 this.socket.emit('message',data);
19 }
20
21 getMessage(): Observable<any> {
22 return new Observable(observer => {
23 this.socket.on('new message', (data:any) => {
24 observer.next(data);
25 });
26 });
27 }
28
29 getJoinedUsers(): Observable<any> {
30 return new Observable(observer => {
31 this.socket.on('user joined', (data:any) => {
32 observer.next(data);
33 });
34 });
35 }
36
37 leaveRoom(data:any):
38 void{
39 this.socket.emit('leave',data);
40 }
41}
42
Typescript
1// Example usage in a component
2import { Component, OnInit } from '@angular/core';
3import { ChatService } from './chat.service';
4
5@Component({
6 selector: 'app-chat',
7 templateUrl: './chat.component.html',
8 styleUrls: ['./chat.component.css']
9})
10export class ChatComponent implements OnInit {
11
12 constructor(private chatService: ChatService) { }
13
14 ngOnInit(): void {
15 this.chatService.getMessage().subscribe((msg:any) => {
16 console.log(msg);
17 });
18 }
19
20 sendMessage(){
21 this.chatService.sendMessage({message: 'test'});
22 }
23
24}
25
Remember to replace
'http://localhost:3000'
with the actual URL of your Socket.IO server. You'll also need to set up the Socket.IO server using Node.js. This is a separate step, and the code for the server is beyond the scope of this blog post.Integrating Firebase
First, install the AngularFire library and Firebase SDK:
1npm install @angular/fire firebase
2
Initialize Firebase in your
app.module.ts
:Typescript
1import { NgModule } from '@angular/core';
2import { BrowserModule } from '@angular/platform-browser';
3import { AngularFireModule } from '@angular/fire/compat';
4import { AngularFireDatabaseModule } from '@angular/fire/compat/database';
5import { environment } from '../environments/environment';
6
7import { AppComponent } from './app.component';
8
9@NgModule({
10 declarations: [
11 AppComponent
12 ],
13 imports: [
14 BrowserModule,
15 AngularFireModule.initializeApp(environment.firebase),
16 AngularFireDatabaseModule
17 ],
18 providers: [],
19 bootstrap: [AppComponent]
20})
21export class AppModule { }
22
Replace
environment.firebase
with your Firebase project configuration. You can find this configuration in the Firebase console.In your
chat.service.ts
, add the following code to read and write messages to Firebase:Typescript
1import { Injectable } from '@angular/core';
2import { AngularFireDatabase } from '@angular/fire/compat/database';
3import { Observable } from 'rxjs';
4
5@Injectable({
6 providedIn: 'root'
7})
8export class ChatService {
9
10 constructor(private db: AngularFireDatabase) { }
11
12 sendMessage(message: string, user: string) {
13 this.db.list('/messages').push({ user: user, message: message });
14 }
15
16 getMessages(): Observable<any[]> {
17 return this.db.list('/messages').valueChanges();
18 }
19}
20
This code uses the
AngularFireDatabase
service to push new messages to the /messages
node in your Firebase Realtime Database and retrieve existing messages.Building the User Interface
Now, let's design the user interface for our chat app.
Designing the Chat Interface
The chat interface should include the following elements:
- A message input field for typing messages.
- A send button for submitting messages.
- A message display area for showing chat messages.
- A user list (optional) for displaying online users.
Implementing Material Design
Angular Material provides a set of reusable UI components that can help you create a visually appealing and user-friendly chat interface. Install Angular Material:
1ng add @angular/material
2
Typescript
1// Example of using Angular Material components in your template
2<mat-card>
3 <mat-card-content>
4 <mat-form-field>
5 <input matInput placeholder="Message" [(ngModel)]="messageText">
6 </mat-form-field>
7 <button mat-raised-button color="primary" (click)="sendMessage()">Send</button>
8 </mat-card-content>
9</mat-card>
10
11<mat-list>
12 <mat-list-item *ngFor="let message of messages">
13 {{ message.user }}: {{ message.message }}
14 </mat-list-item>
15</mat-list>
16
This example uses
mat-card
, mat-form-field
, matInput
, mat-raised-button
, and mat-list
components to create a basic chat interface.Handling User Input and Message Display
Bind the message input field to a component variable (e.g.,
messageText
) using [(ngModel)]
. When the user clicks the send button, call a function (e.g., sendMessage()
) to send the message to the backend. Display the messages in the message display area using *ngFor
to iterate over an array of messages.Implementing User Authentication
User authentication is essential for securing your chat app and preventing unauthorized access.
Choosing an Authentication Method
Several authentication methods are available, including:
- Firebase Authentication: Provides a complete authentication solution with support for email/password, social logins, and more.
- Custom authentication: Requires you to implement your own authentication logic, including user registration, login, and password management.
Integrating Firebase Authentication
Enable Firebase Authentication in your Firebase console. Then, install the AngularFire Authentication library:
1npm install @angular/fire/compat/auth
2
Typescript
1// Example of setting up Firebase Authentication in your component
2import { Component } from '@angular/core';
3import { AngularFireAuth } from '@angular/fire/compat/auth';
4
5@Component({
6 selector: 'app-login',
7 templateUrl: './login.component.html',
8 styleUrls: ['./login.component.css']
9})
10export class LoginComponent {
11
12 constructor(public auth: AngularFireAuth) {}
13
14 login() {
15 this.auth.signInWithEmailAndPassword('test@example.com', 'password'); //Replace
16 }
17
18 logout() {
19 this.auth.signOut();
20 }
21}
22
Typescript
1// Example of protecting chat routes using Angular Guards
2import { Injectable } from '@angular/core';
3import { CanActivate, Router } from '@angular/router';
4import { AngularFireAuth } from '@angular/fire/compat/auth';
5import { Observable } from 'rxjs';
6import { map } from 'rxjs/operators';
7
8@Injectable({
9 providedIn: 'root'
10})
11export class AuthGuard implements CanActivate {
12
13 constructor(private auth: AngularFireAuth, private router: Router) {}
14
15 canActivate(): Observable<boolean> {
16 return this.auth.authState.pipe(
17 map(user => {
18 if (user) {
19 return true;
20 } else {
21 this.router.navigate(['/login']);
22 return false;
23 }
24 })
25 );
26 }
27}
28
This code uses
AngularFireAuth
to sign in and sign out users. The AuthGuard
protects chat routes by redirecting unauthenticated users to the login page.Secure Data Handling
Always validate and sanitize user input to prevent security vulnerabilities such as cross-site scripting (XSS) attacks. Use secure coding practices to protect sensitive data, such as passwords.
Testing and Deployment
Thorough testing and proper deployment are crucial for ensuring the quality and reliability of your chat app.
Unit Testing
Write unit tests to verify the functionality of individual components and services. Use a testing framework like Jest or Karma.
End-to-End Testing
Perform end-to-end testing to ensure that the entire chat app works correctly, including user authentication, real-time communication, and UI interactions. Use a testing framework like Cypress or Protractor.
Deployment Strategies
Deploy your Angular chat app to a hosting platform such as Netlify, Vercel, or Firebase Hosting. Configure your backend server to handle real-time communication and data persistence. Set up a CI/CD pipeline to automate the deployment process.
Advanced Features and Optimization
Consider adding the following advanced features to enhance your chat app:
Handling Multiple Rooms
Implement the ability to create and join multiple chat rooms. Allow users to switch between rooms and participate in different conversations.
Implementing Private Messaging
Enable private messaging between individual users. Provide a mechanism for users to initiate private conversations and exchange messages securely.
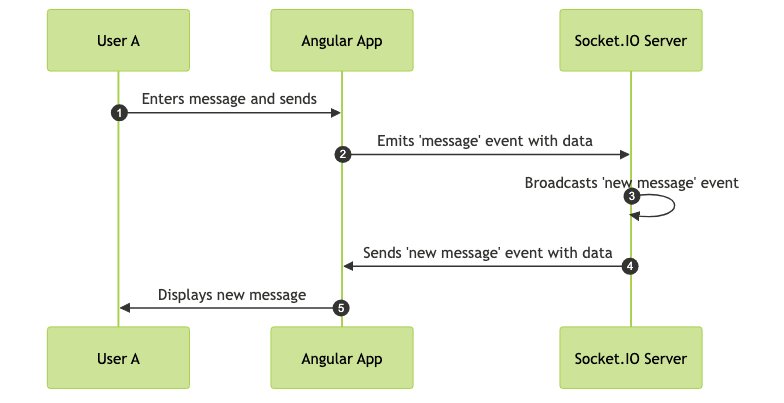
Conclusion: Your Angular Chat App is Ready!
Congratulations! You have successfully built a real-time chat application using Angular. This guide has covered the essential steps, from setting up your development environment to implementing advanced features and deployment strategies. Remember to continue exploring and refining your chat app to meet the specific needs of your users.
- Learn more about Angular:
Official Angular documentation
. - Socket.IO documentation:
Documentation for the Socket.IO library
. - Firebase documentation:
Documentation for Firebase
.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ