Introduction to WebSockets and Their Diverse Use Cases
WebSockets have revolutionized real-time communication on the web, offering a persistent, bidirectional communication channel between a client and a server. Unlike traditional HTTP, which follows a request-response model, WebSockets enable full-duplex communication, allowing both the client and server to send data independently and simultaneously. This capability unlocks a vast array of applications that demand low latency and continuous data exchange. We'll explore diverse
websocket use cases
in detail in this article.What are WebSockets?
WebSockets are a communication protocol that provides a persistent connection between a client and a server. This persistent connection facilitates real-time data transfer. It operates over TCP and establishes a single, long-lived connection for bidirectional data flow. The
websocket architecture
is designed to minimize overhead and latency, making it ideal for real-time applications websocket
.Why Use WebSockets?
WebSockets offer several significant advantages over traditional HTTP. The primary benefit is the ability to push data from the server to the client without the client explicitly requesting it. This eliminates the need for frequent polling, reducing latency and bandwidth consumption. WebSockets enable
bidirectional communication websocket
which translates to better user experiences in interactive applications. The websocket benefits
are particularly noticeable in applications requiring real-time updates
.WebSockets vs. Traditional HTTP: A Comparison
Traditional HTTP is a stateless protocol, meaning each request from the client is treated independently. This works well for static content but becomes inefficient for real-time updates. WebSockets, on the other hand, maintain a persistent connection, allowing the server to push updates to the client as soon as they are available. This
full-duplex communication websocket
leads to lower latency and reduced server load compared to techniques like long polling or server-sent events. The core difference lies in the persistent connection and bidirectional nature of WebSockets versus the request-response model of HTTP, making websocket vs http
a key consideration for developers.Top 10 WebSockets Use Cases
WebSockets have become indispensable in many modern applications. Here are ten prominent
websocket use cases
:Real-time Chat Applications
One of the most common
websocket examples
is in real-time chat applications. WebSockets enable instant message delivery, online presence indicators, and group chat functionality. The persistent connection ensures that messages are delivered immediately, creating a seamless chat experience. Libraries like Socket.IO further simplify the development of chat applications.1// Client-side JavaScript
2const socket = new WebSocket('ws://localhost:3000');
3
4socket.onopen = () => {
5 console.log('Connected to WebSocket server');
6};
7
8socket.onmessage = (event) => {
9 const message = event.data;
10 console.log('Received message:', message);
11 // Display the message in the chat window
12};
13
14socket.onclose = () => {
15 console.log('Disconnected from WebSocket server');
16};
17
18function sendMessage(message) {
19 socket.send(message);
20}
21
Collaborative Editing Tools
Websocket collaborative editing
is crucial for applications like Google Docs or online code editors. WebSockets allow multiple users to simultaneously edit the same document, with changes reflected in real-time for all participants. Techniques like Operational Transformation (OT) or Conflict-free Replicated Data Types (CRDTs) are often used to handle concurrent edits.1// Example of sending document changes
2socket.send(JSON.stringify({ type: 'text-update', position: 10, text: 'new text' }));
3
4// Example of handling document changes received from the server
5socket.onmessage = (event) => {
6 const data = JSON.parse(event.data);
7 if (data.type === 'text-update') {
8 // Update the document content based on the received changes
9 updateDocument(data.position, data.text);
10 }
11};
12
13function updateDocument(position, text) {
14 // Implementation to update the document content at the specified position
15 console.log(`Updating document at position ${position} with text: ${text}`);
16}
17
Online Gaming
WebSockets are essential for
websocket gaming
, enabling real-time multiplayer interactions. Games require low latency to ensure a smooth and responsive experience. WebSockets facilitate the exchange of game state information, player movements, and other critical data in real-time. This allows developers to create engaging and interactive online games.Stock Tickers and Financial Data Streaming
Financial institutions use WebSockets for
websocket stock tickers
and websocket streaming
of financial data. Real-time updates of stock prices, market indices, and other financial information are crucial for traders and investors. WebSockets provide a reliable and efficient way to deliver this data with minimal delay.IoT Device Monitoring and Control
In the Internet of Things (IoT), WebSockets enable
websocket IoT
device monitoring and control. Devices can send sensor data to a central server in real-time, and users can remotely control these devices through a web interface. This is particularly useful in applications like smart homes, industrial automation, and environmental monitoring.Live Data Visualization and Dashboards
WebSockets are utilized for creating live data visualization dashboards. Information is pushed to the dashboard immediately, allowing users to monitor key metrics and identify trends. This is valuable in areas like business intelligence, network monitoring, and system administration.
Real-time Notifications and Alerts
Websocket notifications
are used to deliver immediate alerts and updates to users. Whether it's a new email notification, a social media mention, or a system alert, WebSockets ensure that users are informed in real-time. This improves user engagement and responsiveness.Social Media Updates and Feeds
Social media platforms leverage WebSockets to deliver real-time updates to user feeds. New posts, comments, and likes are instantly displayed, creating a dynamic and engaging social experience.
Location Tracking and Mapping
WebSockets facilitate location tracking and mapping applications. Real-time updates of device locations are transmitted to a server, allowing users to monitor the movement of vehicles, people, or assets.
Video Conferencing and Streaming
WebSockets are employed in video conferencing and streaming applications to manage signaling and metadata exchange. While the actual video and audio streams often use other protocols like WebRTC, WebSockets handle the control information, such as session management, user presence, and chat messages.
Building a WebSocket Application: A Step-by-Step Guide
Building a
websocket application
involves several key steps:Choosing the Right Framework or Library
Several frameworks and libraries simplify
websocket implementation
. Some popular options include Socket.IO, ws (Node.js), Autobahn (Python), and Jetty (Java). Socket.IO is a popular choice because it provides fallback mechanisms for older browsers that do not support WebSockets natively. When selecting a library, consider factors such as language support, features, performance, and community support.Setting up the Server
The server-side component is responsible for handling WebSocket connections, managing data exchange, and implementing application logic. You can use a framework or library to simplify server development. The
websocket server
manages connections and message routing.1// Setting up a basic WebSocket server using Node.js and Socket.IO
2const io = require('socket.io')(3000, {
3 cors: {
4 origin: "*",
5 methods: ["GET", "POST"]
6 }
7});
8
9io.on('connection', socket => {
10 console.log('A user connected');
11
12 socket.on('disconnect', () => {
13 console.log('A user disconnected');
14 });
15
16 socket.on('message', message => {
17 console.log(`Received message: ${message}`);
18 io.emit('message', message); // Broadcast the message to all connected clients
19 });
20});
21
Developing the Client-Side Application
The client-side application establishes a WebSocket connection to the server and handles data exchange. JavaScript is commonly used for client-side WebSocket development.
Javascript websocket
integration is well-supported in modern browsers.1// Connecting to a WebSocket server and handling messages in JavaScript
2const socket = new WebSocket('ws://localhost:3000');
3
4socket.onopen = () => {
5 console.log('Connected to WebSocket server');
6};
7
8socket.onmessage = (event) => {
9 const message = event.data;
10 console.log('Received message:', message);
11 // Handle the received message
12};
13
14socket.onclose = () => {
15 console.log('Disconnected from WebSocket server');
16};
17
18function sendMessage(message) {
19 socket.send(message);
20}
21
Implementing Security Measures
Security is paramount when working with WebSockets. Implement measures such as validating user input, sanitizing data, and using secure protocols (WSS) to encrypt communication. Implement proper authentication and authorization mechanisms to control access to WebSocket endpoints.
Advanced WebSocket Techniques and Considerations
Handling WebSocket Disconnections and Reconnections
WebSockets can disconnect due to network issues or server-side problems. Implement robust mechanisms to handle disconnections gracefully and automatically attempt to reconnect. Use techniques like exponential backoff to avoid overwhelming the server with reconnection attempts.
Optimizing WebSocket Performance
Websocket performance
can be improved by minimizing the amount of data transmitted, using efficient data formats (e.g., binary data instead of text), and optimizing server-side processing. Consider using compression techniques to reduce bandwidth consumption.Scaling WebSocket Applications
Scaling
websocket scalability
can be challenging due to the persistent nature of connections. Techniques like load balancing, horizontal scaling, and message queues can be used to distribute WebSocket connections across multiple servers. Consider using a message broker like Redis or RabbitMQ to handle message routing.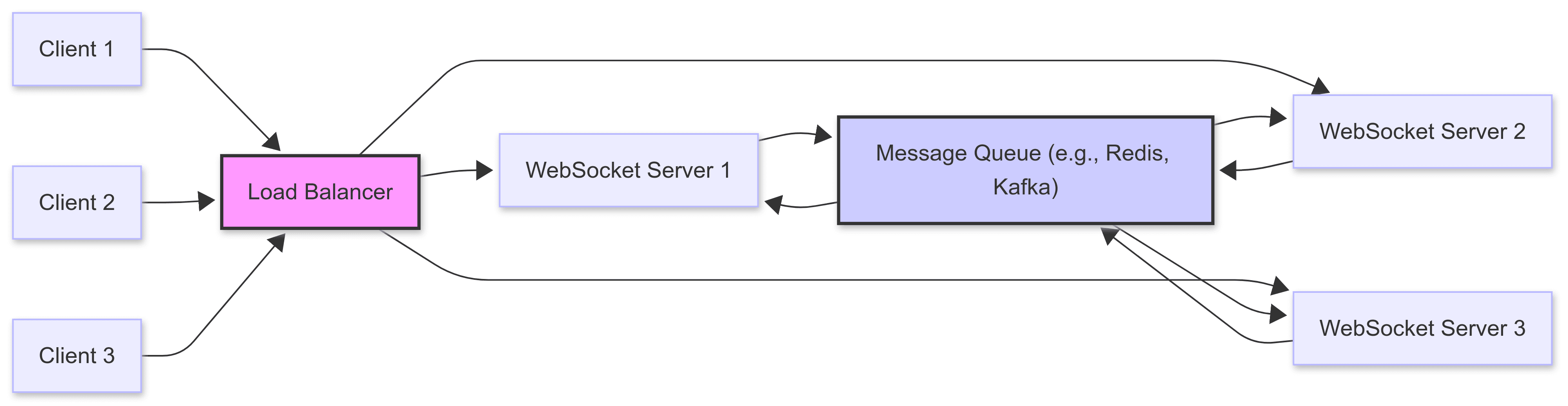
WebSocket Security Best Practices
Follow security best practices, such as validating origin headers to prevent cross-site WebSocket hijacking (CSWSH) attacks, using TLS encryption (WSS), and implementing authentication and authorization mechanisms.
Conclusion: The Future of WebSockets
WebSockets have transformed real-time communication on the web and continue to be a vital technology for modern applications. The ability to establish persistent, bidirectional connections has unlocked a wide range of new possibilities. As the demand for real-time experiences continues to grow, WebSockets will remain a crucial component of web development.
Further Reading:
- Learn more about WebSockets on MDN: "Dive deeper into the technical details of the WebSocket API." [
https://developer.mozilla.org/en-US/docs/Web/API/WebSocket
] - Explore Socket.IO for easier WebSocket development: "Discover a popular framework that simplifies WebSocket development." [
https://socket.io/
]
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ