WebSocket Communication API: A Comprehensive Guide
Introduction: Understanding the Power of WebSocket Communication API
The WebSocket Communication API has revolutionized real-time web applications. Unlike traditional HTTP, which is request-response based, WebSockets offer a persistent, bidirectional communication channel between a client and a server. This enables instant updates and a more interactive user experience.
What is a WebSocket Communication API?
A WebSocket Communication API is a technology that enables full-duplex communication channels over a single TCP connection. It provides a standardized way for clients and servers to exchange data in real-time, without the overhead of constantly re-establishing connections, making it perfect for building real-time applications.
Why Use WebSocket Communication API?
WebSocket communication APIs are ideal when you need instantaneous updates between client and server. This bidirectional and real-time capability makes it well-suited for applications like chat applications, online games, financial tickers, collaborative editing tools, and live dashboards. Using WebSocket improves the user experience by reducing latency and providing faster updates.
Key Advantages of WebSocket Communication API over Traditional HTTP
The key advantage of WebSocket over traditional HTTP lies in its full-duplex communication capability. HTTP requires the client to initiate each request, while WebSocket allows both client and server to send data at any time, resulting in lower latency and reduced server load. WebSocket establishes a persistent connection, eliminating the overhead of establishing a new connection for each message, which is crucial for real-time web applications.
Setting up a WebSocket Connection
Establishing a WebSocket connection involves a handshake process where the client requests an upgrade from HTTP to the WebSocket protocol. Once the connection is established, data can be transmitted bidirectionally until either the client or the server closes the connection.
Choosing the Right Protocol: ws vs. wss
When establishing a WebSocket connection, you have two protocol choices:
ws
and wss
. ws
is the standard WebSocket protocol, while wss
is the secure WebSocket protocol. wss
encrypts the data transmitted over the WebSocket connection using TLS/SSL, providing a secure channel for sensitive data. Always prefer wss
for production environments.Establishing a Connection using JavaScript
Here's how to establish a WebSocket connection in JavaScript:
Javascript
1// or 'wss://example.com/socketserver' for secure connection
2const socket = new WebSocket('ws://example.com/socketserver');
3
4//For a secure connection:
5//const socket = new WebSocket('wss://example.com/socketserver');
6
7socket.onopen = () => {
8 console.log('WebSocket connection established.');
9};
10
Handling Connection Events (open, close, error)
Handling connection events is crucial for managing the WebSocket connection lifecycle. You can listen for the
open
, close
, and error
events to handle different connection states:Javascript
1socket.onopen = () => {
2 console.log('Connection opened');
3 socket.send('Hello Server!');
4};
5
6socket.onclose = (event) => {
7 console.log('Connection closed:', event.code, event.reason);
8};
9
10socket.onerror = (error) => {
11 console.error('WebSocket error:', error);
12};
13
Understanding Connection States (CONNECTING, OPEN, CLOSING, CLOSED)
The WebSocket connection goes through several states during its lifecycle:
- CONNECTING: The connection is being established.
- OPEN: The connection is open and ready for communication.
- CLOSING: The connection is in the process of closing.
- CLOSED: The connection is closed.
You can access the current state of the connection using the
readyState
property of the WebSocket object.Javascript
1if (socket.readyState === WebSocket.OPEN) {
2 // Connection is open
3}
4
Sending and Receiving Data with WebSocket API
Once a WebSocket connection is established, you can send and receive data between the client and server using the
send()
method and the onmessage
event.Sending Data: The send()
method
The
send()
method is used to send data to the server. You can send text or binary data.Javascript
1// Sending text data
2socket.send('Hello, Server!');
3
4// Sending binary data (ArrayBuffer)
5const buffer = new ArrayBuffer(16);
6const view = new Uint8Array(buffer);
7view[0] = 0x01;
8socket.send(buffer);
9
Receiving Data: The onmessage
event
The
onmessage
event is triggered when the client receives data from the server. The event object contains the data in the data
property.Javascript
1socket.onmessage = (event) => {
2 console.log('Received:', event.data);
3};
4
Data Formatting: Text vs. Binary
WebSocket supports both text and binary data formats. Text data is typically encoded as UTF-8. Binary data is sent as
Blob
or ArrayBuffer
. Choosing the right format depends on the type of data you're sending. Binary data can be more efficient for certain types of data, such as images or audio.Handling Large Data Transfers
For large data transfers, it's often beneficial to split the data into smaller chunks. You can use the
send()
method to send each chunk separately. On the receiving end, you can reassemble the chunks into the complete data. Flow control and acknowledgements might be necessary for reliable delivery of large messages. Libraries exist to help with this, often implementing reliable messaging protocols on top of WebSockets.Building a Simple WebSocket Chat Application
Let's build a simple WebSocket chat application using Node.js for the server and JavaScript for the client.
Setting up the Server (Technology Choice Dependent - Example: Node.js with Socket.IO)
We'll use Node.js and Socket.IO, a popular WebSocket library, to set up the server:
Javascript
1const io = require('socket.io')(3000, {
2 cors: {
3 origin: "http://localhost:8080",
4 methods: ["GET", "POST"]
5 }
6});
7
8io.on('connection', socket => {
9 console.log("User connected: " + socket.id)
10 socket.on('send-message', (message, room) => {
11 if (room === '') {
12 socket.broadcast.emit('receive-message', message)
13 } else {
14 socket.to(room).emit('receive-message', message)
15 }
16 })
17 socket.on('join-room', room => {
18 socket.join(room)
19 })
20})
21
Implementing the Client-Side Logic
Here's the client-side JavaScript code:
Javascript
1const socket = io('http://localhost:3000')
2
3const messageInput = document.getElementById('message-input')
4const roomInput = document.getElementById('room-input')
5const form = document.getElementById('form')
6
7form.addEventListener('submit', e => {
8 e.preventDefault()
9 const message = messageInput.value
10 const room = roomInput.value
11 if (message === '') return
12 displayMessage(message)
13 socket.emit('send-message', message, room)
14 messageInput.value = ''
15})
16
17socket.on('receive-message', message => {
18 displayMessage(message)
19})
20
21socket.on('connect', () => {
22 displayMessage(`You connected with id: ${socket.id}`)
23})
24
25function displayMessage(message) {
26 const div = document.createElement('div')
27 div.textContent = message
28 document.getElementById('message-container').append(div)
29}
30
Handling Multiple Clients
Socket.IO makes it easy to handle multiple clients. Each client that connects to the server gets a unique socket ID. You can use this ID to identify and track individual clients. The library takes care of managing the connections and routing messages to the correct clients.
Adding Features: Usernames, Private Messaging
To add features like usernames and private messaging, you can associate a username with each socket connection. When a user sends a message, include their username in the message data. For private messaging, you can use Socket.IO's rooms feature to create a private channel between two users. The server can then route messages only to the users in that room.
Advanced WebSocket Techniques
WebSocket Subprotocols
WebSocket subprotocols are higher-level protocols that run over the base WebSocket protocol. They allow you to add structure and semantics to the data exchanged over the WebSocket connection. Subprotocols are negotiated during the WebSocket handshake. Examples include JSON Web Tokens (JWT) for authentication or specific protocols for game data.
Handling Errors and Disconnections Gracefully
It's crucial to handle errors and disconnections gracefully to provide a robust user experience. Listen for the
error
and close
events on the WebSocket object. When an error occurs, display an informative message to the user and attempt to reconnect. When the connection closes, clean up resources and notify the user.Implementing Security Best Practices
Security is paramount when using WebSockets. Always use
wss
for secure communication. Validate and sanitize all data received from the client to prevent injection attacks. Implement authentication and authorization mechanisms to ensure that only authorized users can access the WebSocket server. Consider using a Content Security Policy (CSP) to mitigate cross-site scripting (XSS) attacks.Choosing the Right WebSocket Framework or Library
Several WebSocket frameworks and libraries can simplify WebSocket development. Choosing the right one depends on your specific needs and the programming language you're using.
Popular Frameworks and Libraries
- Socket.IO: A popular library for Node.js that provides a simple and high-level API for building real-time applications. It handles fallback to HTTP long-polling for browsers that don't support WebSockets.
- AutobahnJS: A JavaScript library for building WebSocket applications with support for WAMP (WebSocket Application Messaging Protocol).
- ws: A simple and fast WebSocket client and server library for Node.js.
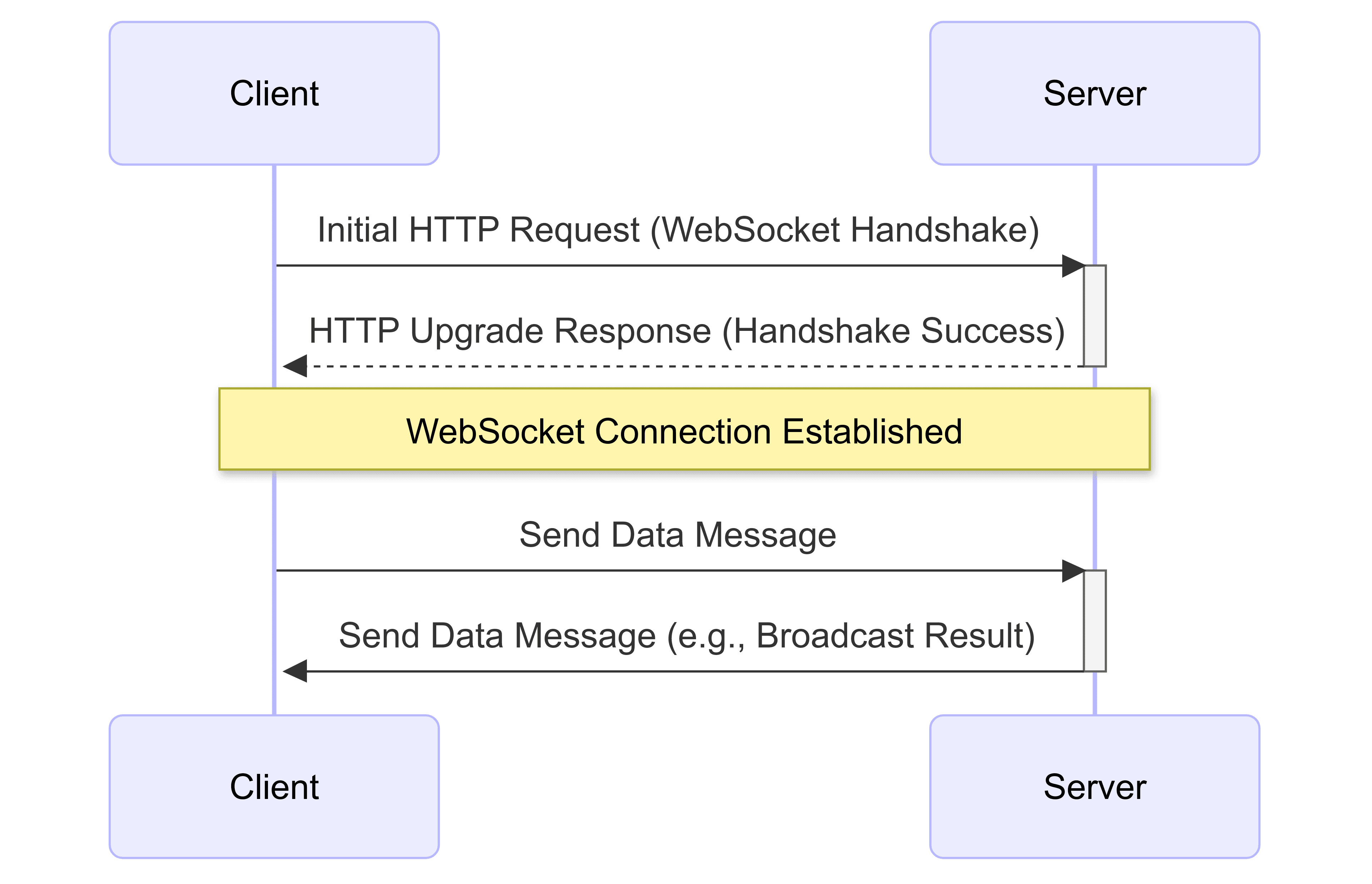
Factors to Consider When Choosing a Framework
Consider the following factors when choosing a WebSocket framework:
- Ease of use: Is the framework easy to learn and use?
- Features: Does the framework provide the features you need?
- Performance: Is the framework performant enough for your application?
- Community support: Is there a large and active community supporting the framework?
- Language support: Does the framework support the programming language you're using?
- Scalability: Can the framework be scaled to handle a large number of concurrent connections?
Conclusion: The Future of WebSocket Communication API
The WebSocket Communication API is a powerful technology for building real-time web applications. Its full-duplex communication capability and low latency make it ideal for a wide range of use cases. As web applications become increasingly interactive and real-time, WebSockets will continue to play a crucial role in delivering engaging user experiences.
Learn more about WebSocket protocols:
IETF WebSocket Protocol Specification
Explore WebSocket API in MDN:
MDN WebSockets API Documentation
Dive deeper into AsyncAPI:
AsyncAPI - Specification for event-driven architectures
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ