Node Websocket Server: A Comprehensive Guide
WebSockets have revolutionized how we build real-time applications, enabling bidirectional communication between a server and client. Node.js, with its non-blocking, event-driven architecture, provides an ideal environment for building scalable and efficient WebSocket servers. This guide will take you through the process of creating, scaling, and deploying a Node.js WebSocket server.
Introduction to Node Websocket Servers
What are WebSockets?
WebSockets provide a persistent connection between a client and a server, allowing for real-time, bidirectional data transfer. Unlike traditional HTTP requests, WebSockets maintain an open connection, reducing latency and overhead.
Why Choose Node.js for WebSockets?
Node.js excels at handling concurrent connections due to its asynchronous, non-blocking I/O model. This makes it well-suited for WebSocket servers that need to manage numerous clients simultaneously. Node's event-driven architecture enables efficient processing of WebSocket events like connection, disconnection, and message handling.
Benefits of Using Node.js WebSockets
- Real-time Communication: Enables instant data updates.
- Scalability: Node.js can handle a large number of concurrent connections.
- Efficiency: Reduced latency compared to traditional HTTP polling.
- Bidirectional Communication: Server and client can send data to each other simultaneously.
- Large Ecosystem: A vast number of libraries and resources are available for Node.js.
Setting Up Your Node.js Websocket Server Environment
Installing Node.js and npm
Before you begin, ensure that Node.js and npm (Node Package Manager) are installed on your system. You can download the latest version from the official Node.js website (
https://nodejs.org
).Choosing a Websocket Library: ws
Several WebSocket libraries are available for Node.js, but
ws
is a popular and well-maintained choice due to its performance and adherence to the WebSocket protocol.1npm install ws
2
Project Setup and Initialization
Create a new directory for your project and initialize it with npm.
1mkdir node-websocket-server
2cd node-websocket-server
3npm init -y
4
This will create a
package.json
file in your project directory. Your basic project will consist of a server.js
file and a package.json
file, at the minimum. You can create this server.js
with the command:1touch server.js
2
JSON
1{
2 "name": "node-websocket-server",
3 "version": "1.0.0",
4 "description": "A basic Node.js WebSocket server",
5 "main": "server.js",
6 "scripts": {
7 "start": "node server.js"
8 },
9 "dependencies": {
10 "ws": "^8.0.0"
11 }
12}
13
Building a Basic Node.js Websocket Server
Creating the Server
Create a file named
server.js
and add the following code to set up a basic WebSocket server using the ws
library.Javascript
1const WebSocket = require('ws');
2
3const wss = new WebSocket.Server({ port: 8080 });
4
5wss.on('connection', ws => {
6 console.log('Client connected');
7
8 ws.on('message', message => {
9 console.log(`Received message: ${message}`);
10 ws.send(`Server received: ${message}`);
11 });
12
13 ws.on('close', () => {
14 console.log('Client disconnected');
15 });
16
17 ws.on('error', error => {
18 console.error(`WebSocket error: ${error}`);
19 });
20
21 ws.send('Welcome to the WebSocket server!');
22});
23
24console.log('WebSocket server started on port 8080');
25
Handling Connections
The
connection
event is triggered when a new client connects to the server. Inside the callback, you can register event listeners for message
, close
, and error
events on the WebSocket instance (ws
).Sending and Receiving Messages
The
message
event is triggered when the server receives a message from the client. The ws.send()
method is used to send messages back to the client. Data is typically transmitted as strings or binary data.Advanced Node.js Websocket Server Techniques
Handling Multiple Clients
To manage multiple clients effectively, you can store connected WebSocket instances in an array or a Map. This allows you to iterate through the clients and broadcast messages to all connected users.
Javascript
1const WebSocket = require('ws');
2
3const wss = new WebSocket.Server({ port: 8080 });
4const clients = new Set();
5
6wss.on('connection', ws => {
7 console.log('Client connected');
8 clients.add(ws);
9
10 ws.on('message', message => {
11 console.log(`Received message: ${message}`);
12 clients.forEach(client => {
13 if (client !== ws && client.readyState === WebSocket.OPEN) {
14 client.send(`Broadcast: ${message}`);
15 }
16 });
17 ws.send(`Server received: ${message}`);
18 });
19
20 ws.on('close', () => {
21 console.log('Client disconnected');
22 clients.delete(ws);
23 });
24
25 ws.on('error', error => {
26 console.error(`WebSocket error: ${error}`);
27 clients.delete(ws);
28 });
29
30 ws.send('Welcome to the WebSocket server!');
31});
32
33console.log('WebSocket server started on port 8080');
34
Implementing Broadcasting
Broadcasting involves sending a message to all connected clients. The above code snippet already provides an example of broadcasting to every client except the client that send the message.
Error Handling and Robustness
Proper error handling is crucial for maintaining a stable WebSocket server. Use
try-catch
blocks to handle potential errors and implement logging to track issues.Javascript
1const WebSocket = require('ws');
2
3const wss = new WebSocket.Server({ port: 8080 });
4
5wss.on('connection', ws => {
6 console.log('Client connected');
7
8 ws.on('message', message => {
9 try {
10 console.log(`Received message: ${message}`);
11 ws.send(`Server received: ${message}`);
12 } catch (error) {
13 console.error(`Error processing message: ${error}`);
14 ws.send(`Error: ${error}`);
15 }
16 });
17
18 ws.on('close', () => {
19 console.log('Client disconnected');
20 });
21
22 ws.on('error', error => {
23 console.error(`WebSocket error: ${error}`);
24 });
25
26 ws.send('Welcome to the WebSocket server!');
27});
28
29console.log('WebSocket server started on port 8080');
30
Security Considerations
- Use TLS/SSL: Secure your WebSocket connection using
wss://
to encrypt data in transit. - Input Validation: Sanitize and validate all data received from clients.
- Authentication and Authorization: Implement authentication mechanisms to verify client identities and authorization to control access to resources.
- Rate Limiting: Prevent abuse by limiting the number of messages a client can send.
Scaling your Node.js Websocket Server
Horizontal Scaling
Scale your WebSocket server horizontally by running multiple instances behind a load balancer. This distributes the workload across multiple servers, increasing capacity and resilience.
Load Balancing
Use a load balancer to distribute incoming WebSocket connections across multiple server instances. Popular load balancers include Nginx, HAProxy, and cloud-based solutions like AWS Elastic Load Balancer.
Clustering
Node.js's built-in
cluster
module allows you to create multiple worker processes that share the same server port. This can improve performance by utilizing multiple CPU cores. However, WebSockets are stateful, so you will also need a method for sharing state. Using Redis or a similar technology can handle this. Here is an example of a diagram that illustrates how this could work.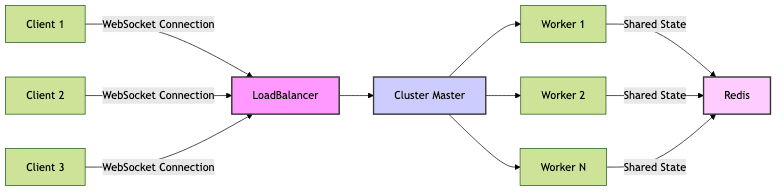
Real-World Applications of Node.js Websocket Servers
Chat Applications
WebSockets are ideal for building real-time chat applications where messages need to be delivered instantly.
Real-time Collaboration Tools
Applications like collaborative document editors and project management tools benefit from WebSockets for real-time updates and synchronization.
Game Development
WebSockets enable real-time multiplayer gaming experiences by providing low-latency communication between players and the game server.
IoT Applications
WebSockets can facilitate real-time data streaming and control between IoT devices and a central server.
Deploying Your Node.js Websocket Server
Choosing a Deployment Platform
Popular deployment platforms for Node.js applications include:
- Heroku: A platform-as-a-service (PaaS) that simplifies deployment.
- AWS Elastic Beanstalk: A PaaS offering from Amazon Web Services.
- DigitalOcean: A cloud infrastructure provider.
- Docker: Containerization platform for consistent deployments.
Deployment Steps
- Prepare your application: Ensure your application is configured for production.
- Choose a deployment platform: Select a platform that meets your requirements.
- Configure your environment: Set up environment variables and dependencies.
- Deploy your application: Deploy the code to the chosen platform.
- Monitor your application: Track performance and errors.
Conclusion
Node.js provides a powerful and efficient platform for building real-time applications using WebSockets. By understanding the fundamentals of WebSocket communication, implementing robust error handling, and considering security and scalability, you can create high-performance WebSocket servers that meet the demands of modern real-time applications.
Further Resources
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ