WebRTC vs. RTSP: A Comprehensive Comparison
Introduction: Choosing the Right Video Streaming Protocol
In today's world, real-time video streaming is more important than ever. From video conferencing and online gaming to live events and surveillance systems, the demand for low-latency, high-quality video experiences is constantly growing. Choosing the right video streaming protocol is crucial for delivering these experiences effectively. This article provides a comprehensive comparison of two popular protocols: WebRTC and RTSP, helping you determine which one best suits your specific needs.
Understanding the Need for Real-Time Video
Real-time video enables immediate interaction and information delivery. Unlike pre-recorded video, real-time video requires protocols that can transmit data with minimal delay, ensuring a smooth and responsive user experience. Factors like latency, bandwidth, and reliability play critical roles in the success of real-time video applications.
WebRTC: Peer-to-Peer Communication for Real-Time Applications
WebRTC (Web Real-Time Communication) is an open-source project that provides real-time communication capabilities to web browsers and mobile applications via simple APIs. It enables peer-to-peer communication, minimizing latency and maximizing efficiency for real-time applications.
Core Functionality and Architecture
WebRTC operates on a peer-to-peer architecture, allowing direct communication between devices without the need for a central server (although a signaling server is required for initial connection setup). This reduces latency and improves performance. The core components of WebRTC include:
- MediaStream: Captures audio and video data.
- RTCPeerConnection: Establishes and manages peer-to-peer connections.
- Data Channels: Enables arbitrary data transfer between peers.
Here's a simplified diagram illustrating the WebRTC process:
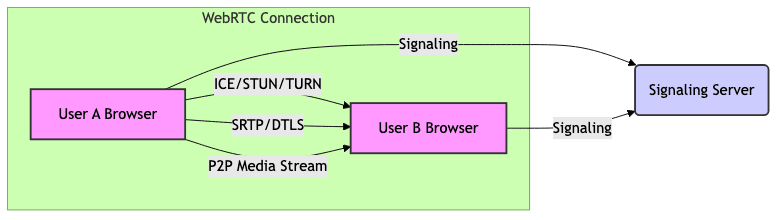
The signaling process facilitates the exchange of metadata (SDP) between peers, using protocols like Session Description Protocol (SDP). ICE (Interactive Connectivity Establishment) then handles NAT traversal using STUN (Session Traversal Utilities for NAT) and TURN (Traversal Using Relays around NAT) servers when direct peer-to-peer connection is not possible.
javascript
1// Example WebRTC signaling (simplified)
2
3// Peer A
4const peerA = new RTCPeerConnection();
5peerA.onicecandidate = (event) => {
6 if (event.candidate) {
7 // Send candidate to Peer B via signaling server
8 signal(JSON.stringify(event.candidate));
9 }
10};
11
12peerA.createOffer()
13 .then(offer => peerA.setLocalDescription(offer))
14 .then(() => {
15 // Send offer to Peer B via signaling server
16 signal(JSON.stringify(peerA.localDescription));
17 });
18
19// Peer B
20const peerB = new RTCPeerConnection();
21peerB.onicecandidate = (event) => {
22 if (event.candidate) {
23 // Send candidate to Peer A via signaling server
24 signal(JSON.stringify(event.candidate));
25 }
26};
27
28// Receive offer from Peer A
29peerB.setRemoteDescription(new RTCSessionDescription(JSON.parse(receivedOffer)))
30 .then(() => peerB.createAnswer())
31 .then(answer => peerB.setLocalDescription(answer))
32 .then(() => {
33 // Send answer to Peer A via signaling server
34 signal(JSON.stringify(peerB.localDescription));
35 });
36
37// After exchanging offers and candidates, the connection is established
38
Key Features and Advantages
- Low Latency: WebRTC's peer-to-peer architecture minimizes latency, making it ideal for real-time applications. The Real-time Transport Protocol (RTP) plays a key role in providing the low latency.
- Browser Compatibility: WebRTC is natively supported by most modern web browsers, eliminating the need for plugins or external software.
- Built-in Security (SRTP/DTLS): WebRTC uses SRTP (Secure Real-time Transport Protocol) and DTLS (Datagram Transport Layer Security) to encrypt media streams and protect against eavesdropping.
- Peer-to-peer Architecture: Direct communication between peers reduces server load and minimizes latency.
Limitations and Use Cases
- Scalability Challenges for Large Audiences: WebRTC's peer-to-peer architecture can be challenging to scale for large audiences, as each peer needs to maintain connections with multiple other peers. Selective Forwarding Units (SFUs) can help mitigate this issue.
- Complex Implementation: Implementing WebRTC can be complex, requiring expertise in signaling, NAT traversal, and media encoding. There are many libraries to use for WebRTC implementation.
- NAT Traversal Issues (STUN/TURN): NAT (Network Address Translation) can prevent direct peer-to-peer connections, requiring the use of STUN and TURN servers to relay traffic.
WebRTC excels in applications requiring low latency and real-time interaction, such as video conferencing, online gaming, and remote control systems.
RTSP: Server-Based Streaming for Reliable Delivery
RTSP (Real Time Streaming Protocol) is a network control protocol designed for use in entertainment and communications systems to control streaming media servers. It allows clients to remotely control playback of media files stored on a server.
Core Functionality and Architecture
RTSP follows a client-server architecture, where clients send control commands (e.g., PLAY, PAUSE, TEARDOWN) to a server that streams the media data. The media data is typically transmitted using RTP (Real-time Transport Protocol) over UDP or TCP.
python
1# Example using python and libVLC to stream an rtsp server
2import vlc
3
4# Create a vlc instance
5instance = vlc.Instance()
6
7# Create a media player
8player = instance.media_player_new()
9
10# Set the RTSP stream URL
11rtsp_url = "rtsp://your_rtsp_server/live"
12media = instance.media_new(rtsp_url)
13
14# Set the media to the player
15player.set_media(media)
16
17# Play the stream
18player.play()
19
20# Keep the script running to continue streaming
21# You might want to add some error handling and stop conditions
22import time
23time.sleep(60) # Stream for 60 seconds
24
25# Stop the player
26player.stop()
27
bash
1# Example RTSP client interaction using ffmpeg
2# To view an RTSP stream
3ffmpeg -i rtsp://your_rtsp_server/live -c copy -f flv rtmp://your_rtmp_server/live
4
5#To grab a single frame and save it as an image
6ffmpeg -i rtsp://your_rtsp_server/live -frames:v 1 output.jpg
7
8#You can use vlc as well
9vlc rtsp://your_rtsp_server/live
10
Key Features and Advantages
- Scalability: RTSP's server-based architecture makes it easier to scale for large audiences, as the server handles the distribution of media data to multiple clients.
- Established Infrastructure: RTSP has been around for a long time and is supported by a wide range of streaming servers, media players, and network devices.
- Compatibility with Existing Hardware: RTSP is compatible with a wide range of existing hardware, including IP cameras, encoders, and decoders.
Limitations and Use Cases
- Higher Latency: RTSP typically has higher latency compared to WebRTC, due to the server-based architecture and the use of TCP for control signaling.
- Less Browser Support: RTSP is not natively supported by most web browsers, requiring the use of plugins or external players. HTTP Live Streaming (HLS) and MPEG-DASH are often preferred for browser-based streaming.
- Security Concerns: RTSP does not provide built-in security features, requiring the use of additional measures such as encryption and authentication to protect media streams.
RTSP is well-suited for applications where scalability and reliable delivery are more important than low latency, such as video surveillance systems, digital signage, and IPTV.
Head-to-Head Comparison: WebRTC vs. RTSP
Let's compare WebRTC and RTSP across several key factors:
Latency
WebRTC offers significantly lower latency than RTSP, typically in the range of a few hundred milliseconds. RTSP latency can range from several seconds to tens of seconds, depending on network conditions and server configuration. For low-latency video streaming, WebRTC is superior.
Scalability
RTSP is more scalable than WebRTC, as it uses a server-based architecture that can handle a large number of concurrent clients. WebRTC's peer-to-peer architecture can become a bottleneck when scaling to large audiences, although SFUs can improve scalability.
Security
WebRTC provides built-in security features such as SRTP and DTLS, which encrypt media streams and protect against eavesdropping. RTSP requires additional security measures to protect media streams, such as encryption and authentication.
Cost
The cost of implementing WebRTC can be lower than RTSP, as it is an open-source project and does not require licensing fees. However, the complexity of implementing WebRTC can lead to higher development costs. RTSP may require licensing fees for certain streaming servers and media players.
Choosing the Right Protocol: Factors to Consider
Selecting between WebRTC and RTSP depends on your specific requirements.
Application Requirements
- Real-time Interaction: If your application requires real-time interaction and low latency, WebRTC is the better choice.
- Large Audiences: If you need to stream to a large audience, RTSP may be more suitable due to its scalability.
- Security: If security is a major concern, WebRTC's built-in security features provide a significant advantage.
Budget and Infrastructure
- Cost: Consider the cost of implementing and maintaining each protocol, including licensing fees, development costs, and infrastructure requirements.
- Existing Infrastructure: If you already have an existing RTSP infrastructure, it may be more cost-effective to stick with RTSP.
Technical Expertise
- Complexity: WebRTC can be more complex to implement than RTSP, requiring expertise in signaling, NAT traversal, and media encoding.
- Skillset: Ensure that your team has the necessary skills and expertise to implement and maintain the chosen protocol. There are many different libraries and platforms to help.
Future Trends and Emerging Technologies
Both WebRTC and RTSP continue to evolve with new features and capabilities being added regularly. Emerging technologies such as AV1 and WebTransport are expected to further enhance the performance and capabilities of these protocols. The introduction of HTTP3 promises more reliable streaming. Furthermore, adaptive bitrate streaming techniques are becoming crucial for optimizing the user experience across various network conditions. Technologies like Common Media Application Format (CMAF) seek to standardize low-latency streaming.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ