In today's digital landscape, WebRTC has emerged as the backbone of real-time communication applications, powering everything from video conferencing platforms to live streaming services. With over 2 billion devices supporting WebRTC globally and its adoption growing at an unprecedented rate, developers are increasingly relying on this technology to build seamless communication experiences.
However, the power of WebRTC comes with its share of complexities. Network inconsistencies, browser compatibility issues, and security concerns can quickly transform a promising application into a frustrating user experience. Whether you're a seasoned developer or just starting with WebRTC implementation, knowing how to test, diagnose, and fix common issues is crucial for application success.
This guide explores comprehensive online testing methodologies, troubleshooting approaches for audio/video quality issues, connection problems, and security vulnerabilities – all designed to help you create robust WebRTC applications that withstand real-world challenges.
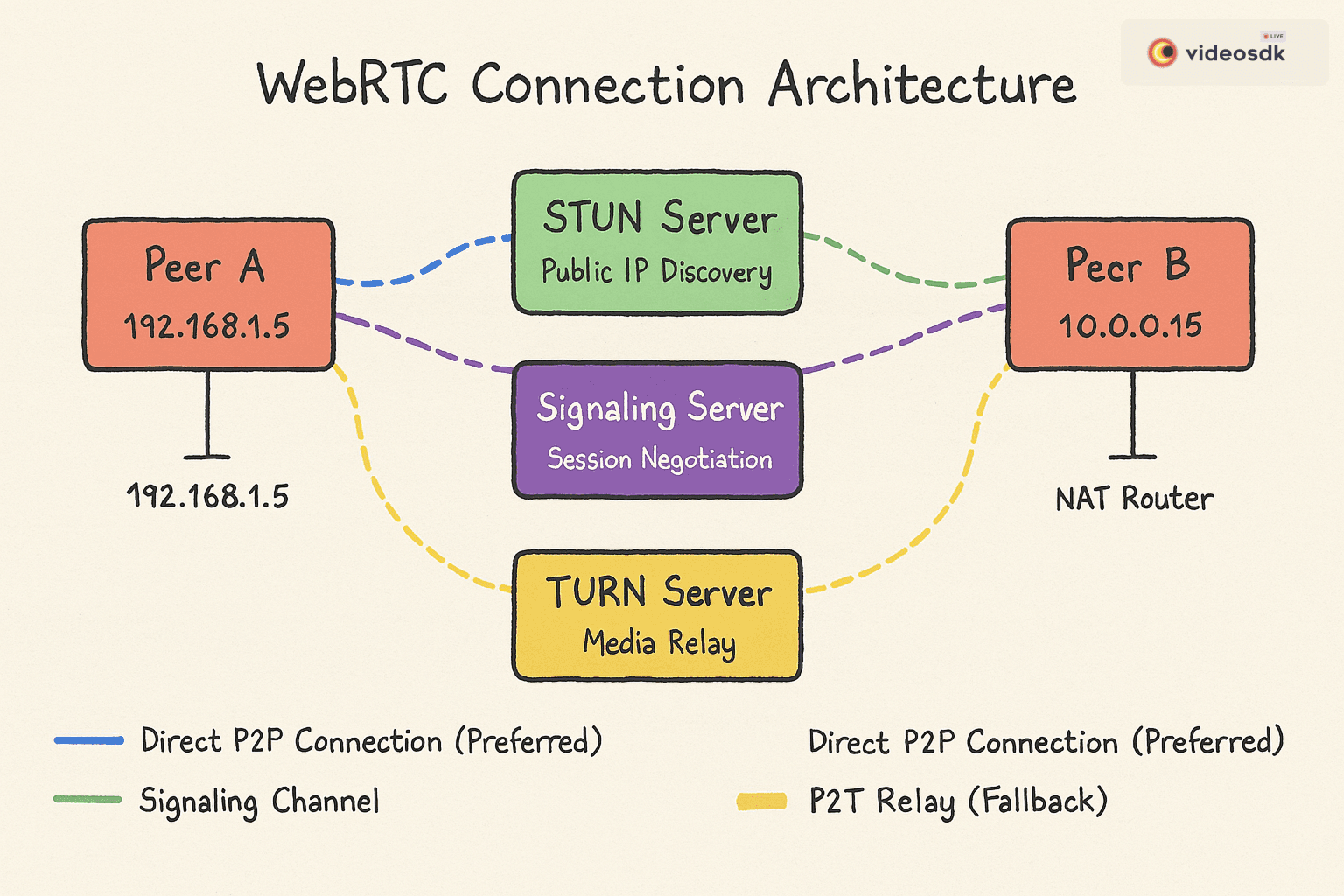
Why WebRTC Testing is Crucial for Application Success
Testing WebRTC applications isn't just a development phase checkbox – it's an ongoing necessity that directly impacts your application's success. Thorough testing ensures smooth user experiences by identifying potential issues before they reach your users. When real-time communication is your product's core functionality, even minor glitches can significantly impact user perception.
Neglecting proper testing can lead to severe consequences:
- Unpredictable audio/video quality causing user frustration
- Frequent connection drops leading to abandoned sessions
- Security vulnerabilities potentially exposing sensitive communications
- Compatibility issues across browsers resulting in fragmented user experiences
WebRTC testing encompasses several critical areas:
- Functional testing to ensure core features work as expected
- Performance testing under various network conditions
- Security testing to protect user privacy and data
- Compatibility testing across different browsers and devices
Implementing a comprehensive testing strategy helps you build confidence in your application's reliability while establishing a foundation for scaling with growing user demands.
Testing WebRTC Applications Online: Available Tools and Techniques
Online testing tools have revolutionized how developers approach WebRTC troubleshooting, offering accessible solutions without complex setup requirements. These tools provide valuable insights into your application's performance across different scenarios.
Essential Online WebRTC Testing Resources
- WebRTC Troubleshooter (test.webrtc.org) This Google-developed tool runs comprehensive tests covering camera/microphone access, resolution capabilities, bandwidth requirements, and connectivity checks. It's particularly useful for quick diagnostics during development stages.
- Trickle ICE Test (webrtc.github.io/samples/src/content/peerconnection/trickle-ice) This specialized tool helps test ICE gathering process and connectivity through STUN/TURN servers – crucial for understanding connection establishment issues.
- AppRTC (appr.tc) A reference WebRTC application that allows testing real-world scenarios between two browsers, providing metrics on packet loss, bandwidth usage, and connection quality.
- Network Link Conditioner Though not strictly an online tool, this utility simulates various network conditions (packet loss, latency, bandwidth restrictions) to test how your WebRTC application performs under challenging scenarios.
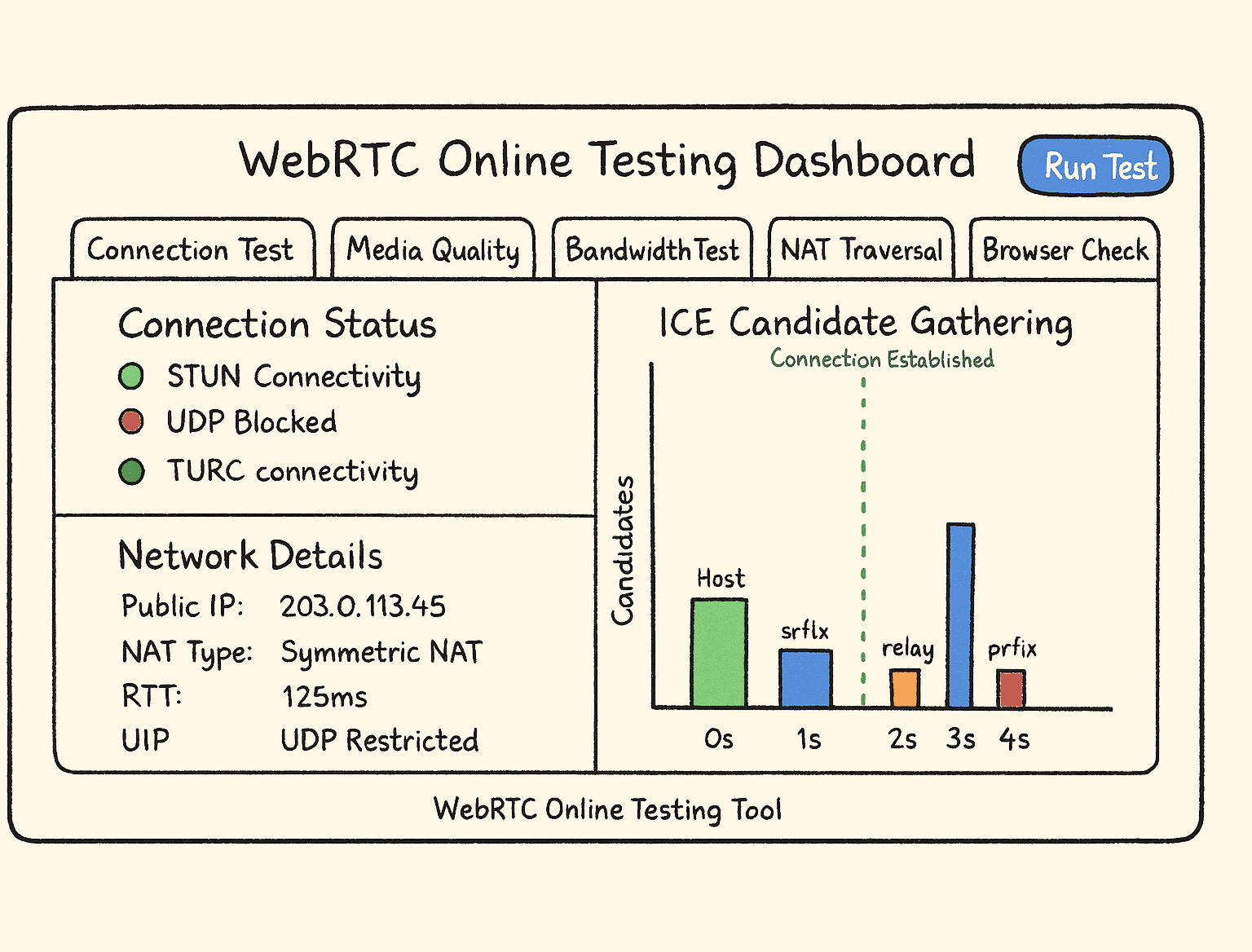
Testing techniques particularly effective with these tools include:
- Simulating different network conditions to stress-test adaptability
- Conducting A/B tests of different configurations to optimize performance
- Performing cross-browser compatibility tests to ensure consistent experience
For enterprise-level applications, consider commercial testing platforms like Testrtc or Arrivy, which offer more advanced features including automated testing and detailed analytics.
Diagnosing Common WebRTC Audio and Video Quality Issues
Identifying the Root Cause of Audio/Video Problems
Audio and video quality issues in WebRTC applications can stem from multiple sources, making systematic troubleshooting essential. Common symptoms users experience include:
- Video freezing or pixelation during calls
- Audio cutting out or sounding robotic
- Misalignment between audio and video streams
- Echo or feedback on audio channels
- High latency causing conversation delays
These symptoms typically result from one or more of these factors:
- Network limitations (insufficient bandwidth, high packet loss, jitter)
- Hardware constraints (camera/microphone quality, CPU limitations)
- Software configuration issues (incorrect codec settings, inefficient processing)
- Browser implementation differences
The choice of codecs significantly impacts quality, with VP8/VP9 and H.264 for video and Opus for audio being the most widely supported options. Each offers different trade-offs between quality, compression efficiency, and compatibility.
Troubleshooting Steps for Audio/Video Quality
Follow this structured approach to diagnose and resolve quality issues:
- Verify Network Performance Use browser developer tools (WebRTC internals in Chrome) to check:
- Available bandwidth (minimum 500kbps for basic video)
- Packet loss percentage (should be under 2% for acceptable quality)
- Round-trip time (ideally under 150ms)
- Jitter buffer size (excessive values indicate network instability)
- Optimize Media Settings Adjust parameters based on network capabilities: ```javascript const videoConstraints = { width: { ideal: 640, max: 1280 }, height: { ideal: 480, max: 720 }, frameRate: { ideal: 24, max: 30 } };// For poor connections, implement dynamic quality scaling peerConnection.getLocalStreams()[0].getVideoTracks()[0].applyConstraints({ width: 320, height: 240, frameRate: 15 }); ```
- Implement Audio Processing Techniques Enable WebRTC's built-in audio enhancements:
javascript const audioConstraints = { echoCancellation: true, noiseSuppression: true, autoGainControl: true };
- Monitor Key Performance Metrics Implement a stats monitoring system to track:
- Frames per second (target: 24-30fps)
- Bitrate fluctuations
- Packet loss events
- CPU usage (excessive values indicate processing bottlenecks)
- Consider Simulcast for Varying Network Conditions Implement simulcast to generate multiple quality versions of the same stream, allowing participants to receive the highest quality their connection supports.
Resolving WebRTC Connection Issues: NAT Traversal, Firewalls, and More
Understanding WebRTC Connection Challenges
WebRTC's peer-to-peer architecture creates unique connectivity challenges, particularly in establishing direct connections between devices behind different networks. The primary obstacles include:
- NAT Traversal: Most internet users are behind Network Address Translation (NAT) devices, which mask their actual IP addresses and complicate direct connections.
- Firewall Restrictions: Corporate networks often implement strict firewall policies that block WebRTC traffic.
- Symmetric NATs: Some NAT configurations are particularly restrictive, making direct connections nearly impossible without relay servers.
WebRTC implements the Interactive Connectivity Establishment (ICE) protocol to overcome these challenges. ICE works by collecting and trying different connection candidates until finding the optimal path between peers.
This process relies on two critical server types:
- STUN (Session Traversal Utilities for NAT) servers help discover the public IP address and port of a device behind NAT.
- TURN (Traversal Using Relays around NAT) servers act as fallback relay points when direct connections can't be established, ensuring connectivity even in challenging network environments.
Steps to Diagnose and Fix Connection Problems
- Implement Proper ICE Configuration Always include multiple STUN servers and at least one TURN server:
javascript const configuration = { iceServers: [ { urls: 'stun:stun.l.google.com:19302' }, { urls: 'stun:stun1.l.google.com:19302' }, { urls: 'turn:your-turn-server.com:3478', username: 'username', credential: 'password' } ], iceTransportPolicy: 'all', iceCandidatePoolSize: 10 };
- Monitor ICE Connection States Implement listeners for connection state changes to diagnose issues:
javascript peerConnection.addEventListener('iceconnectionstatechange', (event) => { console.log('ICE connection state:', peerConnection.iceConnectionState); // Implement recovery logic for failed states });
- Check Firewall Configurations Ensure your application and network allow:
- UDP traffic on ports 19302-19309 (Google STUN)
- TCP/UDP traffic on port 3478 (TURN)
- WebSocket connections (typically port 443)
- Implement Connection Timeouts and Fallbacks Set reasonable timeouts for connection establishment:
javascript const connectionTimeout = setTimeout(() => { if (peerConnection.iceConnectionState !== 'connected') { // Implement fallback strategy (e.g., switch to backup TURN server) } }, 10000); // 10 seconds
- Use Trickle ICE Implement trickle ICE to start transmission as soon as viable candidates are found, rather than waiting for all candidates:
javascript peerConnection.addEventListener('icecandidate', event => { if (event.candidate) { // Send candidate to remote peer immediately signallingChannel.send(JSON.stringify({ 'candidate': event.candidate })); } });
- Consider Geographic Distribution of STUN/TURN Servers Deploy servers in multiple regions to reduce latency and improve connection success rates for global users.
Addressing WebRTC Security Concerns: Encryption and Authentication
WebRTC Security Vulnerabilities and Best Practices
WebRTC provides built-in security through mandatory encryption, but additional measures are necessary to ensure comprehensive protection:
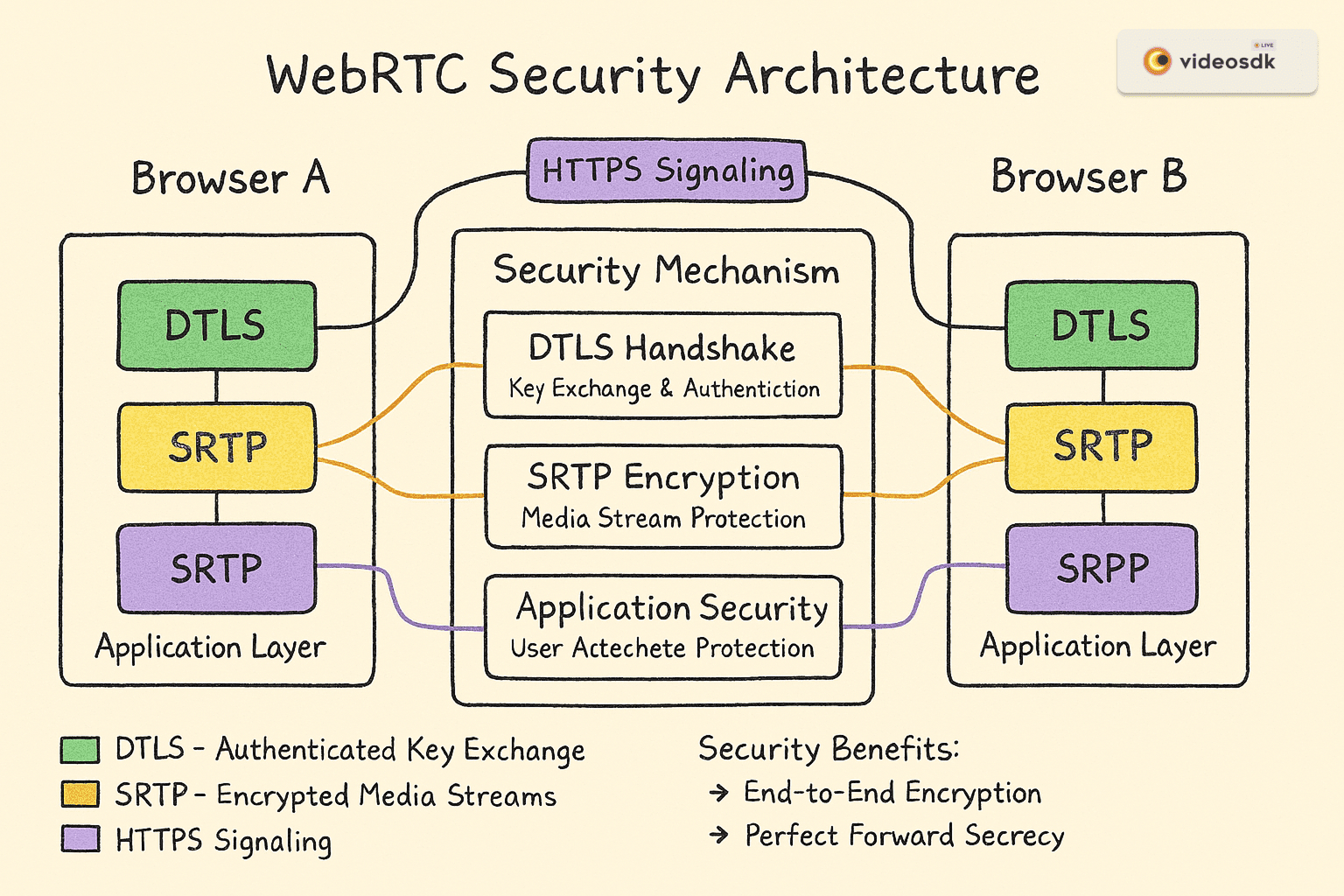
- Understand Built-in Protections WebRTC encrypts all media streams using DTLS-SRTP (Datagram Transport Layer Security for Secure Real-time Transport Protocol), providing protection against eavesdropping and tampering.
- Secure the Signaling Channel WebRTC doesn't specify how signaling should be implemented, making it a potential vulnerability:
- Always use HTTPS for signaling servers
- Implement token-based authentication
- Apply rate limiting to prevent abuse
- Implement Proper User Authentication Verify user identity before allowing connection establishment:
``javascript // Example using JWT for authentication async function connectToRoom(roomId, jwt) { try { const response = await fetch('/api/validate-access', { method: 'POST', headers: { 'Authorization':
Bearer ${jwt}` }, body: JSON.stringify({ roomId }) });1if (response.ok) { 2 // Proceed with WebRTC connection 3} else { 4 // Handle authentication failure 5} 6
} catch (error) { console.error('Authentication error:', error); } } ``` - Protect Against IP Address Leakage WebRTC can expose local IP addresses, which may be a privacy concern:
- Consider using the
{ iceTransportPolicy: 'relay' }
option to force TURN usage when privacy is critical - Implement user consent before accessing network interfaces
- Consider using the
Testing for Security Vulnerabilities Online
Several approaches help validate your WebRTC application's security posture:
- WebRTC-Leak Test Use online tools like BrowserLeaks.com to check if your implementation reveals private IP addresses.
- End-to-End Encryption Verification Implement and test verification mechanisms to ensure encryption is properly applied:
- Check for proper DTLS handshake completion
- Verify SRTP cipher suite negotiation
- Penetration Testing Consider specialized WebRTC security assessments to identify vulnerabilities in your implementation, focusing on:
- Signaling server security
- Authentication mechanisms
- Denial of service resistance
Optimizing WebRTC Performance for Different Network Conditions
Adapting to Varying Network Quality
WebRTC applications must gracefully handle diverse network environments, from high-speed corporate networks to unstable mobile connections.
- Implement Network Quality Monitoring Continuously track network metrics to inform adaptation decisions: ```javascript setInterval(() => { peerConnection.getStats().then(stats => { stats.forEach(report => { if (report.type = 'inbound-rtp' && report.kind = 'video') { const packetLoss = report.packetsLost / report.packetsReceived; const jitter = report.jitter;
1 // Based on these metrics, adapt media quality 2 if (packetLoss > 0.05 || jitter > 30) { 3 reduceMediaQuality(); 4 } else if (packetLoss < 0.01 && jitter < 10) { 5 increaseMediaQuality(); 6 } 7 } 8}); 9
}); }, 2000); ``` - Implement Adaptive Bitrate Control Dynamically adjust video quality based on available bandwidth: ```javascript function adjustBitrateBasedOnBandwidth(estimatedBandwidth) { const videoSender = peerConnection.getSenders() .find(sender => sender.track.kind === 'video');if (videoSender) { const parameters = videoSender.getParameters();
1// Don't proceed if there are no encoding parameters 2if (!parameters.encodings || parameters.encodings.length === 0) { 3 parameters.encodings = [{}]; 4}
// Set maximum bitrate based on estimated bandwidth // Use 70% of available bandwidth to allow for fluctuations const maxBitrate = Math.min(1500000, estimatedBandwidth * 0.7); parameters.encodings[0].maxBitrate = maxBitrate;1return videoSender.setParameters(parameters); 2
} } ``` - Implement Bandwidth Estimation Leverage WebRTC's built-in bandwidth estimation or implement custom logic:
javascript // Listen for bandwidth estimation events peerConnection.addEventListener('connectionstatechange', () => { if (peerConnection.connectionState === 'connected') { // Start bandwidth monitoring startBandwidthEstimation(); } });
- Prioritize Audio Over Video In constrained environments, maintain audio quality even at the expense of video:
javascript const transceiver = peerConnection.addTransceiver('video', { direction: 'sendrecv', streams: [localStream], sendEncodings: [{ maxBitrate: 100000, // Lower starting bitrate priority: 'low' // Lower priority than audio }] });
- Implement Stream Prioritization for Screen Sharing When sharing screens alongside camera feeds, adjust priorities based on content importance.
Key Takeaways
- Comprehensive Testing: Regular testing across different browsers, devices, and network conditions is essential for WebRTC application reliability.
- Connection Establishment: Proper STUN/TURN server configuration is critical for overcoming NAT and firewall restrictions.
- Quality Adaptation: Implementing dynamic quality adjustment based on network conditions significantly improves user experience.
- Security First: Despite WebRTC's built-in encryption, additional measures are necessary to ensure comprehensive protection.
- Performance Monitoring: Continuous monitoring of key metrics allows for early detection and resolution of potential issues.
Conclusion
WebRTC has transformed real-time communication on the web, but its power comes with implementation challenges that require careful testing and troubleshooting. By systematically addressing connectivity issues, optimizing media quality, and implementing security best practices, developers can create robust applications that deliver exceptional communication experiences across diverse network environments.
As WebRTC continues to evolve with new capabilities and improved performance, staying current with testing methodologies and diagnostic approaches will remain crucial for application success. The online testing tools and techniques covered in this guide provide a foundation for identifying and resolving common issues before they impact your users.
Remember that successful WebRTC applications aren't just technically sound—they're resilient to real-world conditions and optimized for the unpredictable environments where users will engage with your platform.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ