In today's digital landscape, real-time communication has become essential for businesses and applications seeking to provide immersive, interactive experiences. WebRTC (Web Real-Time Communication) has emerged as the leading technology powering this revolution, enabling direct browser-to-browser communication without plugins or additional software.
Despite its transformative potential, WebRTC presents a significant learning curve for many developers. This comprehensive guide will walk you through practical WebRTC demos, examples, and samples to help you understand the technology and build powerful real-world applications. Whether you're a seasoned developer or just getting started, this resource will equip you with the knowledge to implement WebRTC in your projects.
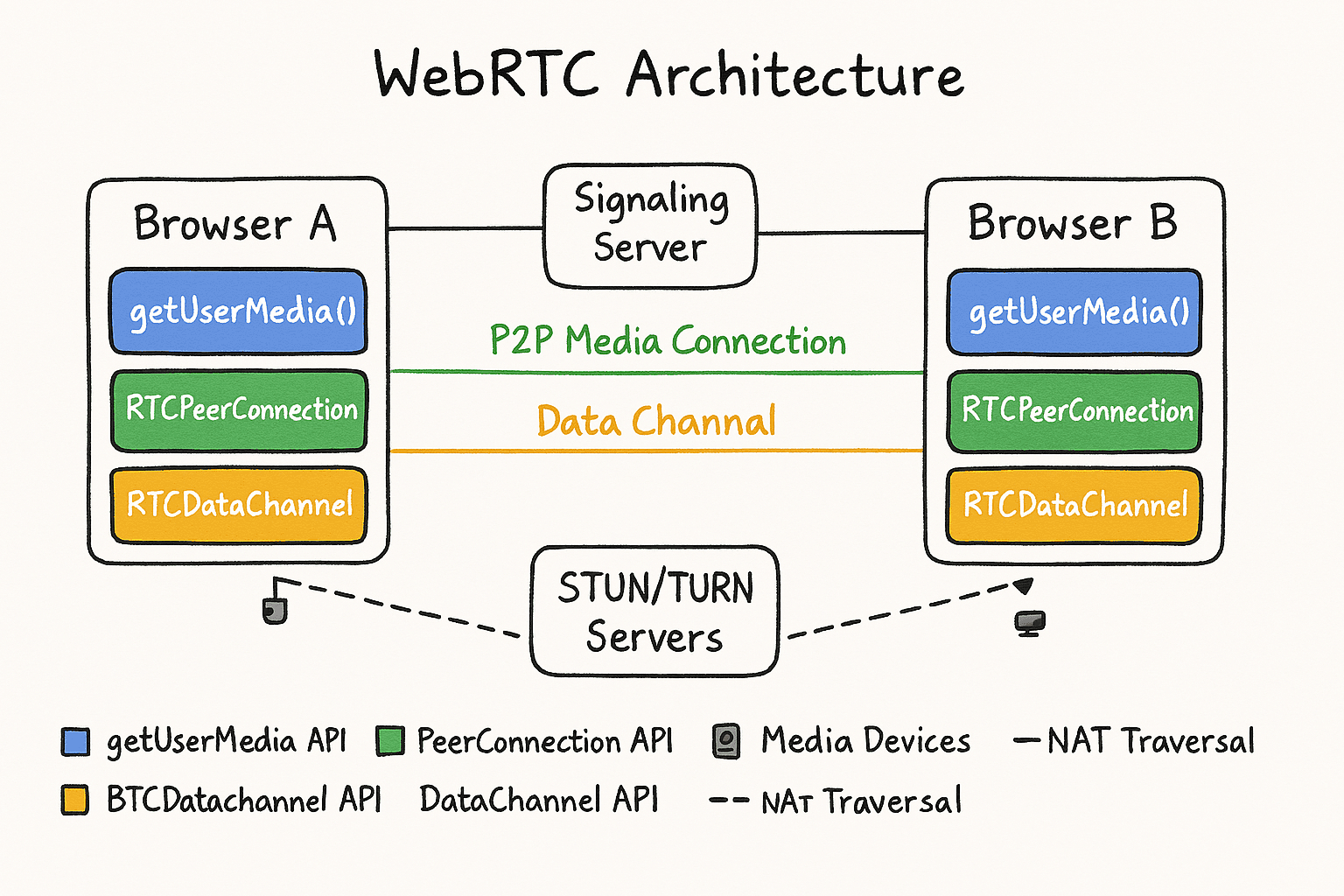
Understanding WebRTC Fundamentals
What is WebRTC?
WebRTC
is an open-source project that provides browsers and mobile applications with real-time communication capabilities through simple APIs. It enables peer-to-peer audio, video, and data sharing directly between browsers without requiring plugins, downloads, or third-party software.Key benefits include:
- Low latency communication
- Peer-to-peer architecture reducing server costs
- Cross-platform support
- Strong security through mandatory encryption
- Open-source implementation
Core WebRTC APIs
WebRTC revolves around three primary JavaScript APIs:
getUserMedia()
: Accesses a user's camera and microphone after permission is granted.RTCPeerConnection
: Establishes and manages connections between peers, handling codec negotiation, NAT traversal, and more.RTCDataChannel
: Enables bidirectional data transfer between peers for text, files, or any application data.
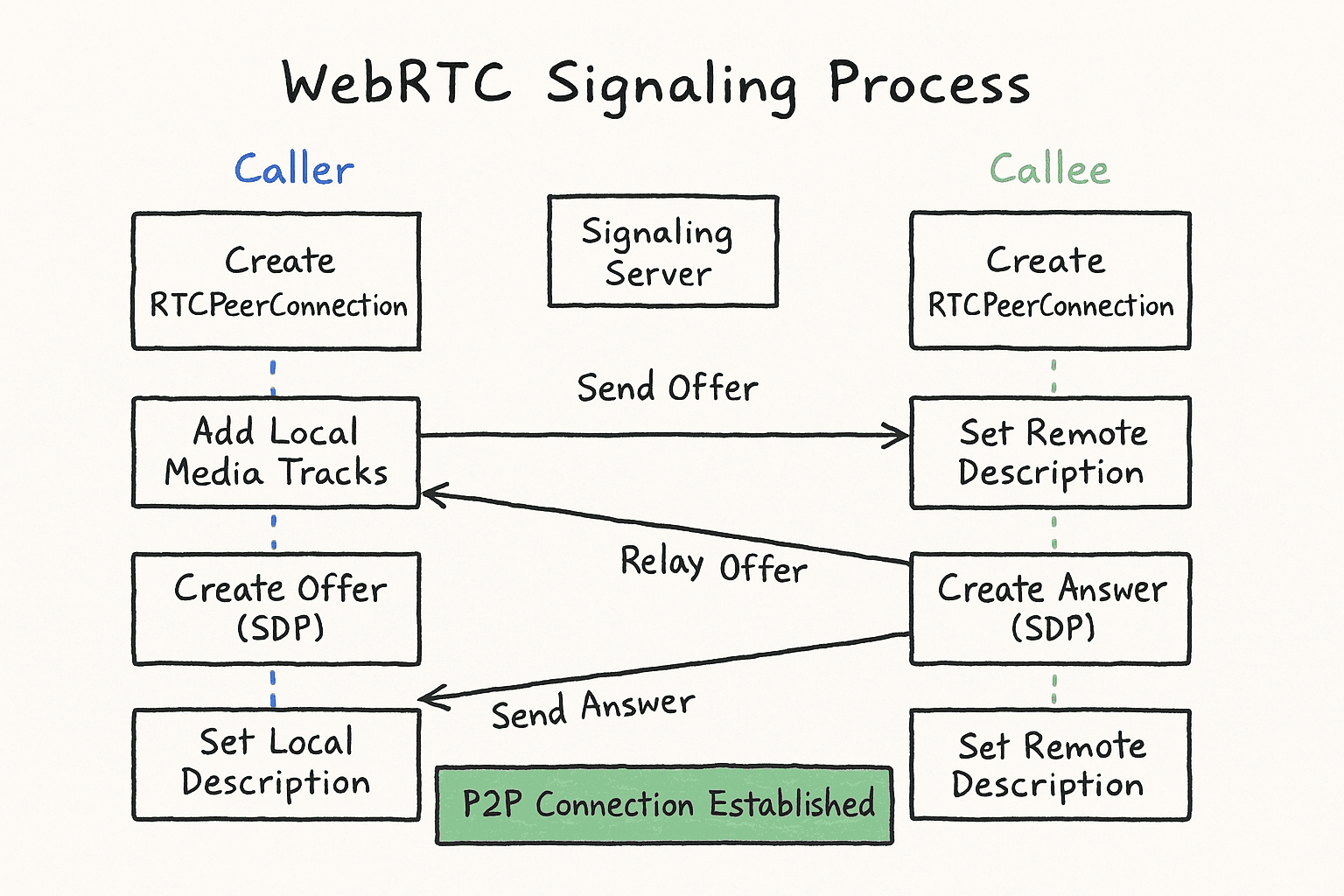
WebRTC Architecture Overview
WebRTC's architecture involves several components working together:
- Signaling: While not part of the WebRTC specification itself, signaling is necessary for peers to exchange metadata and establish connections.
- STUN/TURN servers: Help peers discover their public IP addresses and facilitate connections through firewalls and NATs.
- ICE (Interactive Connectivity Establishment): A framework that finds the optimal path for connecting peers.
Diving into WebRTC Demos and Examples: The Official Samples
The webrtc/samples
GitHub Repository
The official WebRTC project maintains a valuable repository of demos and examples that showcase various aspects of the technology. This repository serves as the go-to resource for developers looking to understand WebRTC implementation.
To run these samples locally:
git clone https://github.com/webrtc/samples.git
cd samples
npm install
npm start
Exploring getUserMedia()
Demos
Basic Camera Access Example
Here's a simple example of accessing a user's camera:
1async function startCamera() {
2 try {
3 const stream = await navigator.mediaDevices.getUserMedia({
4 video: true,
5 audio: false
6 });
7 document.getElementById('localVideo').srcObject = stream;
8 } catch (error) {
9 console.error('Error accessing camera:', error);
10 }
11}
12
Advanced Camera Controls
The official samples demonstrate how to:
- Apply filters using CSS and canvas elements
- Set camera constraints (resolution, frame rate)
- Select specific devices when multiple cameras/microphones are available
Screen Sharing with getDisplayMedia
1async function startScreenShare() {
2 try {
3 const stream = await navigator.mediaDevices.getDisplayMedia({
4 video: true
5 });
6 document.getElementById('screenShare').srcObject = stream;
7 } catch (error) {
8 console.error('Error sharing screen:', error);
9 }
10}
11
Unpacking RTCPeerConnection
Examples
The repository contains various examples that demonstrate peer connections:
Basic Peer Connection
A simplified example of establishing a peer connection:
1// Creating peer connection objects
2const pc1 = new RTCPeerConnection();
3const pc2 = new RTCPeerConnection();
4
5// Adding tracks from local stream to peer connection
6localStream.getTracks().forEach(track => {
7 pc1.addTrack(track, localStream);
8});
9
10// Handling ICE candidates
11pc1.onicecandidate = e => {
12 if (e.candidate) {
13 pc2.addIceCandidate(e.candidate);
14 }
15};
16
17pc2.onicecandidate = e => {
18 if (e.candidate) {
19 pc1.addIceCandidate(e.candidate);
20 }
21};
22
23// Handling incoming streams
24pc2.ontrack = e => {
25 document.getElementById('remoteVideo').srcObject = e.streams[0];
26};
27
28// Creating and setting the offer
29async function startConnection() {
30 const offer = await pc1.createOffer();
31 await pc1.setLocalDescription(offer);
32 await pc2.setRemoteDescription(offer);
33
34 const answer = await pc2.createAnswer();
35 await pc2.setLocalDescription(answer);
36 await pc1.setRemoteDescription(answer);
37}
38
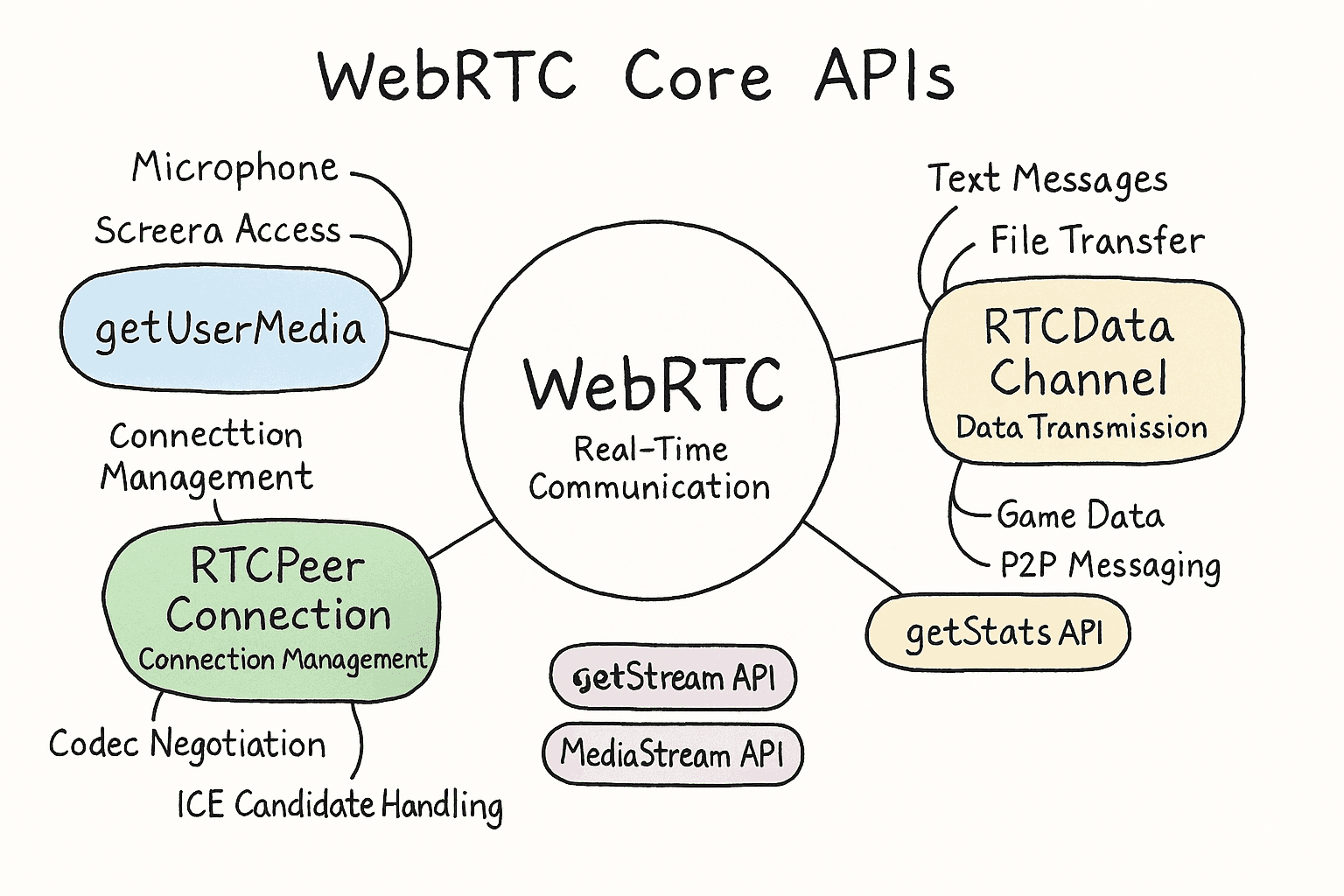
Additional examples in the repository cover:
- Audio-only connections
- Bandwidth adjustment
- ICE restart procedures
- Statistics monitoring
Utilizing RTCDataChannel
Samples
WebRTC isn't limited to audio and video—it also enables powerful data transfer capabilities:
1// Creating a data channel
2const dataChannel = peerConnection.createDataChannel('chat');
3
4// Setting up event handlers
5dataChannel.onopen = () => {
6 console.log('Data channel is open');
7};
8
9dataChannel.onmessage = event => {
10 console.log('Message received:', event.data);
11};
12
13// Sending a message
14function sendMessage(message) {
15 dataChannel.send(message);
16}
17
The official samples demonstrate:
- Text messaging between peers
- File transfers using chunked data
- Latency measurement
- Ordered vs. unordered message delivery
Advanced WebRTC Applications and Use Cases
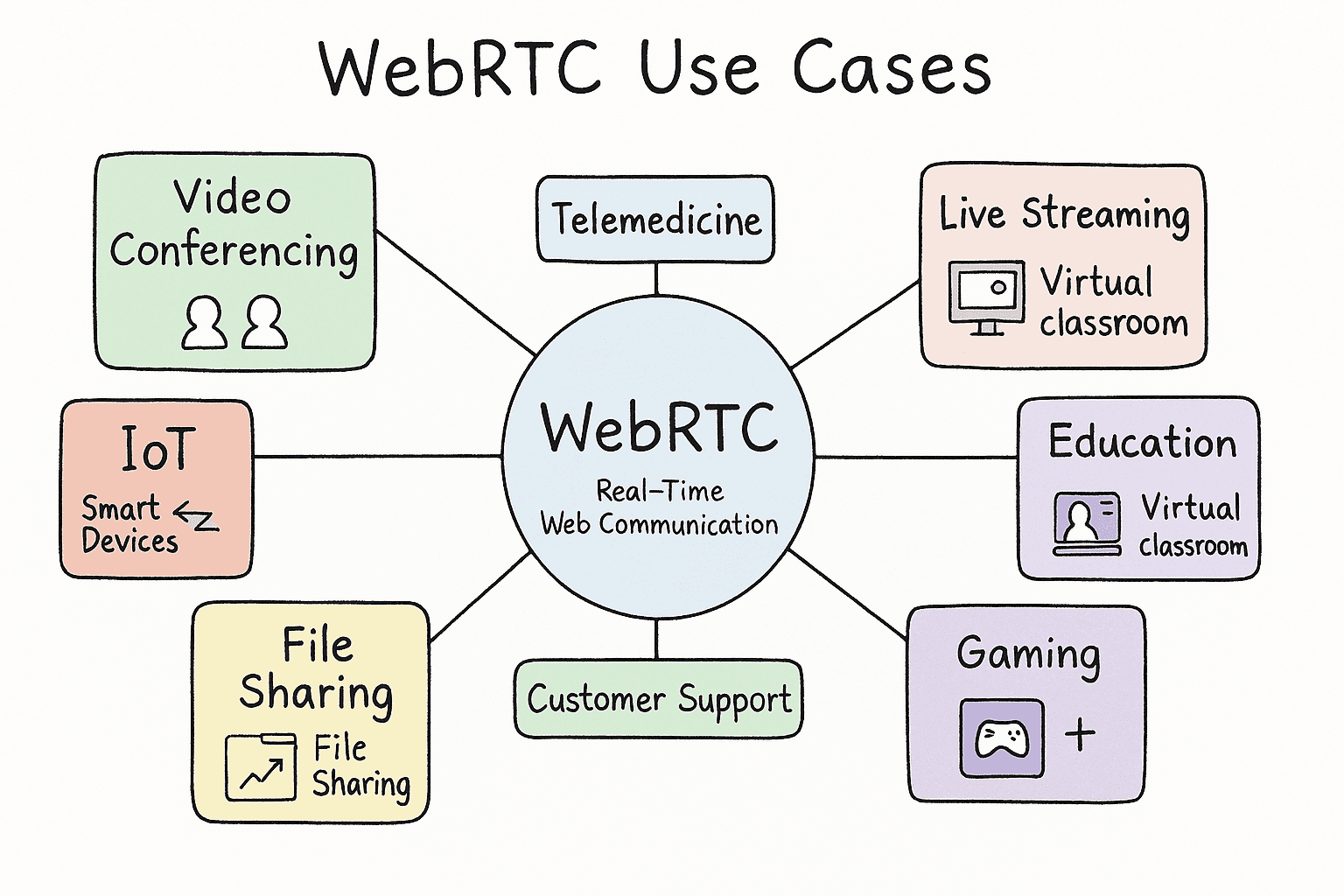
Building a Video Conferencing Application
Creating a full-featured video conferencing application requires handling multiple peers in a mesh or SFU (Selective Forwarding Unit) topology. Key considerations include:
- Scalability: Managing multiple peer connections efficiently
- Room management: Handling participants joining and leaving
- UI considerations: Layout switching, audio/video controls
- Quality adaptation: Adjusting resolution and bitrate based on network conditions
While building from scratch is educational, several libraries and frameworks can accelerate development:
- Jitsi (open-source, scalable)
- Janus WebRTC Gateway
- MediaSoup (SFU implementation)
Live Streaming with WebRTC
WebRTC enables sub-second latency live streaming, significantly outperforming traditional streaming protocols like HLS or DASH. Key components for WebRTC streaming include:
- Ingest servers to receive broadcaster streams
- Media servers for transcoding and distribution
- Scaling strategies for thousands of viewers
Data Streaming and P2P Applications
The
RTCDataChannel
API opens possibilities beyond audio/video:- Collaborative document editing with real-time updates
- Multiplayer gaming with low-latency data exchange
- P2P file sharing without server involvement
- Chat applications with end-to-end encryption
WebRTC and IoT: Connecting Devices in Real-Time
WebRTC is finding applications in IoT scenarios:
- Remote monitoring of camera feeds from IoT devices
- Voice commands to control smart home devices
- Real-time data collection from sensors
- Remote troubleshooting of equipment
Optimizing WebRTC Performance and Reliability
Understanding WebRTC Statistics (getStats API)
WebRTC provides detailed metrics through the
getStats()
API:1async function getConnectionStats(peerConnection) {
2 const stats = await peerConnection.getStats();
3
4 stats.forEach(report => {
5 if (report.type === 'inbound-rtp') {
6 console.log('Packets received:', report.packetsReceived);
7 console.log('Packets lost:', report.packetsLost);
8 }
9
10 if (report.type === 'candidate-pair' && report.state === 'succeeded') {
11 console.log('Current round-trip time:', report.currentRoundTripTime);
12 }
13 });
14}
15
These statistics help identify issues like:
- Packet loss
- Increased latency
- Low bandwidth
- CPU limitations affecting encoding/decoding
Troubleshooting Common WebRTC Issues
Common challenges with WebRTC include:
- NAT traversal problems: Ensuring proper STUN/TURN server configuration
- Codec compatibility: Handling different browser implementations
- Bandwidth constraints: Adapting to network limitations
- Signaling failures: Debugging connection establishment issues
Security Considerations
WebRTC implements mandatory encryption, but developers should still consider:
- Secure signaling channels (WebSockets over TLS)
- Authentication mechanisms for peers
- Permission models for accessing media devices
- Proper TURN server security configuration
Beyond the Basics: Exploring Advanced Features
Insertable Streams: Media Processing with WebRTC
The Insertable Streams API (relatively new) allows developers to:
- Implement custom end-to-end encryption
- Process video frames before rendering (facial recognition, AR effects)
- Apply custom audio processing (noise suppression, voice effects)
WebRTC and WebAssembly
Combining WebRTC with WebAssembly unlocks performance improvements:
- Accelerated video processing
- Custom codec implementations
- Complex real-time data analysis on media streams
Key Takeaways
WebRTC provides a powerful platform for building real-time communication applications:
- The official samples repository offers valuable learning resources
- Three core APIs (
getUserMedia
,RTCPeerConnection
,RTCDataChannel
) form the foundation - Understanding both basic and advanced concepts is key to successful implementation
- Performance optimization and troubleshooting require monitoring and statistics analysis
Conclusion
WebRTC continues to transform how we build interactive, real-time experiences on the web. By exploring the demos, examples, and samples outlined in this guide, you're equipped to leverage WebRTC's capabilities in your own projects.
Whether you're building a simple video chat application or a complex collaborative platform, the open-source nature of WebRTC provides both the flexibility and power to create exceptional user experiences. Start with the basics, experiment with the samples, and build upon them to create your own innovative applications.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ