Introduction to PHP Sockets
What are PHP Sockets?
PHP sockets provide a low-level interface for network communication. They allow your PHP applications to communicate directly with other applications or services over a network, using protocols like TCP/IP and UDP. Think of them as raw communication channels, giving you fine-grained control over data transmission and reception. Unlike higher-level protocols like HTTP handled by cURL, sockets operate closer to the metal, offering more flexibility and control but requiring a deeper understanding of networking concepts.
Why Use PHP Sockets?
PHP sockets offer several advantages:
- Control: You have direct control over the communication protocol and data format.
- Performance: For specific tasks, sockets can be more performant than higher-level abstractions.
- Real-time Communication: Sockets are ideal for real-time applications like chat servers or game servers.
- Custom Protocols: You can implement custom network protocols.
- Low-level Access: You can access and manipulate network headers and options.
When to Use PHP Sockets vs. Alternatives (e.g., cURL)
Consider using PHP sockets when:
- You need real-time, bidirectional communication.
- You're implementing a custom network protocol.
- You need fine-grained control over network communication.
- You are working on the back-end for a chat application or a game server.
Use cURL when:
- You're interacting with standard HTTP APIs.
- You need a simple way to fetch data from a web server.
- The overhead of socket management is not needed.
In essence, cURL is great for client-side HTTP requests, while sockets provide a foundation for building custom servers and handling real-time interactions.
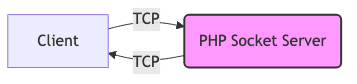
Setting up a Simple TCP/IP Server with PHP Sockets
Let's create a basic TCP/IP server using PHP sockets. This server will listen for incoming connections and echo back any data it receives.
Creating a Socket: socket_create()
The
socket_create()
function creates a socket resource. You need to specify the domain (e.g., AF_INET
for IPv4), type (e.g., SOCK_STREAM
for TCP), and protocol (e.g., SOL_TCP
).1<?php
2$socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP);
3if ($socket === false) {
4 echo "socket_create() failed: reason: " . socket_strerror(socket_last_error()) . "
5";
6 exit;
7}
8
9echo "Socket created successfully.
10";
11?>
12
Binding the Socket: socket_bind()
socket_bind()
assigns an address (IP address and port) to the socket. This tells the operating system which network interface and port the server should listen on.1<?php
2$host = '127.0.0.1'; //localhost
3$port = 12345;
4
5$result = socket_bind($socket, $host, $port);
6if ($result === false) {
7 echo "socket_bind() failed: reason: " . socket_strerror(socket_last_error($socket)) . "
8";
9 exit;
10}
11
12echo "Socket bound to $host:$port successfully.
13";
14?>
15
Listening for Connections: socket_listen()
socket_listen()
puts the socket into a listening state, waiting for incoming connection requests from clients. The second parameter specifies the maximum number of pending connections that can be queued.1<?php
2$result = socket_listen($socket, 3);
3if ($result === false) {
4 echo "socket_listen() failed: reason: " . socket_strerror(socket_last_error($socket)) . "
5";
6 exit;
7}
8
9echo "Socket listening on $host:$port.
10";
11?>
12
Accepting Connections: socket_accept()
socket_accept()
accepts an incoming connection request, creating a new socket resource for the connection. The original socket remains in the listening state.1<?php
2$spawn = socket_accept($socket);
3if ($spawn === false) {
4 echo "socket_accept() failed: reason: " . socket_strerror(socket_last_error($socket)) . "
5";
6 exit;
7}
8
9echo "Socket accepted successfully.
10";
11?>
12
Handling Client Connections and Communication
Once a client connection is accepted, you can use the new socket resource (
$spawn
in the example above) to communicate with the client.Receiving Data from Clients: socket_read()
socket_read()
reads data from the socket. You need to specify the socket resource and the maximum number of bytes to read. It's essential to handle the case where the client closes the connection.1<?php
2$input = socket_read($spawn, 2048);
3if ($input === false) {
4 echo "socket_read() failed: reason: " . socket_strerror(socket_last_error($spawn)) . "
5";
6 exit;
7}
8
9$input = trim($input);
10echo "Received data: $input
11";
12?>
13
Sending Data to Clients: socket_write()
socket_write()
sends data to the socket. You need to specify the socket resource and the data to send.1<?php
2$output = "PHP: You said '$input'
3";
4socket_write($spawn, $output, strlen($output));
5echo "Sent data: $output
6";
7?>
8
Closing Connections: socket_close()
socket_close()
closes the socket resource, releasing the resources associated with it. It's crucial to close the client socket after you're done communicating and also close the main socket after the server is done.1<?php
2socket_close($spawn);
3socket_close($socket);
4
5echo "Socket closed.
6";
7?>
8
Error Handling in PHP Sockets
Proper error handling is essential when working with sockets. Use
socket_last_error()
to retrieve the last socket error code and socket_strerror()
to get a human-readable error message.1<?php
2if ($result === false) {
3 $errorcode = socket_last_error($socket);
4 $errormessage = socket_strerror($errorcode);
5
6echo "Socket Error: [$errorcode] $errormessage
7";
8}
9?>
10
Building a Simple TCP/IP Client with PHP Sockets
Now, let's create a simple client that connects to the server we built earlier.
Connecting to the Server: socket_connect()
socket_connect()
attempts to establish a connection to the specified server address.1<?php
2$socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP);
3if ($socket === false) {
4 echo "socket_create() failed: reason: " . socket_strerror(socket_last_error()) . "
5";
6 exit;
7}
8
9$host = '127.0.0.1';
10$port = 12345;
11
12$result = socket_connect($socket, $host, $port);
13if ($result === false) {
14 echo "socket_connect() failed.
15Reason: (\$result) " . socket_strerror(socket_last_error($socket)) . "
16";
17 exit;
18}
19
20echo "Connected to server
21";
22?>
23
Sending and Receiving Data
The client sends data to the server and then receives the server's response.
1<?php
2$message = "Hello Server!
3";
4socket_write($socket, $message, strlen($message));
5echo "Sent to server :$message";
6
7$result = socket_read ($socket, 2048);
8echo "Reply from Server :" . $result;
9
10socket_close($socket);
11?>
12
Advanced PHP Socket Techniques
Working with UDP Sockets
UDP (User Datagram Protocol) is a connectionless protocol, unlike TCP. It's faster but less reliable. Use
SOCK_DGRAM
for the socket type.1<?php
2$socket = socket_create(AF_INET, SOCK_DGRAM, SOL_UDP);
3
4$host = '127.0.0.1';
5$port = 12345;
6$message = 'Hello UDP Server!';
7
8socket_sendto($socket, $message, strlen($message), 0, $host, $port);
9
10echo "Sent message to UDP server.
11";
12
13socket_close($socket);
14?>
15
Non-blocking Sockets
By default, socket operations are blocking. This means that the script will wait until the operation is complete. Non-blocking sockets allow you to perform other tasks while waiting for data. Use
socket_set_nonblock()
to switch to non-blocking mode.Asynchronous Socket Programming
Asynchronous socket programming involves using techniques like
socket_select()
to monitor multiple sockets simultaneously and handle events as they occur. This is essential for building scalable servers.Secure Sockets Layer (SSL/TLS) with PHP Sockets
To secure socket communication, use SSL/TLS encryption. This involves using functions like
stream_socket_enable_crypto()
to enable encryption on the socket.PHP Socket Frameworks and Libraries
Several PHP frameworks and libraries can simplify socket programming:
- ReactPHP: An event-driven, non-blocking I/O framework for PHP.
- Ratchet: A library for building real-time, bidirectional web applications using WebSockets.
- Swoole: An asynchronous, parallel, high-performance network communication engine for PHP.
Conclusion and Best Practices
PHP sockets provide a powerful tool for building custom network applications. While they require a deeper understanding of networking concepts, they offer unparalleled control and flexibility.
Further Reading:
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ