In today's digital landscape, businesses face a significant challenge: maintaining consistent user interfaces across multiple platforms. Whether it's iOS, Android, web, or emerging platforms, ensuring a unified brand experience while adapting to platform-specific requirements can be resource-intensive and complex. Server Driven UI (SDUI) offers a powerful solution to this dilemma by fundamentally changing how we architect and deliver user interfaces. This approach lets your backend control UI presentation, enabling greater flexibility, faster updates, and improved cross-platform consistency.
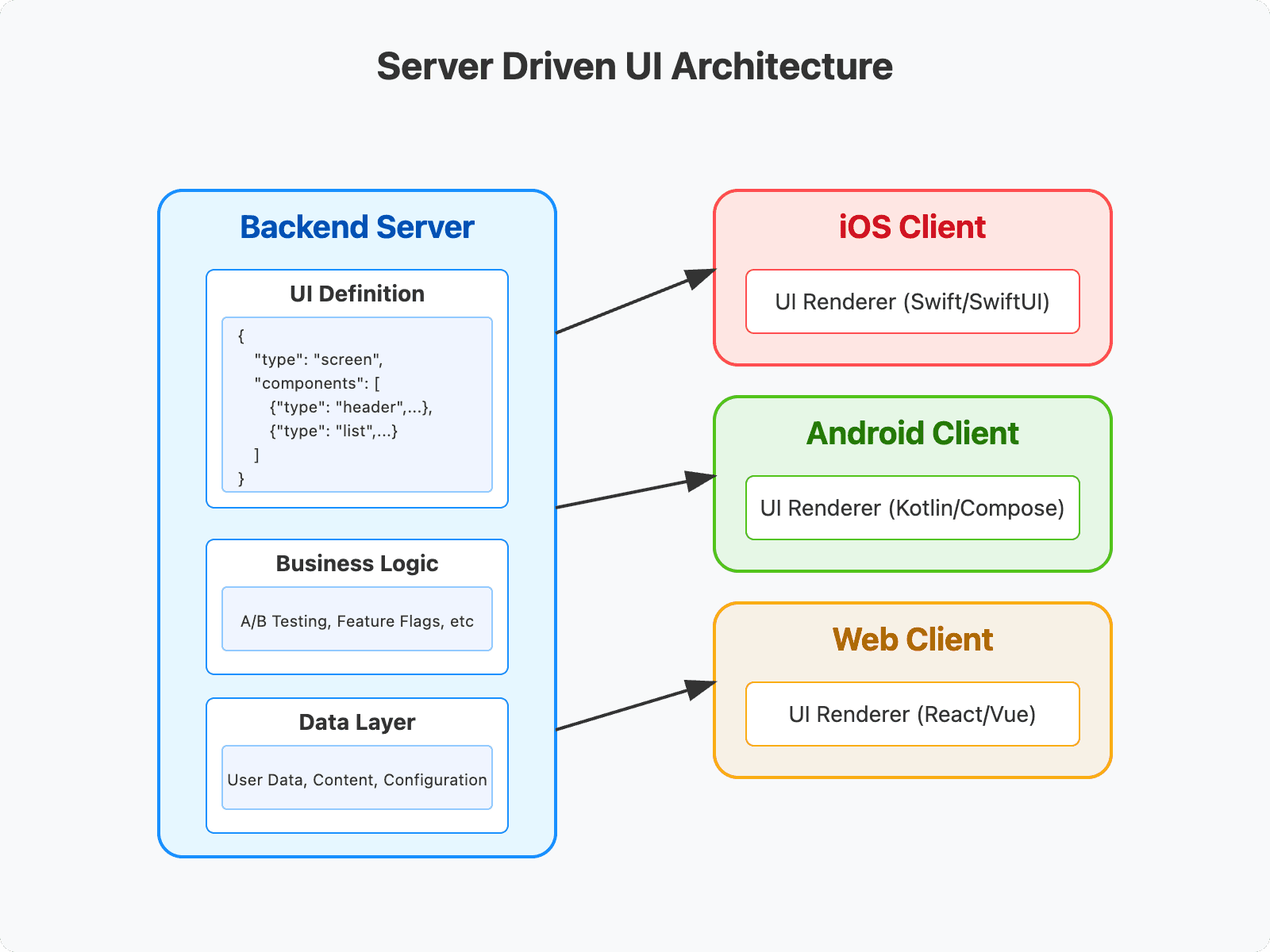
What is Server Driven UI?
Server Driven UI is an architectural approach where the backend server, rather than the client application, defines and controls how the user interface is rendered. In traditional client-driven UI architectures, the client application contains all the UI components and logic, with servers primarily providing data. The client decides how to present that data based on hard-coded UI implementations.
In contrast, SDUI shifts this responsibility to the server, which sends both the data and instructions on how to display it. The server provides a structured description of the UI (typically in JSON format) that the client interprets and renders using native components. This creates a more flexible system where UI changes can be deployed without requiring app updates.
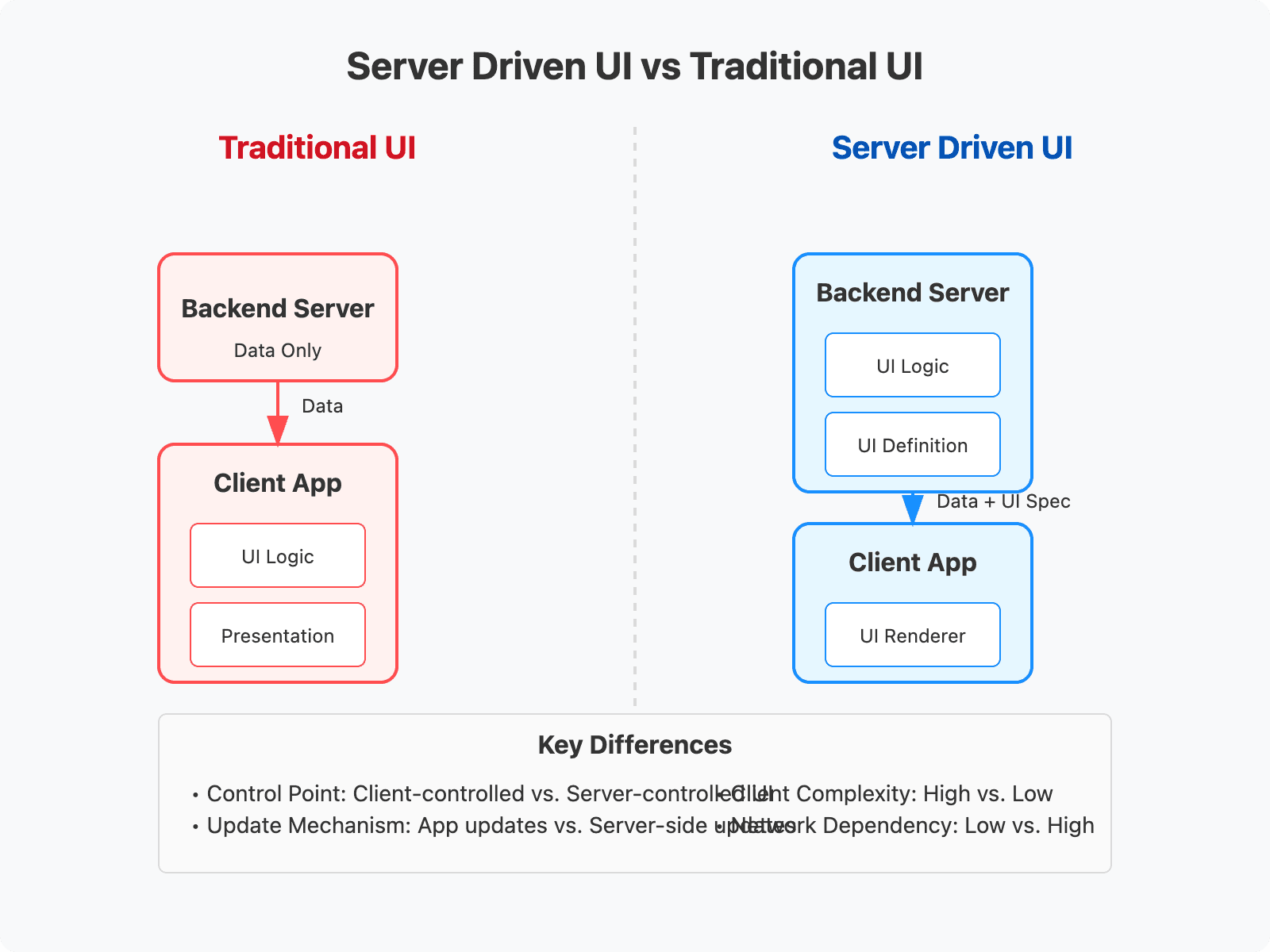
Key differences from traditional approaches:
- Backend Control: The server determines what UI components to display and how they should be arranged
- Dynamic UI: Client apps can receive and render new UI designs without app updates
- Thin Clients: Mobile and web applications focus primarily on rendering capabilities rather than business logic
- Component-Based Design: UI is broken down into reusable components that can be composed in different ways
Benefits of Server Driven UI for Cross-Platform Applications
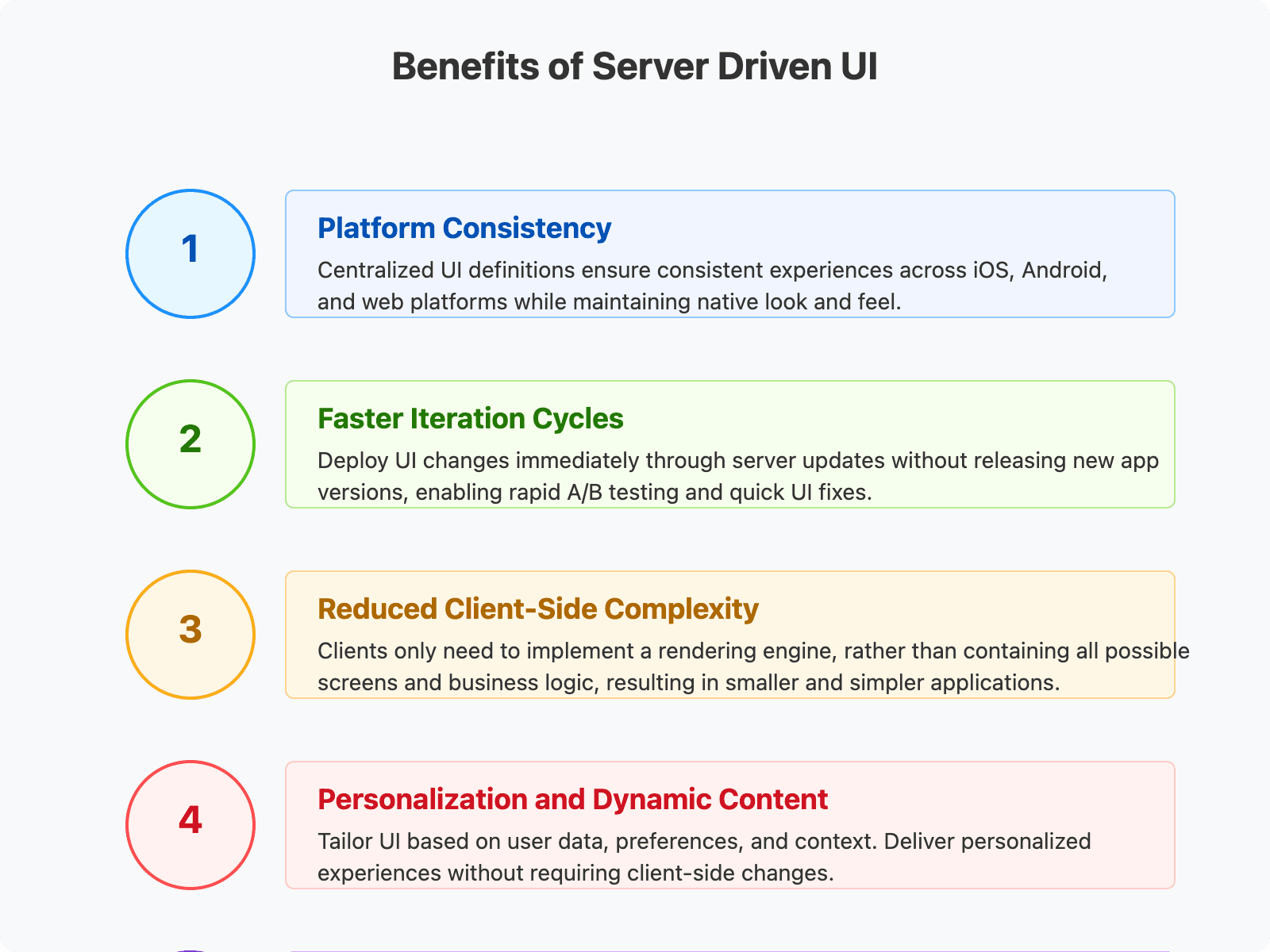
Platform Consistency
One of the most compelling benefits of SDUI is ensuring UI consistency across different platforms. Traditional development requires maintaining separate codebases and UI implementations for iOS, Android, and web platforms. This approach inherently leads to inconsistencies as teams work at different paces or interpret design specifications differently.
SDUI addresses this challenge by centralizing UI logic on the server. Since all platforms receive the same UI specification, the core experience remains consistent. Each platform translates these specifications into native components, maintaining platform-specific behaviors and optimizations while preserving the overall experience.
Faster Iteration Cycles
The traditional mobile app release process involves multiple steps: development, testing, app store review, and user adoption—often taking days or weeks. This slow cycle makes it difficult to quickly respond to user feedback, fix UI issues, or adapt to changing business requirements.
With SDUI, many UI changes can be deployed immediately through server updates without requiring a new app release. This capability enables:
- Rapid A/B testing of different UI layouts and components
- Immediate rollout of design improvements
- Quick resolution of UI-related bugs
- Phased feature launches with controlled rollouts
For example, an e-commerce company might want to test different product detail layouts during a sales event. With SDUI, they can deploy multiple variants simultaneously and measure performance in real-time, optimizing the experience without waiting for app updates.
Reduced Client-Side Complexity
By shifting UI logic to the server, SDUI significantly reduces client application complexity. Client apps primarily need to implement a rendering engine capable of interpreting the server's UI specifications, rather than containing all possible screens and business logic.
This approach results in:
- Smaller application size
- Reduced local code complexity
- Fewer platform-specific bugs
- Easier maintenance of client applications
- More focused development teams (server vs. client specialists)
Personalization and Dynamic Content
SDUI excels at delivering personalized experiences by allowing servers to tailor the UI based on user data, context, or business rules. Rather than requiring all personalization logic to be built into the client, the server can dynamically compose UI elements specific to each user.
For instance, a streaming service might show different content layouts based on viewing history, subscription tier, or device type. With SDUI, these personalized experiences can be implemented and refined without client-side changes.
Challenges of Implementing Server Driven UI
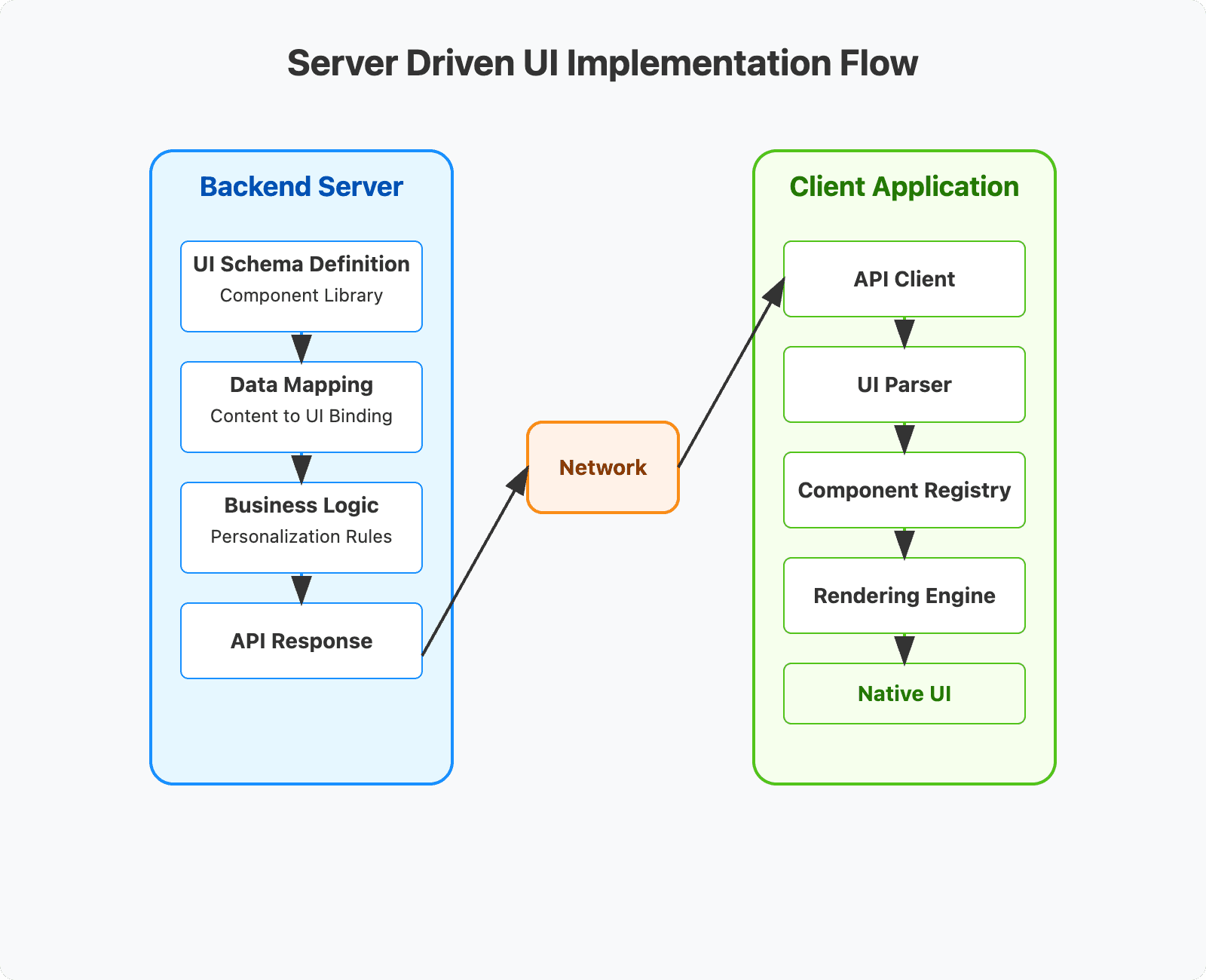
While SDUI offers numerous benefits, it also presents several challenges that organizations must address:
Performance Considerations
A primary concern with SDUI is potential performance impact, including:
- Network Dependency: SDUI requires network requests to fetch UI specifications, which can introduce latency, especially on slower connections
- Parsing Overhead: Client applications must parse and interpret UI specifications before rendering
- Rendering Complexity: Dynamic rendering can be more resource-intensive than static, pre-compiled UI
Mitigation strategies include:
- Implementing aggressive caching of UI specifications
- Using optimized native rendering engines
- Providing fallback UI options for offline scenarios
- Progressive loading of UI components
Complexity on the Backend
SDUI shifts much of the UI complexity from clients to the server, which introduces its own challenges:
- Schema Management: Maintaining a comprehensive UI component schema that evolves over time
- Increased Server Load: Additional processing required to generate UI specifications
- Cross-Platform Knowledge: Backend developers need awareness of client platform capabilities and limitations
- Testing Complexity: Testing UI across multiple platforms becomes a server-side responsibility
Organizations often need to invest in specialized tooling and processes to manage this increased backend complexity effectively.
Maintaining UI State
Managing UI state becomes more complicated in SDUI systems, particularly for interactive elements:
- State Synchronization: Keeping client and server state in sync during user interactions
- Partial Updates: Efficiently updating only the necessary portions of the UI
- Form Input: Managing form data and validation across the client-server boundary
- Interactive Elements: Handling complex interactive components like carousels, drag-and-drop interfaces, etc.
Solutions typically involve a combination of local state management and efficient state synchronization protocols.
Versioning and Backward Compatibility
As UI schemas evolve, maintaining backward compatibility becomes critical:
- Client Version Fragmentation: Users may be running different versions of the client application
- Schema Evolution: Adding new component types or properties without breaking existing clients
- Feature Detection: Clients need mechanisms to signal their capabilities to the server
- Graceful Degradation: Providing fallbacks when clients don't support newer components
Successful SDUI implementations require disciplined versioning strategies and careful consideration of compatibility.
Debugging and Testing
With logic split between client and server, debugging UI issues becomes more challenging:
- Root Cause Analysis: Determining whether issues originate in the client renderer or server specification
- End-to-End Testing: Testing the complete user experience across multiple platforms
- Development Environment: Creating efficient workflows for UI developers to test changes
- Monitoring: Detecting and alerting on UI rendering issues in production
Organizations often need specialized tools to record and replay UI specifications, visualize rendering, and trace issues across the client-server boundary.
Building a Server Driven UI Framework: Key Considerations
Choosing a Data Format
The format used to describe UI components is a fundamental decision in SDUI architecture. JSON is the most common choice due to its wide support, human readability, and efficient parsing. However, other options include:
- Protocol Buffers: More compact and strictly typed, but less human-readable
- GraphQL: Combines data and UI specification in a unified query language
- Custom Binary Formats: Maximum efficiency but typically lacks tooling and readability
Key considerations include:
- Parsing performance on target platforms
- Schema validation capabilities
- Human readability for debugging
- Size efficiency for network transfer
- Ecosystem support and tooling
Example JSON representation of a basic button component:
1{
2 "type": "button",
3 "properties": {
4 "text": "Submit",
5 "style": "primary",
6 "disabled": false
7 },
8 "actions": {
9 "onClick": {
10 "type": "submit_form",
11 "formId": "user_registration"
12 }
13 }
14}
15
Defining a UI Schema
A well-defined UI schema is essential for SDUI success. The schema defines all available components, their properties, and how they can be composed:
- Component Library: Define all available UI building blocks (buttons, cards, lists, etc.)
- Property Types: Specify available properties for each component type
- Validation Rules: Establish constraints on property values and component nesting
- Layout Systems: Define how components position relative to each other
- Action Handlers: Specify available interaction behaviors
Successful schemas balance completeness with flexibility, allowing for a wide range of UI patterns while maintaining consistency and performance.
Client-Side Rendering Engine
The client-side rendering engine is responsible for translating server specifications into native UI elements:
- Component Mapping: Translate abstract component types to platform-specific implementations
- Layout Processing: Apply layout rules consistent with platform guidelines
- Property Application: Apply styling and behavioral properties
- Action Binding: Connect UI elements to appropriate action handlers
- Caching & Optimization: Efficiently manage component instantiation and updates
Rendering engines typically leverage existing UI frameworks (React Native, SwiftUI, Jetpack Compose, etc.) while providing the abstraction layer needed for SDUI.
Handling User Interactions
SDUI systems need well-defined patterns for handling user interactions:
- Action Types: Define standard interaction types (tap, swipe, form submit, etc.)
- Local vs. Remote Processing: Determine which interactions are handled locally vs. sent to server
- Feedback Mechanisms: Provide immediate visual feedback before server responses
- Error Handling: Manage failures in action processing
- State Updates: Update local UI based on interaction outcomes
Successful interaction models balance responsiveness (local processing) with consistency (server control).
Caching Strategies
Effective caching is critical for SDUI performance:
- UI Template Caching: Store frequently used UI templates locally
- Time-Based Expiration: Balance freshness with performance
- Conditional Fetching: Only download changed specifications
- Offline Support: Pre-cache essential UI specifications for offline use
- Partial Updates: Support updating only changed portions of the UI
Sophisticated SDUI implementations often include versioned template systems that allow incremental updates and efficient diff-based synchronization.
Case Studies: Server Driven UI in Action
Zalando's Appcraft
Zalando, one of Europe's largest e-commerce platforms, developed their "Appcraft" SDUI system to address what they call the "TNA dilemma" (Time, Number of developers, and Ambition). With teams working across multiple platforms, they faced challenges maintaining feature parity and consistent experiences.
Key aspects of Zalando's approach:
- ELM Architecture: They adopted the Elm Architecture pattern, separating their UI into Model, Update, and View components.
- Flex Layout: They implemented a cross-platform Flexbox-based layout system using Texture (iOS) and Litho (Android).
- Deep Linking: Screens are created dynamically via deep links, making navigation highly flexible.
- Simplified Versioning: Their approach reduced version compatibility issues between backend and frontend.
Business impact for Zalando included:
- Same-day delivery of feature changes
- Dynamic content adaptation based on user context
- Simplified app theming and branding updates
- Improved resilience through fallback mechanisms
- Enhanced experimentation capabilities
Yelp's CHAOS
Yelp developed their CHAOS (Component Hierarchy As an Output Specification) framework to streamline development across their various platforms. Before CHAOS, Yelp's teams were duplicating effort across iOS, Android, and web platforms.
Key aspects of Yelp's approach:
- GraphQL Integration: CHAOS leverages GraphQL to deliver both data and UI specifications in a single query.
- Hierarchical Structure: UI is organized into Views, Layouts, and Components.
- Action Framework: A standardized system for handling user interactions.
- Dream Query: Yelp's concept for obtaining exactly the UI structure and data needed in a single request.
Yelp's implementation enabled:
- Unified A/B testing across platforms
- Personalized UI based on user preferences (e.g., emphasizing photos for some users, reviews for others)
- Rapid iteration on search result presentation
- Consistent feature launches across platforms
Comparing Approaches
While both Zalando and Yelp built successful SDUI systems, their approaches differ in several ways:
- Architecture Pattern: Zalando adopted Elm Architecture, while Yelp built around GraphQL.
- Component Granularity: Zalando focused on screen-level composition, while Yelp emphasized fine-grained components.
- Data Integration: Yelp tightly coupled data and UI through GraphQL, while Zalando separated these concerns.
- Platform Focus: Zalando emphasized native performance, while Yelp prioritized cross-platform consistency.
These differences highlight that there's no one-size-fits-all approach to SDUI—successful implementations align with specific business needs and technical contexts.
Future Trends and Considerations
Integration with AI/ML
As artificial intelligence and machine learning advance, SDUI systems are increasingly incorporating these technologies:
- Adaptive Layouts: ML algorithms determine optimal layouts based on user behavior data
- Content Prioritization: AI-driven decisions about which content elements receive prominence
- Predictive UI: Anticipating user needs and preemptively adjusting the interface
- Personalization at Scale: Moving beyond segments to truly individualized experiences
Yelp, for example, already uses ML to personalize search results presentation, showing more visual content to users who engage with photos and more text to those who read reviews.
Serverless SDUI
The serverless computing paradigm aligns well with SDUI needs:
- On-Demand UI Generation: Create UI specifications only when needed
- Edge Computing: Generate UI specifications closer to users for lower latency
- Event-Driven Updates: Trigger UI changes based on backend events
- Cost Efficiency: Pay only for actual UI generation usage
As serverless platforms mature, we can expect more SDUI implementations to leverage these capabilities for improved scalability and performance.
Emerging UI Frameworks
Modern UI frameworks are increasingly supporting patterns that align with SDUI concepts:
- Declarative UI: Frameworks like SwiftUI, Jetpack Compose, and React make declarative UI the norm
- Component Models: Standardized component systems facilitate mapping between server specifications and rendered elements
- State Management: Sophisticated state management solutions address the challenges of synchronizing UI state
- Design Systems: Formalized design systems provide the vocabulary necessary for consistent SDUI implementations
These frameworks reduce the implementation complexity of SDUI systems while improving rendering performance and development experience.
Key Takeaways
Server Driven UI represents a paradigm shift in how we approach cross-platform application development. Key points to remember include:
- Centralized Control: SDUI centralizes UI decisions on the server, enabling consistent experiences across platforms
- Agility Benefits: The ability to update UI without app releases dramatically accelerates iteration cycles
- Balance Required: Successful implementations balance server control with client-side performance and user experience
- Architectural Investment: SDUI requires significant upfront investment in architecture, tooling, and processes
- Expanding Adoption: Major platforms like Zalando, Yelp, Airbnb, and others validate the approach for large-scale applications
Organizations considering SDUI should evaluate whether their specific context—multiple platforms, frequent UI changes, personalization needs—justifies the architectural investment.
Conclusion
Server Driven UI represents a powerful approach for organizations striving to deliver consistent, flexible user experiences across multiple platforms. By shifting UI control to the server, teams can accelerate development cycles, ensure platform consistency, and enable personalization at scale.
While the approach introduces challenges in performance, complexity, and state management, companies like Zalando and Yelp demonstrate that these challenges can be overcome with thoughtful architecture and implementation. As UI frameworks and tooling continue to evolve, SDUI adoption will likely become even more accessible to development teams of all sizes.
Whether you're managing a large-scale application across multiple platforms or simply seeking more flexibility in your UI development process, Server Driven UI offers a compelling architectural pattern worth exploring.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ