Real-Time Messaging Protocol (RTMP) has been a cornerstone of live streaming and video-on-demand content delivery for over two decades. Historically, RTMP relied heavily on Adobe Flash Player for playback, creating a seamless solution for streaming media across the web. However, with Flash officially discontinued in December 2020 and blocked by most browsers due to security vulnerabilities, many content creators and developers face a significant challenge: how to leverage RTMP's benefits without its traditional playback environment.
The good news is that modern web technologies have evolved to fill this gap, offering robust alternatives for playing and embedding RTMP streams without Flash. This comprehensive guide explores practical approaches to RTMP streaming in today's Flash-free landscape, with a focus on HTML5 players, JavaScript libraries, and server-side transcoding solutions that keep your content flowing smoothly to viewers on any device.
What is RTMP Streaming?
Real-Time Messaging Protocol (RTMP) is a TCP-based protocol designed for high-performance transmission of audio, video, and data between a server and a client. Originally developed by Macromedia (later acquired by Adobe), RTMP became widely adopted for its low-latency capabilities and reliability in streaming media content.
At its core, RTMP establishes a persistent connection between client and server, chunking data and multiplexing it over a single connection. This approach enables efficient delivery of media streams with minimal delay, making it particularly valuable for live streaming scenarios where real-time interaction matters.
Several variations of RTMP exist, each serving specific purposes:
- RTMP: The standard protocol operating over TCP port 1935
- RTMPS: RTMP over a TLS/SSL connection, adding encryption for security
- RTMPE: RTMP with encryption using Adobe's security mechanism
- RTMPT: RTMP tunneled over HTTP, useful for bypassing firewalls
- RTMFP: Real-Time Media Flow Protocol, a UDP-based alternative
- E-RTMP: Enhanced RTMP, allowing multiple streams in a single connection
Despite these variations, all RTMP formats share the same fundamental approach to media streaming, providing consistent performance and reliability for content delivery.
Why Move Away from Flash for RTMP?
Adobe Flash Player served as the primary vehicle for RTMP playback for many years, but its deprecation has forced a technological shift in the streaming landscape. Understanding why this shift was necessary helps explain the importance of finding alternative playback solutions:
Flash's Decline and End-of-Life
Flash's decline began around 2010 when Apple decided not to support it on iOS devices. Over the following decade, security vulnerabilities, performance issues, and the rise of HTML5 alternatives steadily eroded Flash's dominance. Adobe officially ended support for Flash on December 31, 2020, and major browsers have since blocked Flash content entirely.
Security and Performance Concerns
Throughout its lifetime, Flash was plagued by security vulnerabilities that made it a prime target for exploits. Its proprietary nature, resource-intensive operation, and impact on battery life further contributed to its downfall, particularly as mobile browsing became increasingly prevalent.
Advantages of Modern Alternatives
Moving away from Flash-based RTMP playback offers several significant benefits:
- Enhanced security: Reduced vulnerability to common Flash-based exploits
- Improved performance: Lower CPU and memory usage, resulting in better battery life for mobile devices
- Cross-browser compatibility: Consistent functionality across all modern browsers
- Mobile support: Full compatibility with iOS, Android, and other mobile platforms
- Future-proofing: Reliance on actively maintained technologies rather than deprecated ones
With Flash no longer viable, developers must adopt alternative approaches to ensure their RTMP streams remain accessible to viewers.
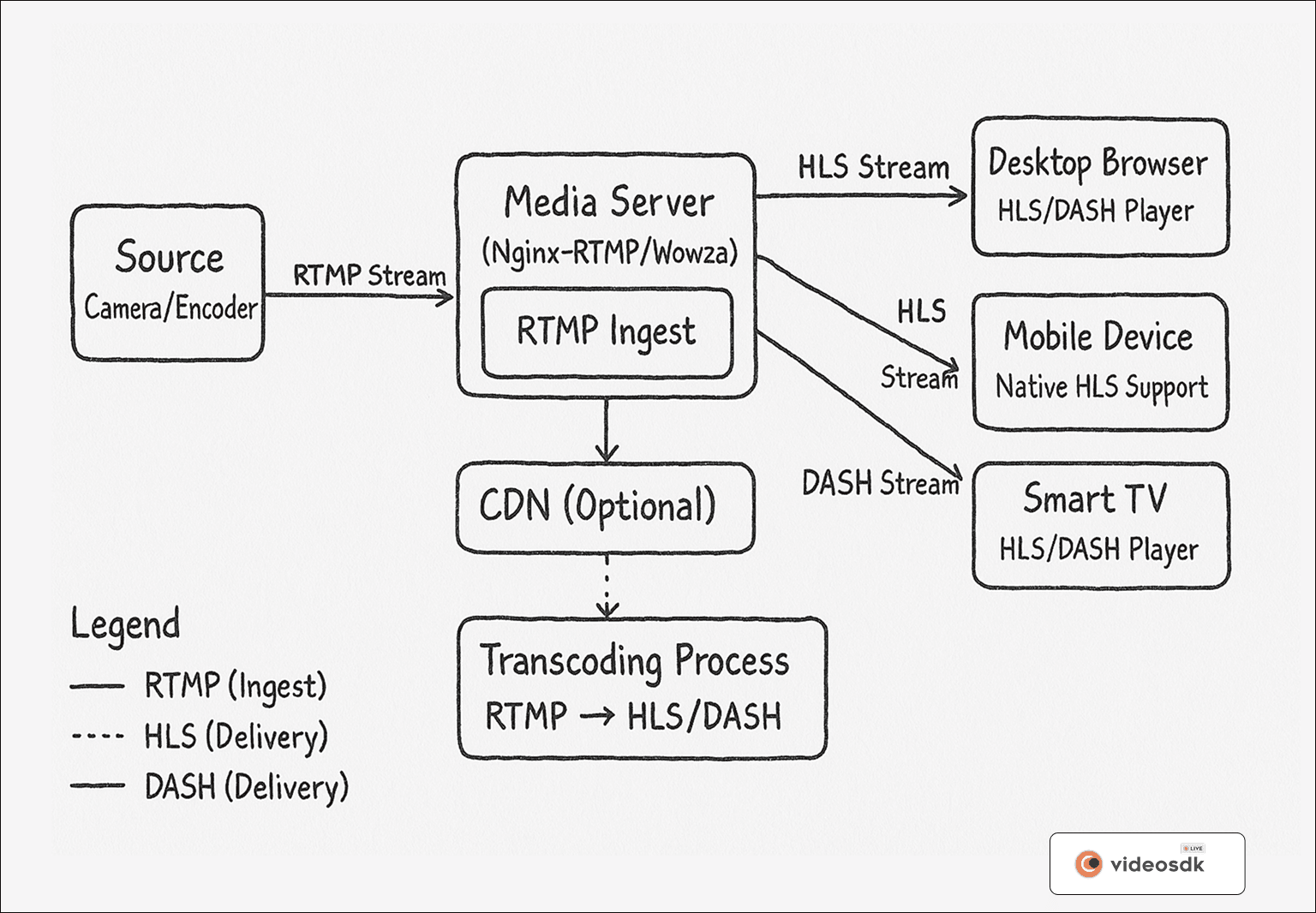
Figure 1: Modern RTMP Streaming Architecture - This diagram illustrates the complete workflow of RTMP streaming in today's Flash-free environment. The source device sends an RTMP stream to a media server, which transcodes it to web-friendly formats like HLS and DASH. These formats can then be delivered to various devices and browsers through optional CDN distribution, ensuring wide compatibility and optimal performance across different platforms.
Playing RTMP Streams Without Flash: Modern Approaches
Several modern technologies and methodologies have emerged to fill the void left by Flash's departure. These approaches offer varying levels of complexity, flexibility, and compatibility, allowing developers to choose solutions that best fit their specific requirements.
HTML5 Video Players with RTMP Support
While HTML5's native
<video>
element doesn't directly support RTMP streams, several third-party players have developed workarounds and extensions to bridge this gap:Video.js with RTMP Plugin
Video.js
is one of the most popular HTML5 video players, and with the appropriate plugin, it can handle RTMP streams through a combination of technologies:1<link href="https://vjs.zencdn.net/7.20.3/video-js.css" rel="stylesheet">
2<script src="https://vjs.zencdn.net/7.20.3/video.min.js"></script>
3<script src="https://unpkg.com/videojs-flash/dist/videojs-flash.js"></script>
4<script src="https://unpkg.com/videojs-contrib-hls/dist/videojs-contrib-hls.js"></script>
5
6<video id="my-video" class="video-js" controls preload="auto" width="640" height="360">
7 <source src="rtmp://streaming-server.com/live/stream-key" type="rtmp/mp4">
8 <p class="vjs-no-js">
9 To view this video please enable JavaScript, and consider upgrading to a
10 web browser that supports HTML5 video
11 </p>
12</video>
13
14<script>
15 var player = videojs('my-video');
16 player.play();
17</script>
18
It's important to note that this approach still partially relies on Flash emulation under the hood for some browsers, which means it may not work consistently across all platforms.
JW Player
JW Player
offers RTMP support through its enterprise-level plans, providing a solution that transcodes RTMP to HLS on the fly for broad compatibility:1<script src="https://cdn.jwplayer.com/libraries/ABCDEFGH.js"></script>
2
3<div id="my-player"></div>
4
5<script>
6 jwplayer("my-player").setup({
7 file: "rtmp://streaming-server.com/live/stream-key",
8 width: "100%",
9 aspectratio: "16:9"
10 });
11</script>
12
JW Player handles the complexity of RTMP playback internally by using RTMP for ingest, then converting to formats like HLS that are natively supported in HTML5 environments.
JavaScript Libraries for RTMP Handling
Several JavaScript libraries have been developed specifically to handle RTMP streams in modern browsers without requiring Flash:
flv.js
flv.js
is an open-source JavaScript library that works by transmoding FLV streams (which can be delivered via HTTP) into a format compatible with the HTML5 Media Source Extensions (MSE). While not directly working with RTMP, it can be used in conjunction with a server that converts RTMP to HTTP-FLV:1<script src="https://cdn.jsdelivr.net/npm/flv.js@1.6.2/dist/flv.min.js"></script>
2
3<video id="player" controls></video>
4
5<script>
6 if (flvjs.isSupported()) {
7 var videoElement = document.getElementById('player');
8 var flvPlayer = flvjs.createPlayer({
9 type: 'flv',
10 url: 'http://example.com/live/livestream.flv'
11 });
12 flvPlayer.attachMediaElement(videoElement);
13 flvPlayer.load();
14 flvPlayer.play();
15 }
16</script>
17
hls.js
hls.js
is another JavaScript library that enables HLS playback in browsers that don't natively support it. When combined with server-side RTMP-to-HLS conversion, it provides a robust solution for RTMP content delivery:1<script src="https://cdn.jsdelivr.net/npm/hls.js@1.1.5/dist/hls.min.js"></script>
2
3<video id="video" controls></video>
4
5<script>
6 var video = document.getElementById('video');
7 if (Hls.isSupported()) {
8 var hls = new Hls();
9 hls.loadSource('https://example.com/playlist.m3u8'); // HLS stream converted from RTMP
10 hls.attachMedia(video);
11 hls.on(Hls.Events.MANIFEST_PARSED, function() {
12 video.play();
13 });
14 }
15 else if (video.canPlayType('application/vnd.apple.mpegurl')) {
16 // Native HLS support (Safari)
17 video.src = 'https://example.com/playlist.m3u8';
18 video.addEventListener('loadedmetadata', function() {
19 video.play();
20 });
21 }
22</script>
23
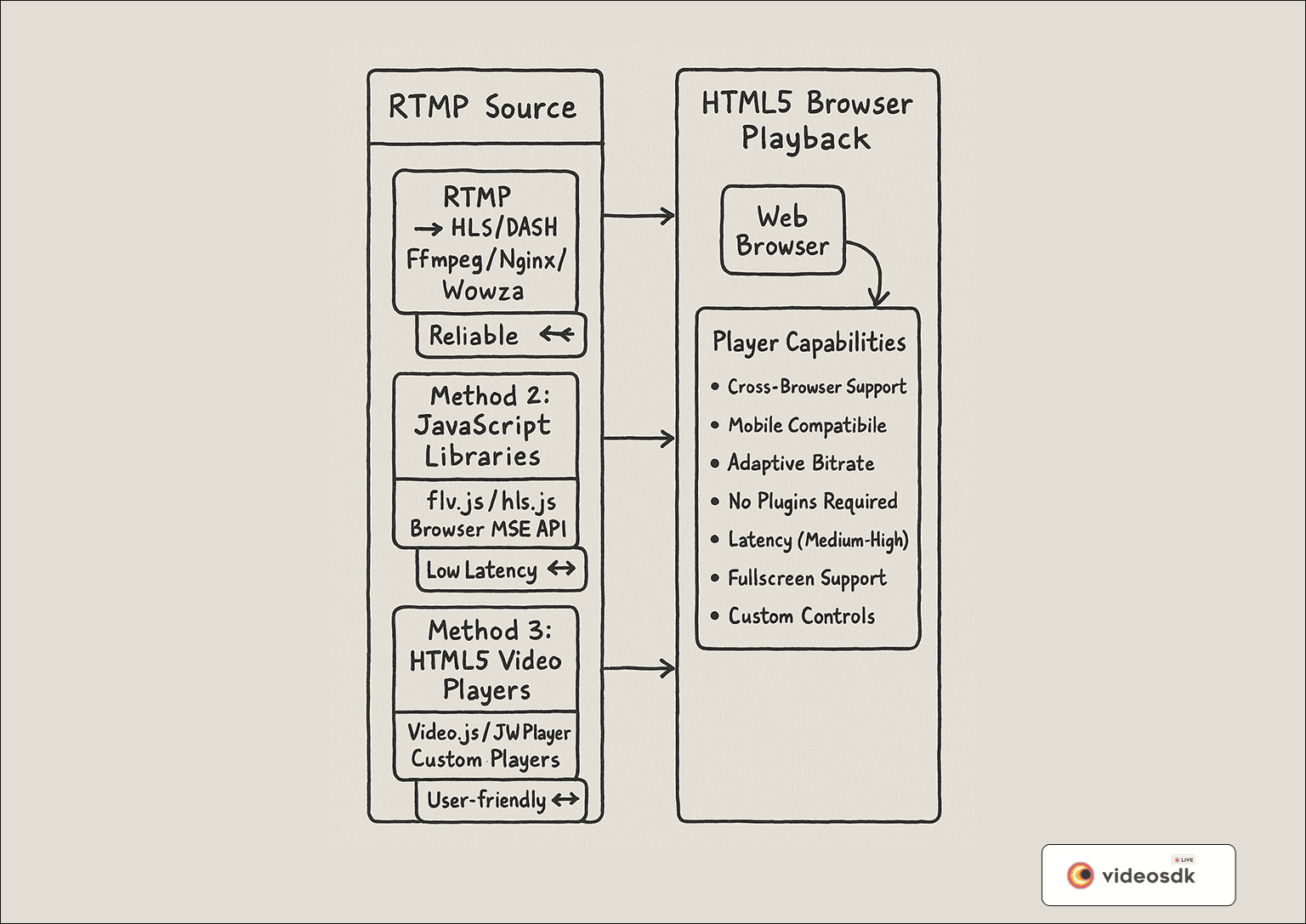
Figure 2: RTMP to HTML5 Implementation Methods - This diagram compares the three main approaches for implementing RTMP playback in HTML5 environments: server-side transcoding (converting RTMP to HLS/DASH), JavaScript libraries (like flv.js and hls.js), and HTML5 video players with RTMP support. Each method has its advantages and disadvantages, with considerations for compatibility, complexity, and performance. The diagram also illustrates how these methods connect to HTML5 browsers and the resulting player capabilities.
Server-Side Transcoding: RTMP to HLS/DASH
Perhaps the most versatile and reliable approach to handling RTMP in a Flash-free world is server-side transcoding. This method involves:
- Receiving the stream via RTMP (which remains excellent for ingest)
- Transcoding it on the server to a more widely supported format like HLS or DASH
- Delivering the transcoded stream to viewers using formats natively supported by HTML5
This approach leverages RTMP's strengths for ingestion while avoiding its limitations for playback:
Using FFmpeg for Transcoding
FFmpeg can be used to transcode RTMP to HLS on the server:
1ffmpeg -i rtmp://source-server/live/stream-key \
2 -c:v libx264 -c:a aac -b:v 1000k -b:a 128k \
3 -f hls -hls_time 4 -hls_playlist_type event \
4 /var/www/html/stream/playlist.m3u8
5
Using Nginx with RTMP Module
Nginx can be configured with the RTMP module to automatically transcode incoming RTMP streams to HLS:
1rtmp {
2 server {
3 listen 1935;
4
5 application live {
6 live on;
7 record off;
8
9 # Convert RTMP to HLS
10 hls on;
11 hls_path /var/www/html/hls;
12 hls_fragment 3;
13 hls_playlist_length 60;
14 }
15 }
16}
17
18http {
19 server {
20 listen 80;
21
22 location /hls {
23 # Serve HLS fragments
24 types {
25 application/vnd.apple.mpegurl m3u8;
26 video/mp2t ts;
27 }
28 root /var/www/html;
29 add_header Cache-Control no-cache;
30 }
31 }
32}
33
WebAssembly-Based Players
WebAssembly (WASM) represents a more advanced and emerging approach to handling complex media tasks in the browser. Several projects are exploring WASM-based solutions for playing various formats, including content derived from RTMP streams:
mpv.js and mpv-player
These libraries utilize WebAssembly to bring the powerful mpv player to the browser, potentially enabling playback of various formats including RTMP-derived content.
Embedding RTMP Streams in Your Website (No Flash)
Now that we've explored the technologies available for Flash-free RTMP playback, let's look at practical implementation steps for embedding these streams in your website.
Implementation Using Video.js
First, include the necessary scripts and styles in your HTML:
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>RTMP Stream Player</title>
7
8 <!-- Video.js CSS -->
9 <link href="https://vjs.zencdn.net/7.20.3/video-js.css" rel="stylesheet">
10</head>
11<body>
12 <div class="container">
13 <h1>Live Stream</h1>
14
15 <!-- Video.js Player -->
16 <video-js id="my-player"
17 class="video-js vjs-big-play-centered vjs-fluid"
18 controls
19 preload="auto"
20 data-setup='{}'>
21 </video-js>
22 </div>
23
24 <!-- Video.js Core -->
25 <script src="https://vjs.zencdn.net/7.20.3/video.min.js"></script>
26
27 <!-- HLS Support for browsers that don't support it natively -->
28 <script src="https://cdn.jsdelivr.net/npm/videojs-contrib-hls@5.15.0/dist/videojs-contrib-hls.min.js"></script>
29
30 <script>
31 // Initialize player with HLS stream (converted from RTMP)
32 var player = videojs('my-player');
33
34 // Load the HLS manifest
35 player.src({
36 src: 'https://yourdomain.com/live/stream/playlist.m3u8',
37 type: 'application/x-mpegURL'
38 });
39
40 player.play();
41 </script>
42</body>
43</html>
44
This implementation assumes you're using server-side transcoding to convert your RTMP stream to HLS.
Implementation Using flv.js for HTTP-FLV
If you've configured your server to provide HTTP-FLV streams converted from RTMP:
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>RTMP Stream Player (via HTTP-FLV)</title>
7 <style>
8 .player-container {
9 max-width: 800px;
10 margin: 0 auto;
11 }
12 video {
13 width: 100%;
14 }
15 </style>
16</head>
17<body>
18 <div class="player-container">
19 <h1>Live Stream</h1>
20 <video id="player" controls></video>
21 </div>
22
23 <script src="https://cdn.jsdelivr.net/npm/flv.js@1.6.2/dist/flv.min.js"></script>
24 <script>
25 document.addEventListener('DOMContentLoaded', function() {
26 if (flvjs.isSupported()) {
27 var videoElement = document.getElementById('player');
28 var flvPlayer = flvjs.createPlayer({
29 type: 'flv',
30 url: 'http://yourdomain.com/live/livestream.flv',
31 isLive: true
32 });
33
34 flvPlayer.attachMediaElement(videoElement);
35 flvPlayer.load();
36
37 // Auto-play if desired
38 videoElement.addEventListener('loadedmetadata', function() {
39 videoElement.play();
40 });
41
42 // Cleanup on page unload
43 window.addEventListener('beforeunload', function() {
44 flvPlayer.unload();
45 flvPlayer.detachMediaElement();
46 flvPlayer.destroy();
47 });
48 } else {
49 console.error('Your browser does not support flv.js');
50 document.getElementById('player').innerHTML = 'Your browser does not support the required technology. Please try a more recent version of Chrome, Firefox, or Edge.';
51 }
52 });
53 </script>
54</body>
55</html>
56
Considerations for Different Browsers and Devices
When implementing RTMP playback without Flash, it's essential to account for varying levels of support across browsers and devices:
- iOS Compatibility: iOS only supports HLS natively, making server-side RTMP-to-HLS transcoding essential for Apple mobile devices.
- Adaptive Bitrate Streaming: Consider generating multiple quality levels in your HLS or DASH streams to accommodate varying network conditions.
- Fallback Mechanisms: Implement detection and fallback logic to provide the best possible experience for each user:
1function setupPlayer() {
2 // Try HLS.js first (for browsers that don't support HLS natively)
3 if (Hls.isSupported()) {
4 setupHlsPlayer();
5 }
6 // Try native HLS support (Safari)
7 else if (video.canPlayType('application/vnd.apple.mpegurl')) {
8 setupNativeHlsPlayer();
9 }
10 // Try flv.js for HTTP-FLV
11 else if (flvjs && flvjs.isSupported()) {
12 setupFlvPlayer();
13 }
14 // Final fallback - perhaps a static image or message
15 else {
16 showFallbackContent();
17 }
18}
19
- Testing: Thoroughly test your implementation across multiple browsers, devices, and network conditions before deploying to production.
Setting Up Your RTMP Server (Brief Overview)
While this article focuses primarily on playing and embedding RTMP streams without Flash, setting up a proper RTMP server is equally important for the complete streaming pipeline. Here's a brief overview of popular options:
Nginx RTMP Module
Nginx with the RTMP module provides a powerful, open-source solution for RTMP streaming:
- Installation (Ubuntu/Debian): ```bash sudo apt-get update sudo apt-get install build-essential libpcre3-dev libssl-dev
Clone Nginx RTMP module
Download and compile Nginx with RTMP module
wgethttp://nginx.org/download/nginx-1.21.6.tar.gz
tar -xf nginx-1.21.6.tar.gz cd nginx-1.21.6./configure --with-http_ssl_module --add-module=../nginx-rtmp-module make sudo make install ``` - Basic Configuration: ```nginx
nginx.conf
rtmp { server { listen 1935; chunk_size 4096;1 application live { 2 live on; 3 record off; 4 5 # HLS 6 hls on; 7 hls_path /var/www/html/hls; 8 hls_fragment 3; 9 hls_playlist_length 60; 10 11 # Optional: DASH 12 dash on; 13 dash_path /var/www/html/dash; 14 } 15}
}http { server { listen 80;1 location /hls { 2 # Serve HLS fragments 3 types { 4 application/vnd.apple.mpegurl m3u8; 5 video/mp2t ts; 6 } 7 root /var/www/html; 8 add_header Cache-Control no-cache; 9 add_header Access-Control-Allow-Origin *; 10 } 11 12 location /dash { 13 root /var/www/html; 14 add_header Cache-Control no-cache; 15 add_header Access-Control-Allow-Origin *; 16 } 17}
} ```
Other RTMP Server Options
- Wowza Streaming Engine: A commercial solution offering comprehensive streaming capabilities, including RTMP ingest with automatic transcoding to various formats.
- Red5: An open-source media server for RTMP streaming, written in Java.
- Node-Media-Server: A Node.js implementation of an RTMP/HTTP-FLV/HLS/DASH media server.
Security Considerations
When deploying an RTMP server, consider these security measures:
- Stream Keys: Implement secure stream keys to prevent unauthorized broadcasting.
- IP Restrictions: Limit RTMP publishing access to trusted IP addresses.
- RTMPS: Use RTMPS (RTMP over SSL) to encrypt the connection between the broadcaster and server.
- Regular Updates: Keep your server software updated to address security vulnerabilities.
Troubleshooting Common RTMP Streaming Issues
Even with modern implementations, RTMP streaming can encounter various issues. Here are some common problems and their solutions:
Connection Problems
Symptoms: Stream fails to connect, or disconnects frequently.
Solutions:
- Verify that the RTMP URL is correct, including the application name and stream key
- Check if port 1935 (default for RTMP) is open in all firewalls between client and server
- Ensure your server has sufficient bandwidth and resources
- For persistent issues, try RTMPT (RTMP Tunneled) which uses HTTP port 80 or 443
Buffering and Latency Issues
Symptoms: Stream playback stutters, buffers frequently, or has high latency.
Solutions:
- Reduce the input bitrate at the encoder
- Adjust server buffer settings
- For HLS, consider reducing segment duration (balancing quality vs. latency)
- Ensure your server has adequate CPU resources for transcoding
- Test with different CDNs if applicable
Codec Compatibility Problems
Symptoms: Stream connects but shows no video or audio.
Solutions:
- Ensure you're using widely supported codecs (H.264 for video, AAC for audio)
- Check that your player supports the specific codec profile used
- Verify that transcoding settings match the capabilities of target devices
- Add multiple renditions with different codec parameters for broader compatibility
Browser and Device-Specific Issues
Symptoms: Stream works on some devices but not others.
Solutions:
- For iOS, ensure you're providing an HLS stream
- For older Android devices, provide lower resolution options
- Use adaptive bitrate streaming to accommodate varying device capabilities
- Implement device detection and serve appropriate stream formats accordingly
Key Takeaways
As we've explored throughout this article, RTMP streaming remains viable in a post-Flash world, though its implementation requires modern approaches:
- Server-side transcoding offers the most universal solution, converting RTMP ingest to formats like HLS and DASH that are widely supported across browsers and devices.
- JavaScript libraries such as hls.js and flv.js bridge compatibility gaps, ensuring consistent playback across different environments.
- HTML5 video players with appropriate plugins provide user-friendly interfaces for RTMP-derived content.
- WebAssembly-based solutions represent the cutting edge of in-browser media handling, with promising developments on the horizon.
The key to success lies in selecting the right combination of technologies based on your specific requirements, audience devices, and technical infrastructure.
Conclusion
The deprecation of Flash created significant challenges for RTMP streaming, but modern web technologies have risen to meet these challenges. By leveraging server-side transcoding, HTML5 video players, and specialized JavaScript libraries, developers can continue to benefit from RTMP's strengths while delivering content in formats that work seamlessly across today's diverse ecosystem of browsers and devices.
As streaming technology continues to evolve, we're likely to see further innovations in low-latency delivery and simplified implementations. WebRTC, for instance, offers sub-second latency for specific use cases, while ongoing improvements to HLS and DASH continue to enhance their capabilities.
The future of streaming is bright, built on a foundation of open standards and broad compatibility rather than proprietary plugins. By adopting the approaches outlined in this article, you can ensure your RTMP streams remain accessible and effective in this new streaming landscape.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ