Introduction to Licode WebRTC
In the rapidly evolving landscape of real-time communication, Licode WebRTC stands out as a robust, open-source platform designed to facilitate seamless video conferencing, streaming, and recording. Licode is built using a combination of C++ and Node.js, leveraging the power of both languages to deliver high-performance media communications. It employs a Multipoint Control Unit (MCU) architecture, which centralizes the processing of video and audio streams, making it an ideal solution for scalable and efficient real-time communication applications.
What is Licode WebRTC?
Licode WebRTC simplifies the development of WebRTC-based applications by providing a comprehensive set of tools and functionalities. This platform enables developers to create sophisticated real-time communication applications with minimal effort, handling the complexities of media transmission and reception under the hood. Whether you're building a video conferencing app, a live streaming service, or a recording solution, Licode offers the flexibility and power needed to meet your project's demands.
By using Licode, developers can focus on enhancing user experience and functionality rather than dealing with the intricate details of WebRTC implementation. Its open-source nature encourages community contributions, ensuring continuous improvement and adaptation to emerging technological trends. This introductory guide will walk you through the essential steps to get started with Licode, offering practical examples and insights to help you harness the full potential of this powerful platform.
Getting Started with the Code
Create a New Licode App
To kickstart your journey with Licode WebRTC, you need to set up a new application by cloning the Licode repository from GitHub. This will give you access to all the source code and necessary tools to build and customize your real-time communication application.
[a] Clone the Repository
bash
1 git clone https://github.com/lynckia/licode.git
2 cd licode
[b] Install Dependencies
Before running the application, you need to install all required dependencies. This includes Node.js and other libraries.
bash
1 ./scripts/installUbuntuDeps.sh
Install
Installing Licode involves a few steps to ensure all components are correctly set up and configured.
[a] Install Node.js
Ensure you have Node.js installed. If not, download and install the latest version from the
Node.js official website
.[b] Configure Environment Variables
Set up your environment variables to ensure Licode can run properly. Create a
.env
file or export variables directly in your terminal.bash
1 export ERIZO_HOME=$(pwd)
2 export PATH=$PATH:$(pwd)/build/scripts
[c] Install Licode
Run the installation script provided in the Licode repository.
bash
1 ./scripts/installErizo.sh
Structure of the Project
Understanding the structure of your Licode project is crucial for efficient development. The main folders and files include:
erizo/
: Contains the core media server components written in C++.licode/
: Includes Node.js server code and modules.scripts/
: Contains installation and configuration scripts.www/
: The directory where you can place your frontend application files.
Each of these directories plays a specific role in the overall architecture, ensuring that media processing, signaling, and application logic are properly separated and managed.
App Architecture
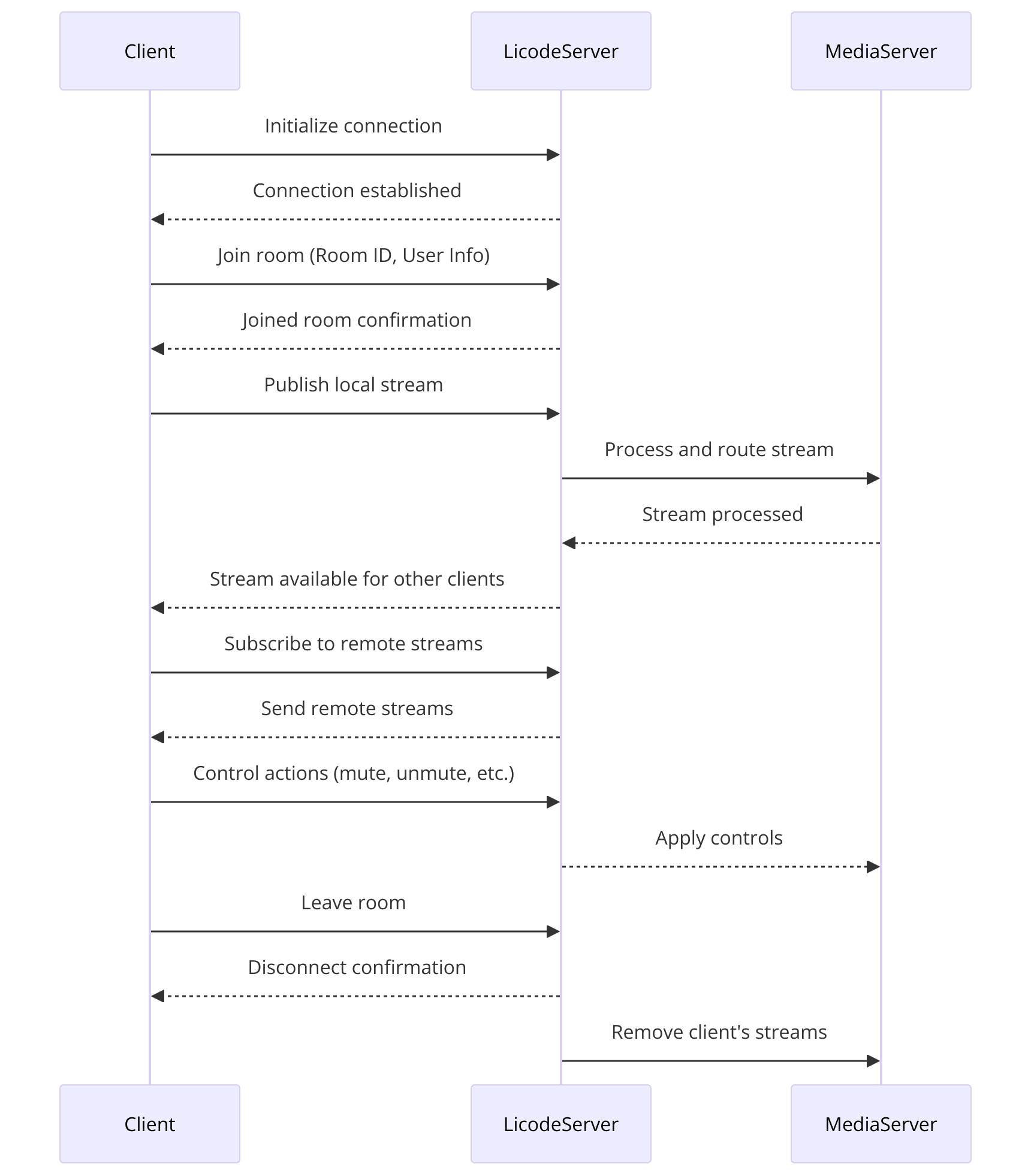
- MCU (Multipoint Control Unit): Centralizes the processing of video and audio streams, optimizing bandwidth usage and enabling efficient media routing.
- ErizoController: Manages signaling and communication between clients and the media server.
- ErizoClient: A JavaScript library for frontend integration, facilitating communication with the ErizoController and managing media streams on the client side.
Building Licode
With the repository cloned and dependencies installed, the next step is to build Licode.
[a] Compile Licode
bash
1 ./scripts/installErizo.sh
[b] Start the Services
Use the provided scripts to start all necessary services, including ErizoController and the Nuve API server.
bash
1 ./scripts/initLicode.sh
Initial Configuration
Before you can start developing your application, some initial configuration is required:
[a] Edit licode_config.js
Customize the configuration file to suit your environment and application needs. This file contains various settings related to network, ports, and media handling.
[b] Run the Application
After configuration, you can run the Licode server and test its functionality.
bash
1 ./scripts/initBasicExample.sh
By following these steps, you will have a functional Licode setup ready for development. In the next sections, we will dive into implementing specific features and building a comprehensive real-time communication application using Licode WebRTC.
Step 1: Get Started with Licode
To effectively use Licode WebRTC, the first step is to set up your development environment and ensure that everything is properly configured for smooth operation. This section will guide you through the essential steps to get started with Licode.
Setting Up the Development Environment
Before you begin building with Licode, make sure your development environment is equipped with the necessary tools and software versions.
[a] Required Tools and Versions
- Node.js: Ensure you have Node.js installed. The recommended version is the latest LTS version.
- Git: Version control system to clone the Licode repository.
- Build Tools: Ensure you have build-essential, cmake, and other necessary tools installed.
To install these tools on Ubuntu, you can use:
bash
1 sudo apt-get update
2 sudo apt-get install -y build-essential cmake git
[b] Initial Configuration
Once you have the required tools, proceed with the initial configuration of Licode.
bash
1 git clone https://github.com/lynckia/licode.git
2 cd licode
3 ./scripts/installUbuntuDeps.sh
[c] Environment Variables
Set up environment variables to ensure that the paths are correctly configured.
bash
1 export ERIZO_HOME=$(pwd)
2 export PATH=$PATH:$(pwd)/build/scripts
Building Licode
After setting up the development environment, the next step is to build Licode. This involves compiling the core components and preparing the server for operation.
[a] Detailed Build Instructions
Run the installation script to build Licode. This script compiles the media server components and sets up the necessary configurations.
bash
1 ./scripts/installErizo.sh
This process may take some time, as it compiles several C++ modules and installs required Node.js packages.
[b] Troubleshooting Common Build Issues
During the build process, you might encounter some issues. Here are a few common problems and their solutions:
- Missing Dependencies: Ensure all dependencies are installed. Refer to the installation script output for any missing packages.
- Compilation Errors: Verify that you have the correct versions of compilers and tools. Sometimes, updating your package list and upgrading installed packages can resolve these errors.
bash
1 sudo apt-get update
2 sudo apt-get upgrade
Running Licode Services
With Licode built successfully, you need to start the core services to get the server up and running.
[a] Starting ErizoController and Nuve API Server
Use the initialization script to start the necessary services.
bash
1 ./scripts/initLicode.sh
[b] Verifying Services
After running the script, verify that all services are running correctly. You should see log messages indicating that ErizoController, Nuve, and the basic example server have started successfully.
bash
1 tail -f erizo_controller/erizoController.log
Initial Configuration
Before you start developing your application, some initial configurations are required to customize Licode to your needs.
[a] Editing licode_config.js
The
licode_config.js
file contains various settings related to network configuration, ports, and media handling. Customize this file according to your deployment environment and requirements.JavaScript
1 var config = {
2 cloudProvider: 'amazon',
3 network: {
4 'publicIP': 'YOUR_PUBLIC_IP',
5 'privateIP': 'YOUR_PRIVATE_IP',
6 'port': 443
7 },
8 ...
9 };
10 module.exports = config;
[b] Running the Basic Example
Licode provides a basic example to test your setup. Run the example to ensure everything is working as expected.
bash
1 ./scripts/initBasicExample.sh
Open a web browser and navigate to
http://localhost:3001
. You should see the basic example application, which allows you to test video and audio streaming functionalities.By completing these steps, you will have a fully functional Licode setup ready for further development. The next sections will guide you through implementing specific features and building a comprehensive real-time communication application using Licode WebRTC.
Step 2 - Wireframe All the Components
In this section, we will design and integrate the user interface components of your Licode WebRTC application. Wireframing helps visualize the layout and functionality of the application before diving into the actual code. Here, we will outline the process of creating wireframes and integrating the UI components into the application.
Designing the Application Layout
To begin, let's design the layout of our application. This layout will include the main components necessary for a typical video conferencing application, such as video streams, controls, and a participant list.
Creating Wireframes for the User Interface
- Video Streams Area: This section will display the video streams of participants. It will occupy the central part of the interface.
- Controls: The controls (e.g., mute, unmute, start video, stop video) will be placed at the bottom or top of the interface for easy access.
- Participant List: A sidebar will list the active participants in the room, providing an overview of who is currently connected.
Wireframe Example
- Central Video Area: 70% of the screen width, centered.
- Controls: Positioned at the bottom, spanning the width of the video area.
- Participant List: Occupies 30% of the screen width, aligned to the right.
Integrating UI Components
With the wireframe in place, the next step is to integrate these components into your application. We'll use HTML and CSS for the layout and JavaScript for interactivity.
[a] Setting Up the HTML Structure
Create an
index.html
file in the www
directory of your Licode project.HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>Licode WebRTC App</title>
7 <link rel="stylesheet" href="styles.css">
8 </head>
9 <body>
10 <div id="video-area">
11 <!-- Video streams will be injected here -->
12 </div>
13 <div id="controls">
14 <button id="mute-btn">Mute</button>
15 <button id="unmute-btn">Unmute</button>
16 <button id="start-video-btn">Start Video</button>
17 <button id="stop-video-btn">Stop Video</button>
18 </div>
19 <div id="participant-list">
20 <h3>Participants</h3>
21 <ul id="participants">
22 <!-- Participant names will be listed here -->
23 </ul>
24 </div>
25 <script src="app.js"></script>
26 </body>
27 </html>
[b] Styling the Components
Create a
styles.css
file to style the HTML elements.CSS
1 body {
2 font-family: Arial, sans-serif;
3 margin: 0;
4 display: flex;
5 flex-direction: column;
6 align-items: center;
7 }
8
9 #video-area {
10 width: 70%;
11 height: 70vh;
12 background-color: #000;
13 margin-top: 20px;
14 display: flex;
15 justify-content: center;
16 align-items: center;
17 }
18
19 #controls {
20 width: 70%;
21 display: flex;
22 justify-content: space-around;
23 margin: 20px 0;
24 }
25
26 #participant-list {
27 width: 30%;
28 background-color: #f0f0f0;
29 padding: 10px;
30 margin-top: 20px;
31 }
32
33 #participants {
34 list-style: none;
35 padding: 0;
36 }
37
38 #participants li {
39 margin: 5px 0;
40 }
[c] Implementing JavaScript for Interactivity
Create an
app.js
file to handle the logic for interacting with Licode and managing the UI components.JavaScript
1 document.getElementById('mute-btn').addEventListener('click', () => {
2 // Logic to mute the audio
3 console.log('Mute button clicked');
4 });
5
6 document.getElementById('unmute-btn').addEventListener('click', () => {
7 // Logic to unmute the audio
8 console.log('Unmute button clicked');
9 });
10
11 document.getElementById('start-video-btn').addEventListener('click', () => {
12 // Logic to start the video
13 console.log('Start Video button clicked');
14 });
15
16 document.getElementById('stop-video-btn').addEventListener('click', () => {
17 // Logic to stop the video
18 console.log('Stop Video button clicked');
19 });
20
21 // Function to add participants to the list
22 function addParticipant(name) {
23 const participantList = document.getElementById('participants');
24 const listItem = document.createElement('li');
25 listItem.textContent = name;
26 participantList.appendChild(listItem);
27 }
28
29 // Example usage
30 addParticipant('User 1');
31 addParticipant('User 2');
32 addParticipant('User 3');
By following these steps, you will have a basic but functional UI for your Licode WebRTC application. The next sections will delve deeper into implementing specific features such as the join screen, controls, and participant views.
Step 3: Implement Join Screen
In this step, we will create and implement the join screen for your Licode WebRTC application. The join screen allows users to enter the necessary information, such as their username and room ID, to join a video conference. This section will cover the design and implementation of the join screen, as well as handling user input and authentication.
Creating the Join Screen
[a] UI Design for the Join Screen
The join screen will have input fields for the room ID and username, along with a button to join the room. The layout will be simple and user-friendly.
HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>Join Licode Room</title>
7 <link rel="stylesheet" href="styles.css">
8 </head>
9 <body>
10 <div id="join-screen">
11 <h1>Join Room</h1>
12 <input type="text" id="room-id" placeholder="Enter Room ID">
13 <input type="text" id="username" placeholder="Enter Username">
14 <button id="join-btn">Join</button>
15 </div>
16 <script src="app.js"></script>
17 </body>
18 </html>
[b] Styling the Join Screen
Add styles to
styles.css
to enhance the appearance of the join screen.CSS
1 body {
2 font-family: Arial, sans-serif;
3 display: flex;
4 justify-content: center;
5 align-items: center;
6 height: 100vh;
7 margin: 0;
8 }
9
10 #join-screen {
11 text-align: center;
12 background-color: #f0f0f0;
13 padding: 20px;
14 border-radius: 10px;
15 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
16 }
17
18 #join-screen h1 {
19 margin-bottom: 20px;
20 }
21
22 #join-screen input {
23 display: block;
24 width: 80%;
25 margin: 10px auto;
26 padding: 10px;
27 border: 1px solid #ccc;
28 border-radius: 5px;
29 }
30
31 #join-screen button {
32 padding: 10px 20px;
33 background-color: #28a745;
34 color: #fff;
35 border: none;
36 border-radius: 5px;
37 cursor: pointer;
38 }
39
40 #join-screen button:hover {
41 background-color: #218838;
42 }
Handling User Authentication
Once the user inputs their room ID and username, you need to handle the authentication process and join them to the specified room.
[a] Implementing User Input Handling
In
app.js
, add the logic to handle user inputs and authenticate the user.JavaScript
1 document.getElementById('join-btn').addEventListener('click', () => {
2 const roomId = document.getElementById('room-id').value;
3 const username = document.getElementById('username').value;
4
5 if (roomId && username) {
6 joinRoom(roomId, username);
7 } else {
8 alert('Please enter both room ID and username');
9 }
10 });
11
12 function joinRoom(roomId, username) {
13 // Logic to join the room using Licode API
14 console.log(`Joining room ${roomId} as ${username}`);
15
16 // Example: Redirect to the main application page
17 window.location.href = `/room.html?roomId=${roomId}&username=${username}`;
18 }
[b] Connecting to the Room
The
joinRoom
function will handle the connection logic. You can use Licode's client library to establish the connection.JavaScript
1 function joinRoom(roomId, username) {
2 const options = {
3 // Define connection options such as room ID and user name
4 room: roomId,
5 user: username,
6 // Other necessary options
7 };
8
9 // Example: Initialize the Licode client and join the room
10 Licode.init(options)
11 .then(client => {
12 console.log('Connected to the room', client);
13 // Save client instance and navigate to the main application page
14 window.location.href = `/room.html?roomId=${roomId}&username=${username}`;
15 })
16 .catch(error => {
17 console.error('Error joining the room:', error);
18 alert('Failed to join the room. Please try again.');
19 });
20 }
By following these steps, you will have a functional join screen that allows users to input their room ID and username and join a video conference room. The next sections will focus on implementing the main application features, such as controls and participant views, to enhance the user experience further.
Step 4: Implement Controls
In this step, we will implement the basic controls for your Licode WebRTC application. These controls will allow users to mute/unmute their microphone, start/stop their video, and perform other essential actions. We'll also enhance the user experience by adding visual feedback for these actions.
Adding Basic Controls
The controls will be added to the HTML structure created in the previous steps. We'll handle the logic for these controls in the
app.js
file.[a] HTML Structure for Controls
Ensure your
index.html
file includes buttons for the controls within the #controls
div.HTML
1 <div id="controls">
2 <button id="mute-btn">Mute</button>
3 <button id="unmute-btn">Unmute</button>
4 <button id="start-video-btn">Start Video</button>
5 <button id="stop-video-btn">Stop Video</button>
6 </div>
[b] JavaScript for Control Functionalities
In your
app.js
file, add event listeners for these buttons and implement the functionality.JavaScript
1 document.getElementById('mute-btn').addEventListener('click', () => {
2 // Logic to mute the audio
3 muteAudio();
4 });
5
6 document.getElementById('unmute-btn').addEventListener('click', () => {
7 // Logic to unmute the audio
8 unmuteAudio();
9 });
10
11 document.getElementById('start-video-btn').addEventListener('click', () => {
12 // Logic to start the video
13 startVideo();
14 });
15
16 document.getElementById('stop-video-btn').addEventListener('click', () => {
17 // Logic to stop the video
18 stopVideo();
19 });
20
21 function muteAudio() {
22 // Example logic to mute audio
23 console.log('Audio muted');
24 // Add Licode specific code to mute audio
25 localStream.audioEnabled = false;
26 }
27
28 function unmuteAudio() {
29 // Example logic to unmute audio
30 console.log('Audio unmuted');
31 // Add Licode specific code to unmute audio
32 localStream.audioEnabled = true;
33 }
34
35 function startVideo() {
36 // Example logic to start video
37 console.log('Video started');
38 // Add Licode specific code to start video
39 localStream.videoEnabled = true;
40 }
41
42 function stopVideo() {
43 // Example logic to stop video
44 console.log('Video stopped');
45 // Add Licode specific code to stop video
46 localStream.videoEnabled = false;
47 }
Enhancing User Experience
To make the controls more user-friendly, we will add visual feedback indicating the current state of the audio and video streams.
[a] Visual Feedback for Controls
Modify the button texts and styles based on the current state.
JavaScript
1 let isAudioMuted = false;
2 let isVideoStopped = false;
3
4 document.getElementById('mute-btn').addEventListener('click', () => {
5 isAudioMuted = !isAudioMuted;
6 muteAudio();
7 updateControlState();
8 });
9
10 document.getElementById('unmute-btn').addEventListener('click', () => {
11 isAudioMuted = !isAudioMuted;
12 unmuteAudio();
13 updateControlState();
14 });
15
16 document.getElementById('start-video-btn').addEventListener('click', () => {
17 isVideoStopped = !isVideoStopped;
18 startVideo();
19 updateControlState();
20 });
21
22 document.getElementById('stop-video-btn').addEventListener('click', () => {
23 isVideoStopped = !isVideoStopped;
24 stopVideo();
25 updateControlState();
26 });
27
28 function updateControlState() {
29 document.getElementById('mute-btn').innerText = isAudioMuted ? 'Unmute' : 'Mute';
30 document.getElementById('start-video-btn').innerText = isVideoStopped ? 'Start Video' : 'Stop Video';
31 }
32
33 function muteAudio() {
34 // Example logic to mute audio
35 console.log('Audio muted');
36 // Add Licode specific code to mute audio
37 localStream.audioEnabled = false;
38 }
39
40 function unmuteAudio() {
41 // Example logic to unmute audio
42 console.log('Audio unmuted');
43 // Add Licode specific code to unmute audio
44 localStream.audioEnabled = true;
45 }
46
47 function startVideo() {
48 // Example logic to start video
49 console.log('Video started');
50 // Add Licode specific code to start video
51 localStream.videoEnabled = true;
52 }
53
54 function stopVideo() {
55 // Example logic to stop video
56 console.log('Video stopped');
57 // Add Licode specific code to stop video
58 localStream.videoEnabled = false;
59 }
[b] Ensuring Responsive Design
Ensure the controls and other UI elements are responsive and adapt well to different screen sizes.
CSS
1 #controls {
2 width: 100%;
3 max-width: 600px;
4 display: flex;
5 justify-content: space-around;
6 margin: 20px auto;
7 }
8
9 #controls button {
10 flex: 1;
11 margin: 0 5px;
12 padding: 10px;
13 font-size: 16px;
14 }
By implementing these controls, your Licode WebRTC application will allow users to manage their audio and video streams effectively, enhancing the overall user experience. The next sections will cover the implementation of the participant view and other advanced features.
Step 5: Implement Participant View
In this step, we will focus on implementing the participant view for your Licode WebRTC application. The participant view allows users to see and interact with other participants in the video conference. This involves managing video streams and dynamically updating the participant list.
Displaying Participants
To display participants, we need to handle the video streams and update the participant list in real-time as users join or leave the room.
[a] HTML Structure for Participant View
Ensure your
index.html
includes a section for displaying video streams and a participant list.HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>Licode WebRTC App</title>
7 <link rel="stylesheet" href="styles.css">
8 </head>
9 <body>
10 <div id="video-area">
11 <!-- Video streams will be injected here -->
12 </div>
13 <div id="controls">
14 <button id="mute-btn">Mute</button>
15 <button id="unmute-btn">Unmute</button>
16 <button id="start-video-btn">Start Video</button>
17 <button id="stop-video-btn">Stop Video</button>
18 </div>
19 <div id="participant-list">
20 <h3>Participants</h3>
21 <ul id="participants">
22 <!-- Participant names will be listed here -->
23 </ul>
24 </div>
25 <script src="app.js"></script>
26 </body>
27 </html>
[b] JavaScript for Managing Video Streams
In
app.js
, implement functions to handle video streams and update the participant list.JavaScript
1 // Initialize Licode client
2 let licodeClient;
3 let localStream;
4 let remoteStreams = [];
5
6 function initLicode() {
7 // Initialize Licode client and join room
8 const room = 'exampleRoom';
9 const username = 'exampleUser';
10
11 Licode.init({ room, user: username })
12 .then(client => {
13 licodeClient = client;
14 setupLocalStream();
15 setupEventListeners();
16 })
17 .catch(error => {
18 console.error('Error initializing Licode:', error);
19 });
20 }
21
22 function setupLocalStream() {
23 // Create and publish local stream
24 localStream = Licode.createLocalStream({ audio: true, video: true });
25 licodeClient.publish(localStream);
26 addStreamToVideoArea(localStream, true);
27 }
28
29 function setupEventListeners() {
30 // Handle remote stream addition
31 licodeClient.on('stream-added', stream => {
32 licodeClient.subscribe(stream);
33 });
34
35 // Handle remote stream subscription
36 licodeClient.on('stream-subscribed', stream => {
37 remoteStreams.push(stream);
38 addStreamToVideoArea(stream, false);
39 });
40
41 // Handle participant joining
42 licodeClient.on('participant-joined', participant => {
43 addParticipantToList(participant);
44 });
45
46 // Handle participant leaving
47 licodeClient.on('participant-left', participant => {
48 removeParticipantFromList(participant);
49 });
50 }
51
52 function addStreamToVideoArea(stream, isLocal) {
53 const videoArea = document.getElementById('video-area');
54 const videoElement = document.createElement('video');
55 videoElement.srcObject = stream.mediaStream;
56 videoElement.autoplay = true;
57 videoElement.muted = isLocal;
58 videoArea.appendChild(videoElement);
59 }
60
61 function addParticipantToList(participant) {
62 const participantList = document.getElementById('participants');
63 const listItem = document.createElement('li');
64 listItem.textContent = participant.name;
65 listItem.id = `participant-${participant.id}`;
66 participantList.appendChild(listItem);
67 }
68
69 function removeParticipantFromList(participant) {
70 const listItem = document.getElementById(`participant-${participant.id}`);
71 if (listItem) {
72 listItem.remove();
73 }
74 }
75
76 // Initialize Licode when the document is ready
77 document.addEventListener('DOMContentLoaded', initLicode);
Managing Video Streams
Managing multiple video streams efficiently is key to a smooth user experience. We'll handle adding, updating, and removing video streams as participants join or leave.
[a] Handling Multiple Video Streams
Ensure the
addStreamToVideoArea
function can handle multiple streams without performance issues.JavaScript
1 function addStreamToVideoArea(stream, isLocal) {
2 const videoArea = document.getElementById('video-area');
3 const videoContainer = document.createElement('div');
4 videoContainer.className = 'video-container';
5
6 const videoElement = document.createElement('video');
7 videoElement.srcObject = stream.mediaStream;
8 videoElement.autoplay = true;
9 videoElement.muted = isLocal;
10
11 videoContainer.appendChild(videoElement);
12 videoArea.appendChild(videoContainer);
13 }
[b] Optimizing Video Quality and Performance
Use Licode's features to optimize video quality and performance. This includes adjusting video resolution and bitrate dynamically based on network conditions.
JavaScript
1 function setupLocalStream() {
2 // Create and publish local stream with specified options
3 localStream = Licode.createLocalStream({
4 audio: true,
5 video: true,
6 videoSize: [640, 480, 640, 480], // Minimum and maximum video size
7 frameRate: 30,
8 bitrate: 500 // Adjust based on your requirements
9 });
10 licodeClient.publish(localStream);
11 addStreamToVideoArea(localStream, true);
12 }
[c] Handling Stream Removal
Implement logic to handle the removal of streams when participants leave the room.
JavaScript
1 licodeClient.on('stream-removed', stream => {
2 const videoContainer = document.querySelector(`.video-container[data-stream-id="${stream.id}"]`);
3 if (videoContainer) {
4 videoContainer.remove();
5 }
6 });
By implementing these features, you will create a dynamic and responsive participant view that enhances the overall user experience of your Licode WebRTC application. The final step will be to run and test your application to ensure everything is working correctly.
Step 6: Run Your Code Now
With all components set up and implemented, the final step is to run your Licode WebRTC application and ensure everything is working smoothly. This involves testing the application locally, addressing any issues, and preparing for deployment.
Testing the Application Locally
[a] Run the Basic Example
Start by running the basic example provided by Licode to ensure that the core functionalities are working correctly.
bash
1 ./scripts/initBasicExample.sh
[b] Run Your Custom Application
Ensure your custom application files (
index.html
, styles.css
, app.js
) are placed in the appropriate directory, typically the www
folder. Start the Licode server and access your custom application.bash
1 ./scripts/initLicode.sh
Navigate to your custom application's URL, typically
http://localhost:3001
, to test the full functionality.[c] Common Issues and Solutions
- No Video/Audio: Ensure your browser has granted permission to use the camera and microphone.
- Connection Issues: Verify that the Licode server is running and properly configured. Check network configurations and firewall settings.
- Console Errors: Inspect the browser console for any errors. These can provide clues for troubleshooting issues with your code.
Deploying Licode
Once you have tested your application locally and confirmed that everything is working correctly, the next step is to deploy it to a server.
[a] Choose a Hosting Environment
Licode can be deployed on various hosting environments, such as AWS, Google Cloud, or DigitalOcean. Choose an environment that suits your needs and set up a server.
[b] Configure the Server
Install necessary dependencies on the server, including Node.js, Git, and any other required tools.
bash
1 sudo apt-get update
2 sudo apt-get install -y build-essential cmake git nodejs
[c] Clone the Licode Repository
Clone your Licode project to the server and install dependencies.
bash
1 git clone https://github.com/lynckia/licode.git
2 cd licode
3 ./scripts/installUbuntuDeps.sh
4 ./scripts/installErizo.sh
[d] Set Up Environment Variables
Configure environment variables for your server. Edit the
licode_config.js
file to match your server's public IP and other settings.JavaScript
1 var config = {
2 cloudProvider: 'amazon',
3 network: {
4 'publicIP': 'YOUR_SERVER_PUBLIC_IP',
5 'privateIP': 'YOUR_SERVER_PRIVATE_IP',
6 'port': 443
7 },
8 ...
9 };
10 module.exports = config;
[e] Start the Licode Services
Run the initialization scripts to start all necessary services on your server.
bash
1 ./scripts/initLicode.sh
[f] Access Your Application
Open a web browser and navigate to your server's IP address to access your Licode WebRTC application. Ensure that the application functions correctly in the deployed environment.
Conclusion
By following these steps, you have successfully set up and deployed a Licode WebRTC application. This application allows for real-time video conferencing, leveraging the power of Licode's open-source platform. Here, we summarize the key points and address common questions.
Summary of Key Points
- Licode Overview: Licode is an open-source WebRTC platform that uses C++ and Node.js to provide robust real-time communication features.
- Setup and Configuration: Detailed steps to set up the development environment, build Licode, and configure the application.
- UI Implementation: Creation of a join screen, controls, and participant views to enhance user interaction.
- Deployment: Instructions for deploying Licode to a server and accessing the application remotely.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ