Introduction to GO2RTC WebRTC
What is GO2RTC?
GO2RTC
is an ultimate camera streaming application designed to support multiple streaming protocols, including RTSP, RTMP, HTTP-FLV, WebRTC, MSE, HLS, MP4, MJPEG, HomeKit, and FFmpeg. This flexibility makes it an excellent choice for developers looking to implement real-time communication and media streaming capabilities in their applications. GO2RTC is built using the Go programming language, known for its efficiency and performance, which ensures robust and high-performance streaming solutions.Overview of WebRTC Technology
WebRTC, or Web Real-Time Communication, is an open-source project that provides web applications and sites with real-time communication capabilities via simple application programming interfaces (APIs). It enables audio, video, and data sharing between peers, facilitating the creation of rich, interactive multimedia applications. WebRTC's seamless integration with web technologies has revolutionized the way developers build video conferencing, live streaming, and
real-time data
sharing applications.Key Features of GO2RTC
GO2RTC stands out due to its comprehensive support for a wide range of streaming protocols and its integration with popular streaming technologies. Here are some of the key features:
- RTSP (Real-Time Streaming Protocol): Commonly used for streaming video from IP cameras.
- RTMP (Real-Time Messaging Protocol): Widely used for streaming audio, video, and data over the Internet.
- HTTP-FLV (Flash Video): A format for streaming media over HTTP.
- WebRTC: Allows real-time communication directly between browsers without requiring an intermediary server.
- MSE (Media Source Extensions): Enables JavaScript to manage media streams for playback.
- HLS (HTTP Live Streaming): A protocol for streaming content over the web.
- MP4: A digital multimedia format commonly used to store video and audio.
- MJPEG (Motion JPEG): A video format where each video frame is a JPEG image.
- HomeKit: Apple's framework for home automation.
- FFmpeg: A multimedia framework for handling video, audio, and other multimedia files and streams.
GO2RTC leverages these technologies to offer a versatile, high-performance solution for streaming applications, making it a powerful tool for developers.
In the following sections, we will dive deeper into setting up and utilizing GO2RTC, providing step-by-step instructions and code snippets to help you build your own streaming application.
Getting Started with GO2RTC
Creating a New GO2RTC App
To get started with GO2RTC, you'll first need to set up a new project. Follow these steps to create a new GO2RTC app:
[a] Install Go
Ensure that Go is installed on your system. You can download and install it from the official
Go website
.[b] Set Up Your Project Directory
Create a new directory for your GO2RTC project.
sh
1 mkdir go2rtc-app
2 cd go2rtc-app
3
[c] Initialize a New Go Module
Initialize a new Go module in your project directory.
sh
1 go mod init github.com/yourusername/go2rtc-app
2
Installing GO2RTC
Next, you'll need to install GO2RTC. You can do this by cloning the GO2RTC repository and building the project.
[a] Clone the Repository
sh
1 git clone https://github.com/AlexxIT/go2rtc.git
2 cd go2rtc
3
[b] Build the Project
sh
1 go build
2
This command will compile the GO2RTC source code and create an executable file in your project directory.
Structure of the Project
Understanding the structure of your GO2RTC project is crucial for efficient development. Here’s a brief overview of the typical project structure:
- main.go: The entry point of your application.
- config.yaml: Configuration file for setting up various streaming protocols and options.
- handlers/: Directory containing handlers for different streaming endpoints.
- static/: Directory for static assets like HTML, CSS, and JavaScript files.
App Architecture
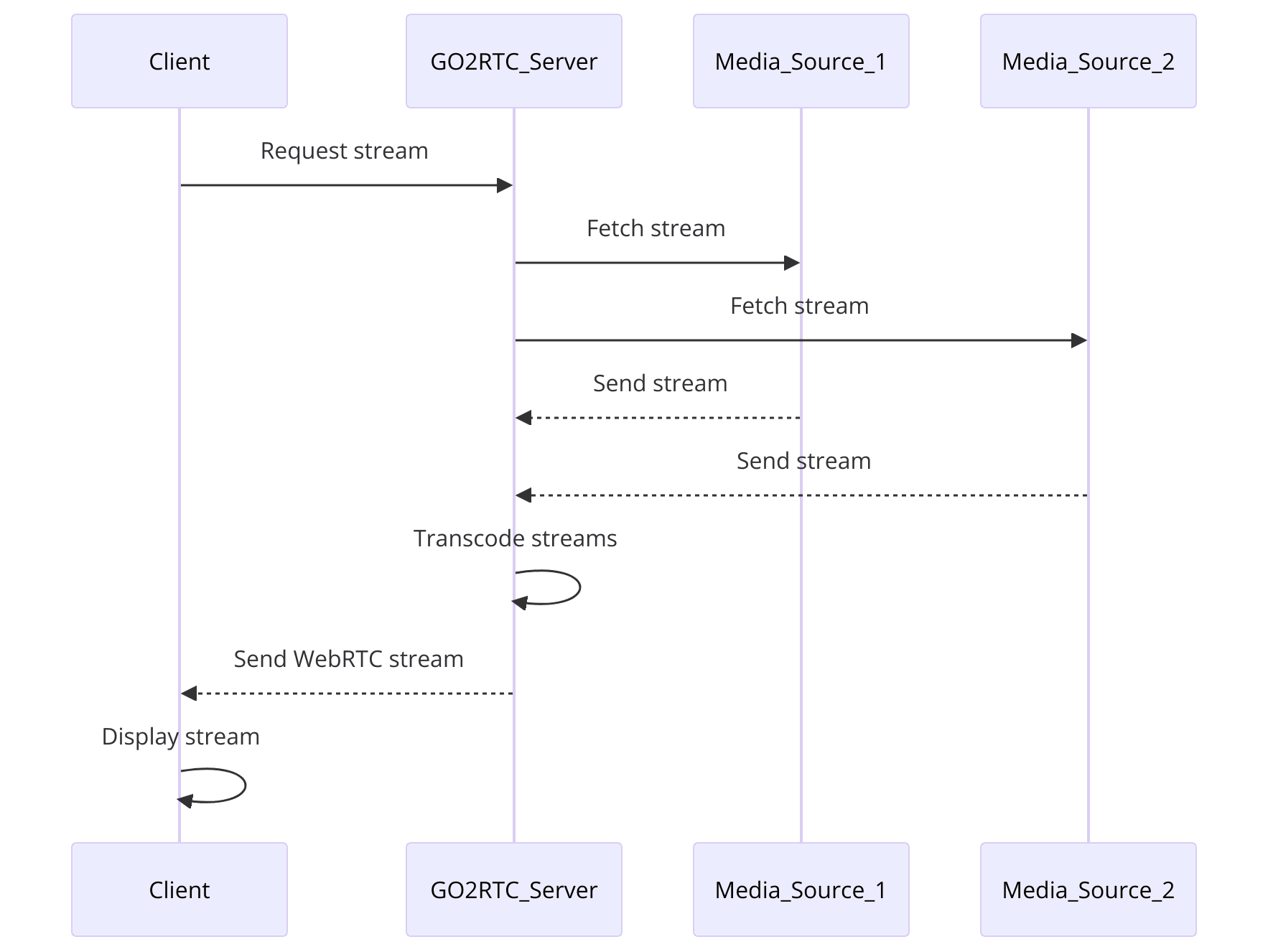
- Input Modules: These modules handle various input streams like RTSP, RTMP, and WebRTC.
- Output Modules: These modules manage output streams to different protocols such as HTTP-FLV, MSE, and HLS.
- Configuration Management: Centralized configuration management to easily switch between different streaming setups.
- Stream Handlers: Functions that process incoming streams and route them to the appropriate output modules.
Step-by-Step Setup
Now that you have an overview of the project structure and architecture, let’s dive into the detailed steps to set up your GO2RTC application.
[a] Setting Up the Environment
Get Started with main.go
Create a
main.go
file in your project directory.go
1 package main
2
3 import (
4 "github.com/AlexxIT/go2rtc/cmd"
5 )
6
7 func main() {
8 cmd.Execute()
9 }
10
This code initializes and starts the GO2RTC application.
Configuring the Project
Create a
config.yaml
file in your project directory to configure your streams.yaml
1 rtsp:
2 - url: rtsp://your_camera_ip_address
3 webhooks:
4 - url: http://localhost:8080
5
[b] Creating the User Interface
Wireframe All the Components
Design the basic layout and user interface for your streaming application. You can use HTML and CSS for a simple UI.
HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <title>GO2RTC Streaming App</title>
6 <style>
7 body { font-family: Arial, sans-serif; }
8 .container { width: 80%; margin: 0 auto; }
9 .stream { margin: 20px 0; }
10 </style>
11 </head>
12 <body>
13 <div class="container">
14 <h1>GO2RTC Streaming App</h1>
15 <div class="stream" id="stream1"></div>
16 </div>
17 </body>
18 </html>
19
Adding Streaming Elements
Use JavaScript to dynamically add streaming elements and manage the streams.
JavaScript
1 document.addEventListener("DOMContentLoaded", function() {
2 const streamContainer = document.getElementById('stream1');
3 const video = document.createElement('video');
4 video.src = 'rtsp://your_camera_ip_address';
5 video.controls = true;
6 streamContainer.appendChild(video);
7 });
8
With these steps, you have set up the basic structure and UI for your GO2RTC streaming application. In the following sections, we will implement more advanced features, such as the join screen, controls, and participant view, to create a fully functional streaming application.
Step 1: Setting Up the Environment
Get Started with main.go
In this section, we will focus on setting up the environment for your GO2RTC project by configuring the
main.go
file and initializing the project.Initial Setup and Configuration
[a] Initialize the Project
Make sure you have Go installed on your system. You can verify the installation by running:
sh
1 go version
2
Initialize a new Go module in your project directory:
sh
1 mkdir go2rtc-app
2 cd go2rtc-app
3 go mod init github.com/yourusername/go2rtc-app
4
[b] Set Up main.go
Create a
main.go
file in your project directory. This file will be the entry point of your application.Go
1 package main
2
3 import (
4 "github.com/AlexxIT/go2rtc/cmd"
5 "github.com/AlexxIT/go2rtc/config"
6 "log"
7 )
8
9 func main() {
10 // Load configuration
11 err := config.Load("config.yaml")
12 if err != nil {
13 log.Fatalf("Error loading config: %v", err)
14 }
15
16 // Execute the main command
17 cmd.Execute()
18 }
19
This code initializes and starts the GO2RTC application by loading the configuration from
config.yaml
and then executing the main command from the GO2RTC library.Code Snippets for Initializing the Project
To ensure a smooth setup, here are the detailed steps and code snippets for configuring your project environment:
[c] Create the Configuration File:
Create a
config.yaml
file in your project directory. This file will contain the necessary configuration settings for your GO2RTC application.Yaml
1 rtsp:
2 - url: rtsp://your_camera_ip_address
3 rtmp:
4 - url: rtmp://your_rtmp_server_address
5 httpflv:
6 - url: http://your_http_flv_server_address
7 webrtc:
8 - url: webrtc://your_webrtc_server_address
9 ffmpeg:
10 - input: rtsp://your_camera_ip_address
11 output: -c:v copy -f flv rtmp://your_rtmp_server_address
12
[d] Install Required Dependencies
Ensure you have the necessary dependencies installed. If you cloned the GO2RTC repository, the required dependencies should be included. If not, you can install them using:
sh
1 go get github.com/AlexxIT/go2rtc
2
[e] Run the Application
Once everything is set up, you can run your GO2RTC application:
sh
1 go run main.go
2
This initial setup will prepare your environment to handle the various streaming protocols supported by GO2RTC. The
main.go
file initializes the application, loads the configuration, and starts the necessary services.Configuring the Project
Understanding the Configuration File
The
config.yaml
file is crucial for setting up your streams. Here is a brief explanation of the configuration options:1 - **RTSP**: URL of the RTSP stream (e.g., from an IP camera).
2 - **RTMP**: URL of the RTMP server to which the stream will be sent.
3 - **HTTP-FLV**: URL for HTTP-FLV streaming.
4 - **WebRTC**: URL for WebRTC streaming.
5 - **FFmpeg**: Command for processing streams using FFmpeg.
Customizing the Configuration
Modify the
config.yaml
file to suit your specific requirements. For example, you can add multiple RTSP sources or configure different output formats.With the environment set up and the initial configuration in place, you are now ready to proceed with creating the user interface and implementing the core features of your GO2RTC application. In the next section, we will focus on designing and building the user interface, ensuring a user-friendly experience for streaming and managing video feeds.
Step 2: Creating the User Interface
Wireframe All the Components
In this section, we will design and implement the user interface for your GO2RTC streaming application. The user interface is crucial for providing a seamless experience for users to interact with the streaming functionalities.
Design the Basic Layout and User Interface
[a] HTML Structure
Start by creating an
index.html
file in your project directory. This file will contain the basic structure of your application.HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>GO2RTC Streaming App</title>
7 <link rel="stylesheet" href="styles.css">
8 </head>
9 <body>
10 <div class="container">
11 <header>
12 <h1>GO2RTC Streaming App</h1>
13 </header>
14 <main>
15 <div class="stream" id="stream1">
16 <h2>Stream 1</h2>
17 <video id="video1" controls autoplay></video>
18 </div>
19 <div class="stream" id="stream2">
20 <h2>Stream 2</h2>
21 <video id="video2" controls autoplay></video>
22 </div>
23 </main>
24 <footer>
25 <p>© 2024 GO2RTC Streaming App</p>
26 </footer>
27 </div>
28 <script src="scripts.js"></script>
29 </body>
30 </html>
31
[b] CSS for Styling
Create a
styles.css
file to style the application. This will enhance the visual appeal and ensure a responsive design.CSS
1 body {
2 font-family: Arial, sans-serif;
3 margin: 0;
4 padding: 0;
5 background-color: #f4f4f4;
6 }
7
8 .container {
9 width: 80%;
10 margin: 0 auto;
11 padding: 20px;
12 background-color: #fff;
13 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
14 }
15
16 header {
17 text-align: center;
18 margin-bottom: 20px;
19 }
20
21 main {
22 display: flex;
23 justify-content: space-between;
24 }
25
26 .stream {
27 width: 48%;
28 background-color: #ececec;
29 padding: 10px;
30 border-radius: 5px;
31 box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
32 }
33
34 video {
35 width: 100%;
36 height: auto;
37 border-radius: 5px;
38 }
39
40 footer {
41 text-align: center;
42 margin-top: 20px;
43 padding-top: 10px;
44 border-top: 1px solid #ddd;
45 }
46
Adding Streaming Elements
[c] JavaScript for Managing Streams
Create a
scripts.js
file to handle the video streams and manage their playback.JavaScript
1 document.addEventListener("DOMContentLoaded", function() {
2 // Get video elements
3 const video1 = document.getElementById('video1');
4 const video2 = document.getElementById('video2');
5
6 // Set stream sources
7 video1.src = 'rtsp://your_camera_ip_address1';
8 video2.src = 'rtsp://your_camera_ip_address2';
9
10 // Play videos
11 video1.play();
12 video2.play();
13 });
14
[d] Enhancing the User Experience
Add functionality to manage the video streams more effectively, such as play, pause, and stop controls. Update the
scripts.js
file as needed.JavaScript
1 document.addEventListener("DOMContentLoaded", function() {
2 const video1 = document.getElementById('video1');
3 const video2 = document.getElementById('video2');
4
5 // Function to initialize video streams
6 function initVideoStream(videoElement, streamUrl) {
7 videoElement.src = streamUrl;
8 videoElement.play().catch(error => {
9 console.error('Error playing video:', error);
10 });
11 }
12
13 // Initialize streams
14 initVideoStream(video1, 'rtsp://your_camera_ip_address1');
15 initVideoStream(video2, 'rtsp://your_camera_ip_address2');
16 });
17
With these steps, you have created a basic but functional user interface for your GO2RTC streaming application. The HTML structure provides a clean layout, while the CSS ensures the application looks good across different devices. The JavaScript handles the video streams, enabling users to view the streams directly from their browsers.
In the next section, we will implement the join screen, which will allow users to join streams and interact with the application more dynamically. This will include user authentication and session management to provide a secure and personalized streaming experience.
Step 3: Implementing the Join Screen
In this section, we will implement a join screen for your GO2RTC streaming application. The join screen allows users to enter a stream by providing necessary credentials or information, ensuring secure and personalized access to the streaming service.
Implement Join Screen
Creating the Join Screen Interface
[a] HTML for Join Screen
Modify your
index.html
file to include a join screen that users will see before accessing the streams.HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>GO2RTC Streaming App</title>
7 <link rel="stylesheet" href="styles.css">
8 </head>
9 <body>
10 <div class="container">
11 <header>
12 <h1>GO2RTC Streaming App</h1>
13 </header>
14 <div id="join-screen">
15 <h2>Join Stream</h2>
16 <form id="join-form">
17 <label for="username">Username:</label>
18 <input type="text" id="username" name="username" required>
19 <label for="stream-key">Stream Key:</label>
20 <input type="text" id="stream-key" name="stream-key" required>
21 <button type="submit">Join</button>
22 </form>
23 </div>
24 <main id="stream-screen" style="display: none;">
25 <div class="stream" id="stream1">
26 <h2>Stream 1</h2>
27 <video id="video1" controls autoplay></video>
28 </div>
29 <div class="stream" id="stream2">
30 <h2>Stream 2</h2>
31 <video id="video2" controls autoplay></video>
32 </div>
33 </main>
34 <footer>
35 <p>© 2024 GO2RTC Streaming App</p>
36 </footer>
37 </div>
38 <script src="scripts.js"></script>
39 </body>
40 </html>
41
[b] CSS for Join Screen
Update your
styles.css
file to style the join screen form.CSS
1 body {
2 font-family: Arial, sans-serif;
3 margin: 0;
4 padding: 0;
5 background-color: #f4f4f4;
6 }
7
8 .container {
9 width: 80%;
10 margin: 0 auto;
11 padding: 20px;
12 background-color: #fff;
13 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
14 }
15
16 header {
17 text-align: center;
18 margin-bottom: 20px;
19 }
20
21 #join-screen {
22 text-align: center;
23 }
24
25 form {
26 display: inline-block;
27 text-align: left;
28 margin-top: 20px;
29 }
30
31 label {
32 display: block;
33 margin-bottom: 8px;
34 }
35
36 input {
37 width: 100%;
38 padding: 8px;
39 margin-bottom: 10px;
40 border: 1px solid #ccc;
41 border-radius: 4px;
42 }
43
44 button {
45 padding: 10px 20px;
46 background-color: #4CAF50;
47 color: #fff;
48 border: none;
49 border-radius: 4px;
50 cursor: pointer;
51 }
52
53 button:hover {
54 background-color: #45a049;
55 }
56
57 main {
58 display: flex;
59 justify-content: space-between;
60 }
61
62 .stream {
63 width: 48%;
64 background-color: #ececec;
65 padding: 10px;
66 border-radius: 5px;
67 box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
68 }
69
70 video {
71 width: 100%;
72 height: auto;
73 border-radius: 5px;
74 }
75
76 footer {
77 text-align: center;
78 margin-top: 20px;
79 padding-top: 10px;
80 border-top: 1px solid #ddd;
81 }
82
Implementing the Join Screen Functionality*
[c] JavaScript for Join Screen
Update your
scripts.js
file to handle the join form submission and validate the user before displaying the streams.JavaScript
1 document.addEventListener("DOMContentLoaded", function() {
2 const joinForm = document.getElementById('join-form');
3 const joinScreen = document.getElementById('join-screen');
4 const streamScreen = document.getElementById('stream-screen');
5 const video1 = document.getElementById('video1');
6 const video2 = document.getElementById('video2');
7
8 joinForm.addEventListener('submit', function(event) {
9 event.preventDefault();
10
11 // Retrieve form values
12 const username = document.getElementById('username').value;
13 const streamKey = document.getElementById('stream-key').value;
14
15 // Validate credentials (for simplicity, we just check if not empty)
16 if (username && streamKey) {
17 // Hide join screen and show stream screen
18 joinScreen.style.display = 'none';
19 streamScreen.style.display = 'flex';
20
21 // Initialize video streams
22 initVideoStream(video1, 'rtsp://your_camera_ip_address1');
23 initVideoStream(video2, 'rtsp://your_camera_ip_address2');
24 } else {
25 alert('Please enter valid credentials.');
26 }
27 });
28
29 // Function to initialize video streams
30 function initVideoStream(videoElement, streamUrl) {
31 videoElement.src = streamUrl;
32 videoElement.play().catch(error => {
33 console.error('Error playing video:', error);
34 });
35 }
36 });
37
With these steps, you have implemented a join screen that allows users to enter their credentials before accessing the streams. This not only provides a layer of security but also ensures a personalized experience for the users.
The join screen interface prompts users for their username and stream key, and upon validation, it displays the streaming interface with the video streams. This approach makes the application user-friendly and secure.
In the next section, we will focus on implementing controls for managing the video streams, such as play, pause, and stop functionalities, to enhance user interaction with the streaming content.
Step 4: Implementing Controls
In this section, we will add controls to manage the video streams in your GO2RTC application. These controls will enhance user interaction by allowing them to play, pause, and stop the video streams.
Implement Controls
Enhancing the User Interface with Controls
[a] HTML for Controls
Modify your
index.html
file to include control buttons for each video stream.HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>GO2RTC Streaming App</title>
7 <link rel="stylesheet" href="styles.css">
8 </head>
9 <body>
10 <div class="container">
11 <header>
12 <h1>GO2RTC Streaming App</h1>
13 </header>
14 <div id="join-screen">
15 <h2>Join Stream</h2>
16 <form id="join-form">
17 <label for="username">Username:</label>
18 <input type="text" id="username" name="username" required>
19 <label for="stream-key">Stream Key:</label>
20 <input type="text" id="stream-key" name="stream-key" required>
21 <button type="submit">Join</button>
22 </form>
23 </div>
24 <main id="stream-screen" style="display: none;">
25 <div class="stream" id="stream1">
26 <h2>Stream 1</h2>
27 <video id="video1" controls autoplay></video>
28 <div class="controls">
29 <button id="play1">Play</button>
30 <button id="pause1">Pause</button>
31 <button id="stop1">Stop</button>
32 </div>
33 </div>
34 <div class="stream" id="stream2">
35 <h2>Stream 2</h2>
36 <video id="video2" controls autoplay></video>
37 <div class="controls">
38 <button id="play2">Play</button>
39 <button id="pause2">Pause</button>
40 <button id="stop2">Stop</button>
41 </div>
42 </div>
43 </main>
44 <footer>
45 <p>© 2024 GO2RTC Streaming App</p>
46 </footer>
47 </div>
48 <script src="scripts.js"></script>
49 </body>
50 </html>
51
[b] CSS for Controls
Update your
styles.css
file to style the control buttons.CSS
1 body {
2 font-family: Arial, sans-serif;
3 margin: 0;
4 padding: 0;
5 background-color: #f4f4f4;
6 }
7
8 .container {
9 width: 80%;
10 margin: 0 auto;
11 padding: 20px;
12 background-color: #fff;
13 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
14 }
15
16 header {
17 text-align: center;
18 margin-bottom: 20px;
19 }
20
21 #join-screen {
22 text-align: center;
23 }
24
25 form {
26 display: inline-block;
27 text-align: left;
28 margin-top: 20px;
29 }
30
31 label {
32 display: block;
33 margin-bottom: 8px;
34 }
35
36 input {
37 width: 100%;
38 padding: 8px;
39 margin-bottom: 10px;
40 border: 1px solid #ccc;
41 border-radius: 4px;
42 }
43
44 button {
45 padding: 10px 20px;
46 background-color: #4CAF50;
47 color: #fff;
48 border: none;
49 border-radius: 4px;
50 cursor: pointer;
51 margin-right: 5px;
52 }
53
54 button:hover {
55 background-color: #45a049;
56 }
57
58 main {
59 display: flex;
60 justify-content: space-between;
61 }
62
63 .stream {
64 width: 48%;
65 background-color: #ececec;
66 padding: 10px;
67 border-radius: 5px;
68 box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
69 }
70
71 video {
72 width: 100%;
73 height: auto;
74 border-radius: 5px;
75 }
76
77 .controls {
78 text-align: center;
79 margin-top: 10px;
80 }
81
82 footer {
83 text-align: center;
84 margin-top: 20px;
85 padding-top: 10px;
86 border-top: 1px solid #ddd;
87 }
88
Implementing JavaScript Functions for Controls
[c] JavaScript for Controls
Update your
scripts.js
file to handle the control buttons' functionality for each video stream.JavaScript
1 document.addEventListener("DOMContentLoaded", function() {
2 const joinForm = document.getElementById('join-form');
3 const joinScreen = document.getElementById('join-screen');
4 const streamScreen = document.getElementById('stream-screen');
5 const video1 = document.getElementById('video1');
6 const video2 = document.getElementById('video2');
7
8 joinForm.addEventListener('submit', function(event) {
9 event.preventDefault();
10
11 // Retrieve form values
12 const username = document.getElementById('username').value;
13 const streamKey = document.getElementById('stream-key').value;
14
15 // Validate credentials (for simplicity, we just check if not empty)
16 if (username && streamKey) {
17 // Hide join screen and show stream screen
18 joinScreen.style.display = 'none';
19 streamScreen.style.display = 'flex';
20
21 // Initialize video streams
22 initVideoStream(video1, 'rtsp://your_camera_ip_address1');
23 initVideoStream(video2, 'rtsp://your_camera_ip_address2');
24 } else {
25 alert('Please enter valid credentials.');
26 }
27 });
28
29 // Function to initialize video streams
30 function initVideoStream(videoElement, streamUrl) {
31 videoElement.src = streamUrl;
32 videoElement.play().catch(error => {
33 console.error('Error playing video:', error);
34 });
35 }
36
37 // Add event listeners for controls
38 document.getElementById('play1').addEventListener('click', function() {
39 video1.play().catch(error => {
40 console.error('Error playing video:', error);
41 });
42 });
43
44 document.getElementById('pause1').addEventListener('click', function() {
45 video1.pause();
46 });
47
48 document.getElementById('stop1').addEventListener('click', function() {
49 video1.pause();
50 video1.currentTime = 0;
51 });
52
53 document.getElementById('play2').addEventListener('click', function() {
54 video2.play().catch(error => {
55 console.error('Error playing video:', error);
56 });
57 });
58
59 document.getElementById('pause2').addEventListener('click', function() {
60 video2.pause();
61 });
62
63 document.getElementById('stop2').addEventListener('click', function() {
64 video2.pause();
65 video2.currentTime = 0;
66 });
67 });
68
With these steps, you have successfully implemented controls for the video streams in your GO2RTC application. The HTML structure includes control buttons for each stream, the CSS styles these buttons, and the JavaScript handles the play, pause, and stop functionalities.
The control buttons enhance the user experience by providing intuitive ways to manage the video streams, making the application more interactive and user-friendly.
In the next section, we will implement the participant view, which will allow displaying multiple participants' streams and managing interactions, further enhancing the application's functionality.
Step 5: Implementing Participant View
In this section, we will implement a participant view for your GO2RTC streaming application. The participant view allows displaying multiple participants' streams, making it ideal for scenarios like video conferencing or multi-camera setups.
Implement Participant View
Enhancing the User Interface for Multiple Participants
[a] HTML for Participant View
Modify your
index.html
file to accommodate multiple participant streams.HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>GO2RTC Streaming App</title>
7 <link rel="stylesheet" href="styles.css">
8 </head>
9 <body>
10 <div class="container">
11 <header>
12 <h1>GO2RTC Streaming App</h1>
13 </header>
14 <div id="join-screen">
15 <h2>Join Stream</h2>
16 <form id="join-form">
17 <label for="username">Username:</label>
18 <input type="text" id="username" name="username" required>
19 <label for="stream-key">Stream Key:</label>
20 <input type="text" id="stream-key" name="stream-key" required>
21 <button type="submit">Join</button>
22 </form>
23 </div>
24 <main id="stream-screen" style="display: none;">
25 <div class="participant" id="participant1">
26 <h2>Participant 1</h2>
27 <video id="video1" controls autoplay></video>
28 <div class="controls">
29 <button id="play1">Play</button>
30 <button id="pause1">Pause</button>
31 <button id="stop1">Stop</button>
32 </div>
33 </div>
34 <div class="participant" id="participant2">
35 <h2>Participant 2</h2>
36 <video id="video2" controls autoplay></video>
37 <div class="controls">
38 <button id="play2">Play</button>
39 <button id="pause2">Pause</button>
40 <button id="stop2">Stop</button>
41 </div>
42 </div>
43 <div class="participant" id="participant3">
44 <h2>Participant 3</h2>
45 <video id="video3" controls autoplay></video>
46 <div class="controls">
47 <button id="play3">Play</button>
48 <button id="pause3">Pause</button>
49 <button id="stop3">Stop</button>
50 </div>
51 </div>
52 <div class="participant" id="participant4">
53 <h2>Participant 4</h2>
54 <video id="video4" controls autoplay></video>
55 <div class="controls">
56 <button id="play4">Play</button>
57 <button id="pause4">Pause</button>
58 <button id="stop4">Stop</button>
59 </div>
60 </div>
61 </main>
62 <footer>
63 <p>© 2024 GO2RTC Streaming App</p>
64 </footer>
65 </div>
66 <script src="scripts.js"></script>
67 </body>
68 </html>
69
[b] CSS for Participant View
Update your
styles.css
file to style the participant streams.CSS
1 body {
2 font-family: Arial, sans-serif;
3 margin: 0;
4 padding: 0;
5 background-color: #f4f4f4;
6 }
7
8 .container {
9 width: 80%;
10 margin: 0 auto;
11 padding: 20px;
12 background-color: #fff;
13 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
14 }
15
16 header {
17 text-align: center;
18 margin-bottom: 20px;
19 }
20
21 #join-screen {
22 text-align: center;
23 }
24
25 form {
26 display: inline-block;
27 text-align: left;
28 margin-top: 20px;
29 }
30
31 label {
32 display: block;
33 margin-bottom: 8px;
34 }
35
36 input {
37 width: 100%;
38 padding: 8px;
39 margin-bottom: 10px;
40 border: 1px solid #ccc;
41 border-radius: 4px;
42 }
43
44 button {
45 padding: 10px 20px;
46 background-color: #4CAF50;
47 color: #fff;
48 border: none;
49 border-radius: 4px;
50 cursor: pointer;
51 margin-right: 5px;
52 }
53
54 button:hover {
55 background-color: #45a049;
56 }
57
58 main {
59 display: flex;
60 flex-wrap: wrap;
61 justify-content: space-between;
62 }
63
64 .participant {
65 width: 48%;
66 background-color: #ececec;
67 padding: 10px;
68 margin-bottom: 20px;
69 border-radius: 5px;
70 box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
71 }
72
73 video {
74 width: 100%;
75 height: auto;
76 border-radius: 5px;
77 }
78
79 .controls {
80 text-align: center;
81 margin-top: 10px;
82 }
83
84 footer {
85 text-align: center;
86 margin-top: 20px;
87 padding-top: 10px;
88 border-top: 1px solid #ddd;
89 }
90
Implementing JavaScript Functions for Multiple Participants
[c] JavaScript for Participant Streams
Update your
scripts.js
file to handle the video streams and control functionalities for multiple participants.JavaScript
1 document.addEventListener("DOMContentLoaded", function() {
2 const joinForm = document.getElementById('join-form');
3 const joinScreen = document.getElementById('join-screen');
4 const streamScreen = document.getElementById('stream-screen');
5
6 // Participant video elements
7 const videos = [
8 document.getElementById('video1'),
9 document.getElementById('video2'),
10 document.getElementById('video3'),
11 document.getElementById('video4')
12 ];
13
14 // Control buttons
15 const controls = [
16 { play: document.getElementById('play1'), pause: document.getElementById('pause1'), stop: document.getElementById('stop1') },
17 { play: document.getElementById('play2'), pause: document.getElementById('pause2'), stop: document.getElementById('stop2') },
18 { play: document.getElementById('play3'), pause: document.getElementById('pause3'), stop: document.getElementById('stop3') },
19 { play: document.getElementById('play4'), pause: document.getElementById('pause4'), stop: document.getElementById('stop4') }
20 ];
21
22 joinForm.addEventListener('submit', function(event) {
23 event.preventDefault();
24
25 // Retrieve form values
26 const username = document.getElementById('username').value;
27 const streamKey = document.getElementById('stream-key').value;
28
29 // Validate credentials (for simplicity, we just check if not empty)
30 if (username && streamKey) {
31 // Hide join screen and show stream screen
32 joinScreen.style.display = 'none';
33 streamScreen.style.display = 'flex';
34
35 // Initialize video streams
36 initVideoStream(videos[0], 'rtsp://your_camera_ip_address1');
37 initVideoStream(videos[1], 'rtsp://your_camera_ip_address2');
38 initVideoStream(videos[2], 'rtsp://your_camera_ip_address3');
39 initVideoStream(videos[3], 'rtsp://your_camera_ip_address4');
40 } else {
41 alert('Please enter valid credentials.');
42 }
43 });
44
45 // Function to initialize video streams
46 function initVideoStream(videoElement, streamUrl) {
47 videoElement.src = streamUrl;
48 videoElement.play().catch(error => {
49 console.error('Error playing video:', error);
50 });
51 }
52
53 // Add event listeners for controls
54 controls.forEach((control, index) => {
55 control.play.addEventListener('click', function() {
56 videos[index].play().catch(error => {
57 console.error('Error playing video:', error);
58 });
59 });
60
61 control.pause.addEventListener('click', function() {
62 videos[index].pause();
63 });
64
65 control.stop.addEventListener('click', function() {
66 videos[index].pause();
67 videos[index].currentTime = 0;
68 });
69 });
70 });
71
With these steps, you have successfully implemented a participant view that allows displaying multiple streams simultaneously. The HTML structure includes multiple participant sections, the CSS styles these sections, and the JavaScript manages the video streams and their controls.
The participant view enhances the application's functionality by supporting multiple streams, making it suitable for video conferencing and other multi-camera scenarios.
In the next section, we will focus on running your code to ensure everything works as expected and troubleshooting any common issues that may arise.
Step 6: Running Your Code
In this section, we will focus on running your GO2RTC application to ensure everything works as expected. We will also cover troubleshooting common issues that may arise during the process.
Running Your Code
[a] Ensure Dependencies are Installed
Before running your application, make sure all the necessary dependencies are installed. If you haven't already, navigate to your project directory and install the required Go packages:
sh
1 go mod tidy
2
[b] Run the GO2RTC Application
Use the following command to run your application:
sh
1 go run main.go
2
This command will start your GO2RTC application, initializing the streams and setting up the web server to serve your HTML interface.
[c] Access the Application in a Browser
- Open your web browser and navigate to
http://localhost:8080
(or the port specified in your GO2RTC configuration). - You should see the join screen where you can enter your username and stream key.
[d] Join the Stream
Enter a valid username and stream key, then click the "Join" button. If the credentials are valid, the join screen will be replaced by the participant view, displaying the video streams.
Troubleshooting Common Issues
Common Issues and Solutions
Video Stream Not Playing
- Check Stream URLs: Ensure the RTSP/RTMP/HTTP-FLV/WebRTC URLs in your
config.yaml
file are correct and accessible. - Console Errors: Open the browser console (F12) to check for any errors. Common issues include incorrect stream URLs, network problems, or CORS issues.
- Firewall and Network Settings: Make sure there are no firewall or network settings blocking the streams.
Credentials Validation Failure
- Input Fields: Ensure the username and stream key fields are not empty.
- Form Handling: Verify that the form submission is correctly handled in your
scripts.js
file. - Configuration: Check if the validation logic (if any) for the credentials is correctly implemented.
Application Crashes or Errors
- Go Code: Check the logs in your terminal for any errors in the Go code. Common issues might include configuration file errors or missing dependencies.
- Debugging: Use Go’s debugging tools to step through the code and identify issues.
Stream Control Issues
- Button Functionality: Ensure the play, pause, and stop buttons are correctly wired to their respective video elements.
- JavaScript Errors: Check for any JavaScript errors in the browser console that might prevent the controls from working.
Example Debugging Session
Here’s an example of how you might debug an issue where the video stream is not playing:
- Open the Browser Console: Press
F12
to open the developer tools and navigate to the "Console" tab. - Check for Errors: Look for any error messages related to the video elements or the stream URLs.
- Verify Stream URL: Copy the stream URL from your
config.yaml
and try to access it directly in a media player like VLC to verify it’s working. - Network Tab: Use the "Network" tab in the developer tools to check if the video streams are being requested correctly and if there are any network-related issues.
- Adjust Configuration: If the issue is related to the configuration, make the necessary changes in your
config.yaml
file and restart the application.
By following these steps, you should be able to successfully run your GO2RTC application and troubleshoot any issues that arise.
Conclusion
In this article, we have covered the setup and implementation of a GO2RTC streaming application using WebRTC technology. We started with the basic environment setup, moved on to creating a user interface, implemented a join screen, added controls for video streams, and finally, implemented a participant view.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ