The fitness app market is booming, with an increasing demand for personalized experiences driving innovation in the space. As users seek more comprehensive insights into their health and fitness journeys, developers face both challenges and opportunities in integrating diverse fitness data. This is where fitness APIs come into play.
Fitness APIs serve as critical connectors in the health tech ecosystem, enabling developers to build sophisticated applications that leverage data from various sources. From activity tracking to nutrition analysis and wearable device integration, these APIs offer powerful capabilities for creating engaging and effective fitness solutions. In this guide, we'll explore different types of fitness APIs, their applications, and how developers can harness their potential to build innovative fitness apps that stand out in a competitive market.
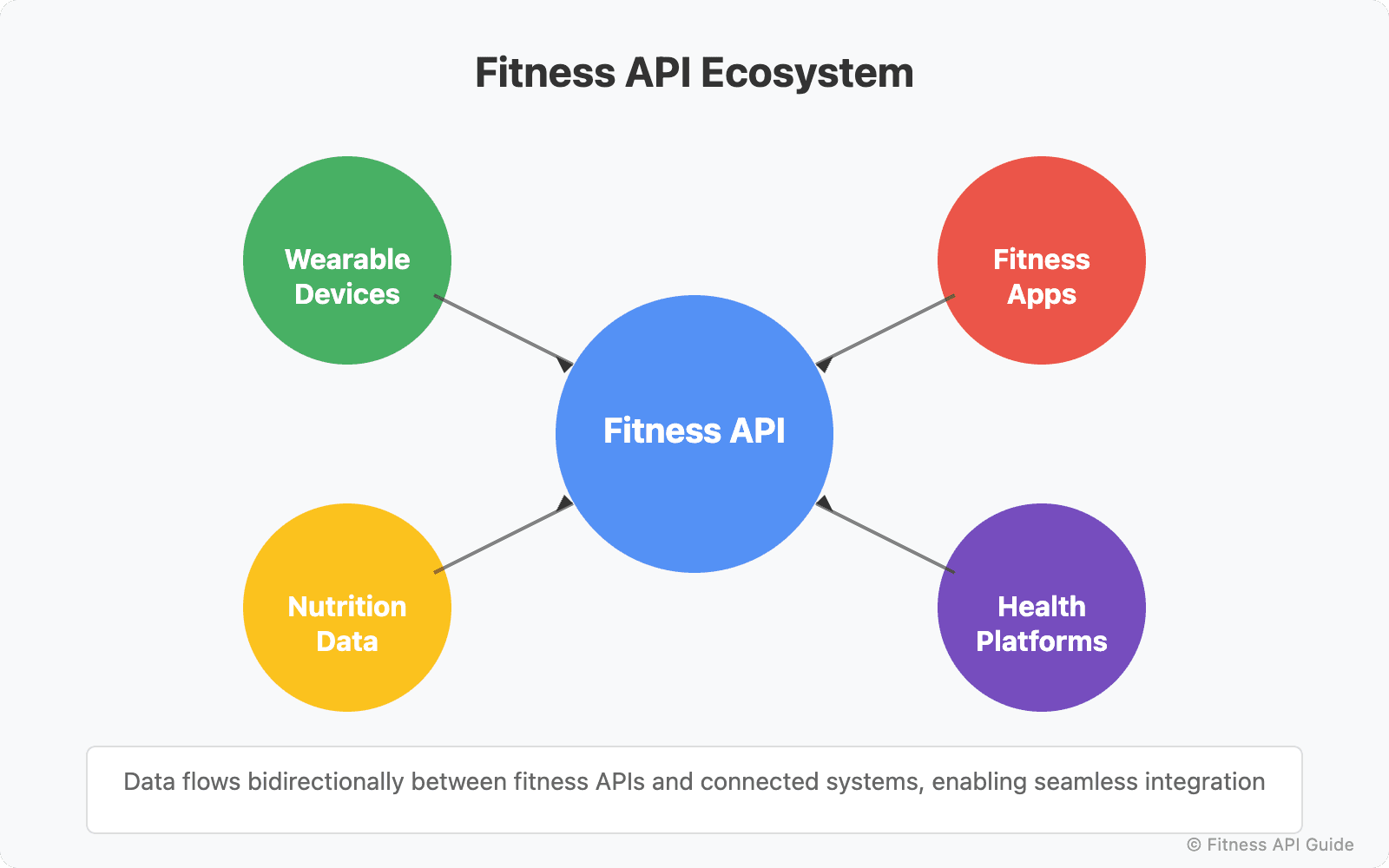
What is a Fitness API?
A fitness API (Application Programming Interface) is a software interface that allows different applications to exchange fitness-related data. Acting as a bridge between various fitness devices, apps, and platforms, these APIs facilitate seamless communication and data sharing across the fitness technology ecosystem.
Fitness APIs typically handle a wide range of data types, including:
- Physical activity metrics (steps, distance, calories burned)
- Biometric data (heart rate, blood pressure, body temperature)
- Sleep statistics (duration, quality, stages)
- Nutrition information (calorie intake, macronutrients, food logs)
- Workout details (exercise type, duration, intensity)
- Location data (routes, elevation, pace)
By providing standardized methods for accessing and exchanging this information, fitness APIs enable developers to create more comprehensive and integrated fitness experiences for users without having to build every feature from scratch.
Types of Fitness APIs
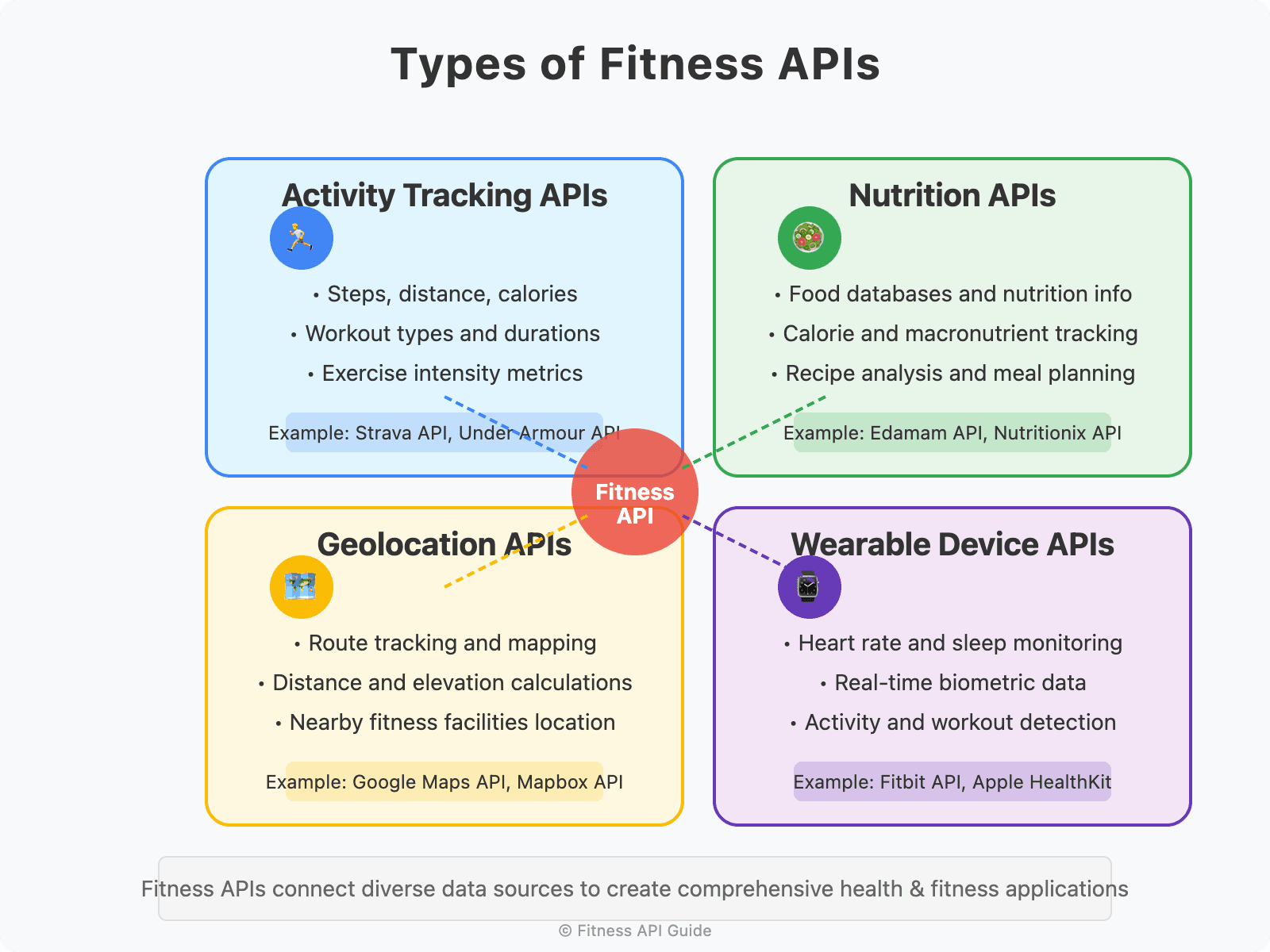
Activity Tracking APIs
Activity tracking APIs focus on data related to physical movement and exercise. These APIs provide access to metrics such as steps taken, distance covered, calories burned, and workout types.
Examples:
- Strava API: Popular among runners and cyclists, offering detailed activity tracking and social features
- Under Armour API: Part of the UA Connected Fitness platform, providing comprehensive activity data
- Garmin Health API: Offering activity metrics from Garmin wearable devices
Nutrition APIs
Nutrition APIs provide access to food databases, nutritional information, and dietary analysis tools. These APIs enable developers to incorporate food tracking and nutritional guidance into their fitness applications.
Examples:
- Edamam Nutrition Analysis API: Offering extensive food database and nutrient analysis
- FoodData Central API: Providing access to the USDA's comprehensive food composition database
- Nutritionix API: Featuring natural language processing for easy food logging and analysis
Geolocation and Mapping APIs
Geolocation and mapping APIs offer location-based services crucial for tracking routes, finding nearby fitness facilities, and calculating distances. These APIs enhance fitness apps with spatial context.
Examples:
- Google Maps APIs: Providing comprehensive mapping and location services
- Apple MapKit APIs: Offering mapping integration for iOS applications
- Mapbox API: Delivering customizable maps and location intelligence
Wearable Device APIs
Wearable device APIs connect to smartwatches, fitness trackers, and other wearable technology to retrieve real-time and historical fitness data directly from these devices.
Examples:
- Fitbit Web API: Accessing data from Fitbit's range of fitness trackers and smartwatches
- Apple HealthKit API: Integrating with Apple Watch and other compatible health devices
- Garmin Health API: Connecting with Garmin's ecosystem of fitness wearables
Key Benefits of Using a Fitness API
Enhanced User Experience
Fitness APIs enable the creation of personalized insights and recommendations based on aggregated data from multiple sources. This holistic approach provides users with a more comprehensive understanding of their health and fitness status. By integrating with users' favorite apps and devices, fitness applications can deliver a seamless experience that fits naturally into their daily routines.
Data-Driven Decision Making
Access to rich fitness data empowers users to make informed decisions about their health and wellness. With detailed tracking of progress over time, users can optimize their fitness routines based on objective metrics rather than guesswork. Fitness APIs also enable the identification of trends and patterns in user behavior, leading to better health management and more sustainable lifestyle changes.
App Development Efficiency
For developers, fitness APIs offer pre-built functionalities for data retrieval and analysis, significantly reducing development time and resources. Rather than building complex data collection and processing systems from scratch, developers can leverage existing APIs to focus on creating unique value for their users. Additionally, fitness APIs provide access to a wider ecosystem of fitness-related services, expanding the potential capabilities of fitness applications.
Building Niche Communities
Social elements powered by fitness APIs can help foster engaged communities within fitness apps. Features like activity feeds, challenges, and shared workouts create opportunities for users to connect around common fitness goals. This social dimension not only enhances user engagement but also improves retention rates as users become part of a supportive community.
Integrating a Fitness API: A Step-by-Step Guide
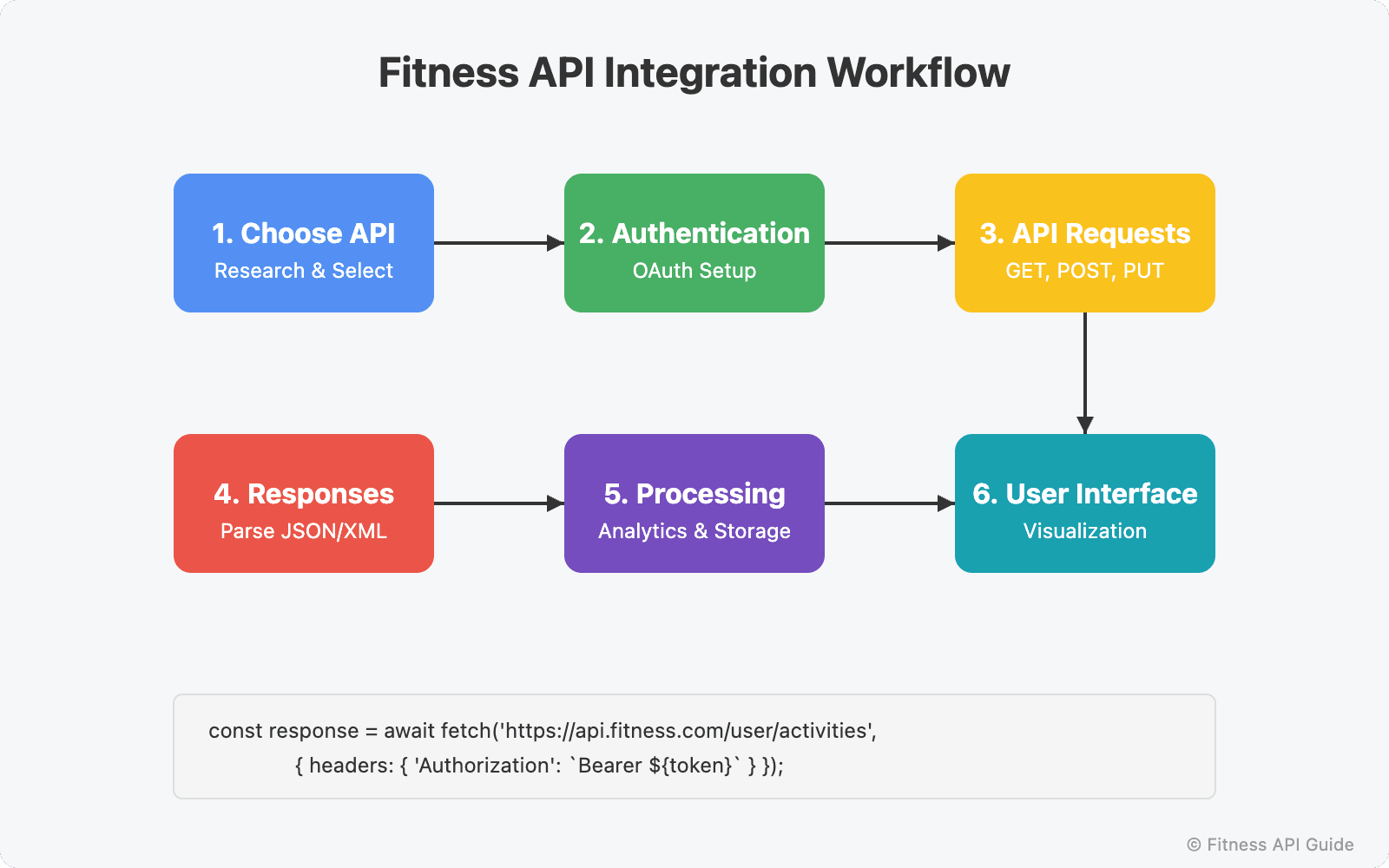
Choosing the Right API
Selecting the appropriate fitness API for your application requires careful consideration of several factors:
- Data types offered: Ensure the API provides access to the specific fitness metrics your application needs
- Pricing: Evaluate the cost structure and whether it aligns with your business model
- Documentation: Look for comprehensive, clear documentation to facilitate implementation
- API stability: Consider the API's track record for reliability and backward compatibility
- Scalability: Assess whether the API can handle your expected user growth
- Support: Check for responsive support channels and an active developer community
- Privacy policies: Verify HIPAA compliance and other privacy protections for handling sensitive health data
Authentication and Authorization
Fitness APIs typically employ robust security measures to protect sensitive user data:
- API keys: Simple authentication tokens that identify your application
- OAuth: An authorization framework that allows users to grant limited access to their data without sharing credentials
- JWT (JSON Web Tokens): Secure tokens for transferring claims between parties
Implementing proper authentication not only ensures compliance with the API provider's requirements but also builds trust with users concerned about their personal health data.
Making API Requests
Most fitness APIs follow REST (Representational State Transfer) principles, using standard HTTP methods:
- GET: Retrieve data (e.g., fetching a user's step count)
- POST: Create new data (e.g., logging a completed workout)
- PUT: Update existing data (e.g., modifying workout details)
- DELETE: Remove data (e.g., deleting an erroneous activity log)
Example of a GET request to retrieve user activity data:
1const fetchActivityData = async (userId, accessToken) => {
2 try {
3 const response = await fetch(`https://api.fitnessprovider.com/v1/users/${userId}/activities`, {
4 method: 'GET',
5 headers: {
6 'Authorization': `Bearer ${accessToken}`,
7 'Content-Type': 'application/json'
8 }
9 });
10
11 if (!response.ok) {
12 throw new Error(`HTTP error! Status: ${response.status}`);
13 }
14
15 const data = await response.json();
16 return data;
17 } catch (error) {
18 console.error('Error fetching activity data:', error);
19 throw error;
20 }
21};
22
Handling API Responses
Fitness APIs typically return data in JSON (JavaScript Object Notation) or sometimes XML format. Proper handling of these responses includes:
- Parsing the response data into usable objects
- Validating the data to ensure it meets your application's requirements
- Implementing error handling to gracefully manage API failures
Example of parsing a JSON response:
1const processActivityData = (activityData) => {
2 try {
3 // Extract relevant metrics
4 const {
5 date,
6 steps,
7 distance,
8 activeMinutes,
9 caloriesBurned
10 } = activityData;
11
12 // Validate data
13 if (!steps || !distance || !caloriesBurned) {
14 console.warn('Missing essential activity metrics');
15 }
16
17 // Process and return the formatted data
18 return {
19 date: new Date(date),
20 metrics: {
21 steps: Number(steps),
22 distance: Number(distance),
23 activeMinutes: Number(activeMinutes),
24 caloriesBurned: Number(caloriesBurned)
25 }
26 };
27 } catch (error) {
28 console.error('Error processing activity data:', error);
29 throw error;
30 }
31};
32
Navigating the Deprecation of Google Fit REST API & Health Connect
The fitness API landscape is evolving, with significant changes like Google's decision to deprecate its Google Fit REST API. This transition represents both a challenge and an opportunity for developers in the fitness app space.
The End of an Era
Google officially announced the deprecation of the Google Fit REST API, marking a significant shift in how developers interact with fitness data on Android devices. This change requires developers who relied on this API to adapt their strategies and explore alternative solutions.
Understanding Health Connect
As the intended replacement for Google Fit REST API, Health Connect offers a new approach to fitness data integration on Android. Health Connect aims to provide a more unified and privacy-focused platform for health and fitness data, with improved interoperability between different apps and services.
Migration Strategies
Developers looking to transition from Google Fit REST API to Health Connect can find comprehensive resources and guides to facilitate the migration process. Google provides official documentation on making this transition as smooth as possible.
Comparing Options
When evaluating alternatives to Google Fit REST API, developers should consider the relative strengths of options like Health Connect and Fitbit Web APIs. Each offers different capabilities, integration options, and ecosystems that may better align with specific app requirements and user bases.
Challenges and Considerations
Data Privacy and Security
Handling fitness data comes with significant responsibilities regarding user privacy. Developers must ensure HIPAA compliance where applicable and implement robust security measures to protect sensitive health information. This includes secure data transmission, proper storage practices, and transparent privacy policies.
Data Accuracy and Reliability
Fitness data collected from various sensors and devices may contain inaccuracies that can affect the reliability of insights and recommendations. Implementing validation mechanisms and calibration options can help mitigate these issues and provide users with more trustworthy information.
API Rate Limits and Throttling
Most fitness APIs impose usage limits to prevent overloading their systems. Developers need to design their applications with these constraints in mind, implementing strategies like caching, batching requests, and graceful degradation to handle rate limiting situations effectively.
Key Takeaways
Fitness APIs serve as powerful tools for developers looking to create innovative health and wellness applications. By leveraging these interfaces, developers can access rich fitness data, integrate with popular devices and platforms, and deliver personalized experiences to users.
The landscape of fitness APIs continues to evolve, as evidenced by developments like the Google Fit API deprecation and the emergence of Health Connect. Staying informed about these changes and maintaining flexibility in implementation approaches is essential for long-term success.
Careful planning and implementation, with particular attention to data privacy, accuracy, and API limitations, will help ensure that fitness applications built using these APIs deliver genuine value to users while respecting their trust and privacy.
Conclusion
The integration of fitness APIs represents a significant opportunity for developers to create impactful applications that genuinely improve users' health and wellness journeys. As the fitness technology ecosystem continues to expand and evolve, these APIs will play an increasingly important role in connecting disparate systems and providing users with holistic insights into their fitness data.
We encourage developers to explore the diverse range of fitness APIs available and consider how they might leverage these powerful tools to create the next generation of innovative fitness applications. Whether you're building a specialized workout tracker, a comprehensive health platform, or a social fitness community, the right API integrations can elevate your application and deliver exceptional value to your users.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ