Picture this: your users are trying to watch a video on your Android app while commuting, but as they move from Wi-Fi to spotty cellular coverage, their video keeps buffering and switching between high and low quality. Frustrating, right? This is the exact problem that HTTP Live Streaming (HLS) technology solves, and implementing a robust HLS player in your Android application can dramatically improve the streaming experience for your users.
In this comprehensive guide, we'll explore everything you need to know about implementing HLS playback on Android – from native options built into the Android framework to third-party libraries and open-source GitHub projects. Whether you're building a video streaming app from scratch or enhancing an existing application, you'll learn how to deliver a seamless, buffer-free video experience across the diverse landscape of Android devices and network conditions.
What is HLS and Why Use It on Android?
Understanding HLS Technology
HTTP Live Streaming (HLS) is an adaptive bitrate streaming protocol developed by Apple that has become the industry standard for video delivery. Unlike traditional video formats that require downloading an entire file or establishing specialized streaming connections, HLS works by:
- Breaking videos into small segments (typically 2-10 seconds each)
- Creating multiple versions of these segments at different quality levels
- Using standard HTTP to deliver the content
- Dynamically switching between quality levels based on available bandwidth
This approach is particularly valuable on mobile devices like Android phones and tablets, where network conditions can change rapidly as users move around.
Benefits of HLS on Android Devices
Implementing HLS playback on your Android application offers numerous advantages:
- Adaptive Quality: Automatically adjusts video quality based on network conditions, preventing buffering while maximizing quality
- Wide Compatibility: Works across virtually all Android versions and device types
- Efficient Bandwidth Usage: Only uses the bandwidth needed for the current network conditions
- Better Battery Life: Less processing power is required for smooth playback
- Reliable Delivery: Standard HTTP delivery means better compatibility with firewalls, proxies, and CDNs
- Built-in Support: Recent Android versions have native support for HLS playback
HLS Workflow Explained
Here's how HLS works behind the scenes:
- Content Preparation: Your source video (typically MP4 or MOV) is encoded into multiple renditions at different bitrates and resolutions.
- Segmentation: Each rendition is divided into small chunks (usually .ts files).
- Playlist Creation:
- A media playlist (.m3u8 file) is created for each quality level, listing its segments
- A master playlist (.m3u8) lists all available quality levels
- Delivery: The segments and playlists are hosted on a standard HTTP server.
- Playback: The HLS player on the Android device:
- Requests the master playlist
- Selects the appropriate quality level based on current conditions
- Downloads segments sequentially for playback
- Continuously monitors network conditions to switch quality levels as needed
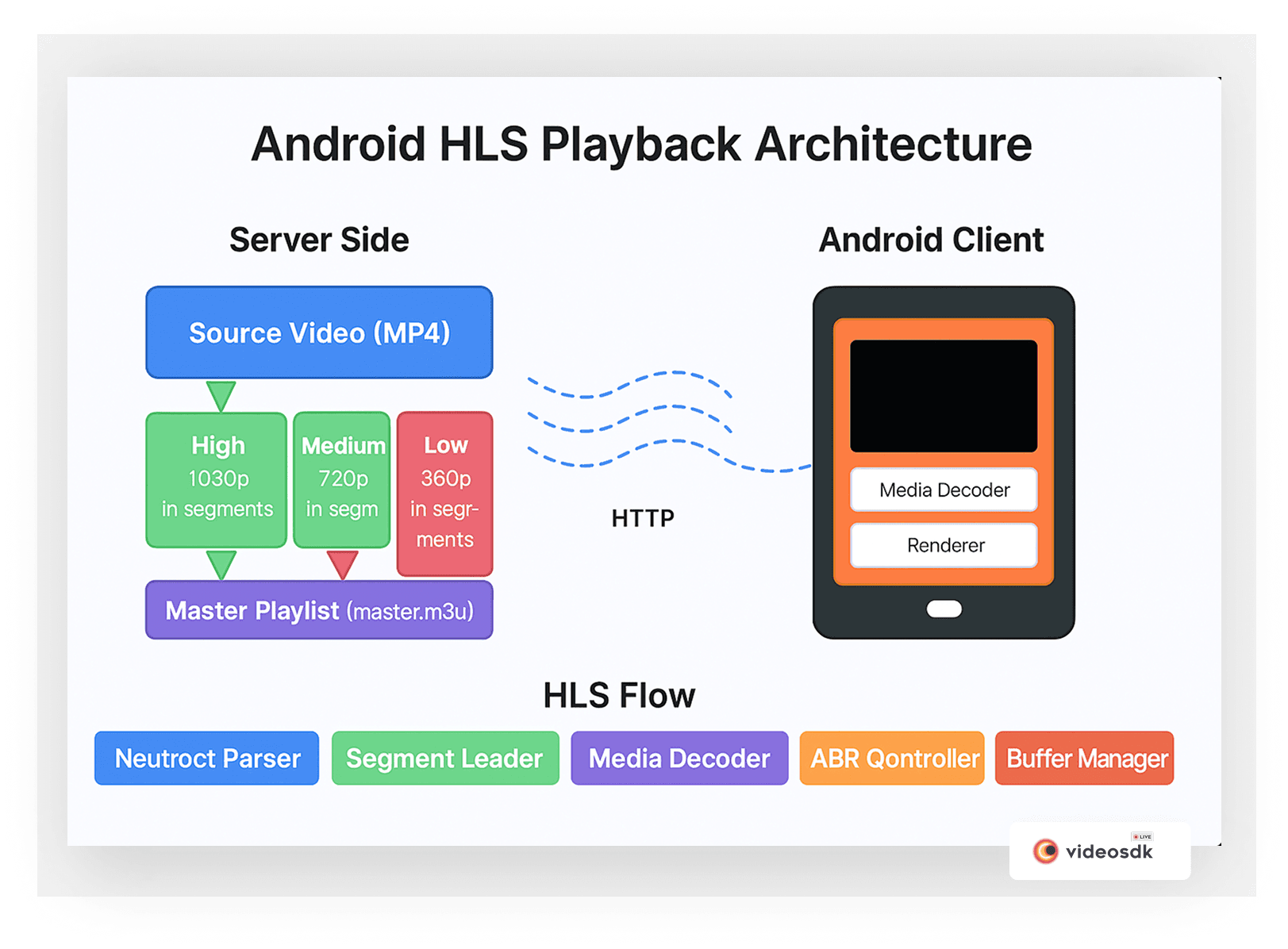
Native Android HLS Playback Options
Android offers several built-in options for implementing HLS playback, with ExoPlayer being the most robust and widely used solution.
ExoPlayer: Google's Recommended Media Player
ExoPlayer is an open-source media player developed by Google specifically for Android. It's designed to be highly customizable and capable of handling complex streaming formats, including HLS. Since its introduction, it has become the de facto standard for video playback on Android.
Key features of ExoPlayer for HLS playback:
- Native support for HLS adaptive streaming
- Smooth quality transitions without interrupting playback
- Support for live streams and video-on-demand (VOD)
- Customizable buffering behavior
- Advanced features like DRM, subtitles, and multiple audio tracks
- Consistent API across different Android versions
- Regular updates and active maintenance by Google
Basic ExoPlayer implementation for HLS:
1// Step 1: Add ExoPlayer dependency to your build.gradle
2// implementation 'com.google.android.exoplayer:exoplayer:2.X.X'
3
4// Step 2: Create a player instance and connect it to your view
5public class PlayerActivity extends AppCompatActivity {
6
7 private SimpleExoPlayer player;
8 private PlayerView playerView;
9
10 @Override
11 protected void onCreate(Bundle savedInstanceState) {
12 super.onCreate(savedInstanceState);
13 setContentView(R.layout.activity_player);
14
15 playerView = findViewById(R.id.player_view);
16
17 // Initialize the player
18 player = new SimpleExoPlayer.Builder(this).build();
19
20 // Attach player to the view
21 playerView.setPlayer(player);
22
23 // Build the media source for HLS
24 String hlsUrl = "https://example.com/playlist.m3u8";
25 MediaSource mediaSource = new HlsMediaSource.Factory(
26 new DefaultHttpDataSourceFactory("user-agent"))
27 .createMediaSource(Uri.parse(hlsUrl));
28
29 // Prepare the player with the source
30 player.setMediaSource(mediaSource);
31 player.prepare();
32
33 // Start playback when ready
34 player.setPlayWhenReady(true);
35 }
36
37 @Override
38 protected void onPause() {
39 super.onPause();
40 if (player != null) {
41 player.setPlayWhenReady(false);
42 }
43 }
44
45 @Override
46 protected void onDestroy() {
47 super.onDestroy();
48 if (player != null) {
49 player.release();
50 player = null;
51 }
52 }
53}
54
With the more recent versions of ExoPlayer, the implementation has been simplified even further with the newer Media3 library:
1// Using Media3 (the newer version of ExoPlayer)
2// Add dependencies:
3// implementation 'androidx.media3:media3-exoplayer:1.X.X'
4// implementation 'androidx.media3:media3-exoplayer-hls:1.X.X'
5// implementation 'androidx.media3:media3-ui:1.X.X'
6
7class PlayerActivity : AppCompatActivity() {
8
9 private var player: ExoPlayer? = null
10
11 override fun onCreate(savedInstanceState: Bundle?) {
12 super.onCreate(savedInstanceState)
13 setContentView(R.layout.activity_player)
14
15 val playerView = findViewById<PlayerView>(R.id.player_view)
16
17 player = ExoPlayer.Builder(this).build()
18 playerView.player = player
19
20 val mediaItem = MediaItem.fromUri("https://example.com/playlist.m3u8")
21 player?.setMediaItem(mediaItem)
22 player?.prepare()
23 player?.playWhenReady = true
24 }
25
26 override fun onPause() {
27 super.onPause()
28 player?.playWhenReady = false
29 }
30
31 override fun onDestroy() {
32 super.onDestroy()
33 player?.release()
34 player = null
35 }
36}
37
Using MediaPlayer for Basic HLS Playback
Before ExoPlayer, Android's built-in
MediaPlayer
class was the go-to option for media playback. While it does support HLS on newer Android versions (4.0+), it has significant limitations compared to ExoPlayer:1MediaPlayer mediaPlayer = new MediaPlayer();
2mediaPlayer.setDataSource("https://example.com/playlist.m3u8");
3mediaPlayer.setOnPreparedListener(mp -> mp.start());
4mediaPlayer.prepareAsync();
5
Limitations of MediaPlayer for HLS:
- Limited control over adaptive streaming behavior
- Inconsistent HLS support across Android versions
- Fewer customization options for buffering and error handling
- Lack of advanced features like DRM and subtitles
- Less reliable playback for complex streams
When to use MediaPlayer for HLS:
- For simple playback needs on newer Android devices
- When minimizing dependencies is a priority
- In apps where video playback is not a central feature
In most cases, ExoPlayer is the recommended choice due to its superior HLS handling and consistent behavior across Android versions.
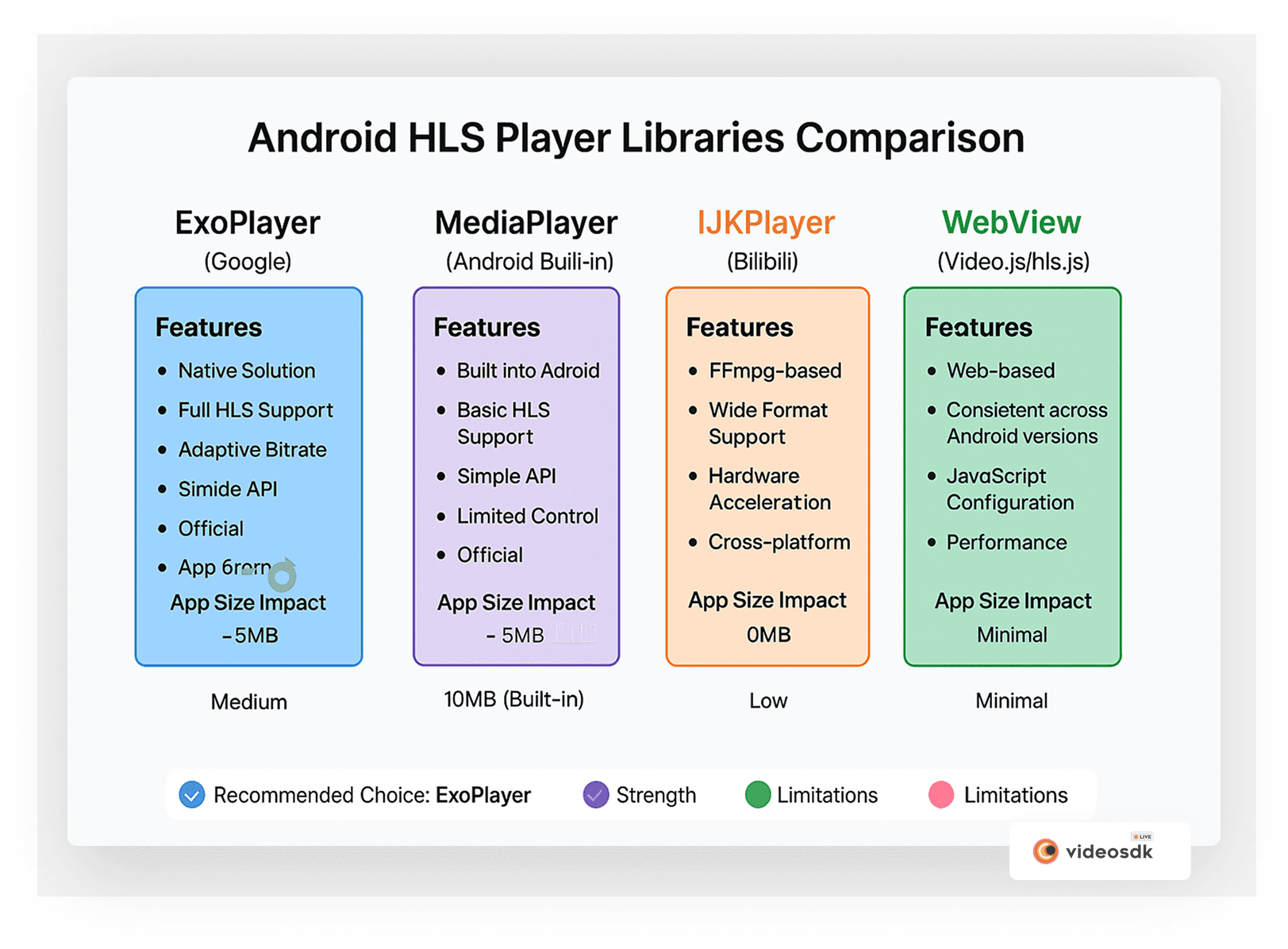
Exploring Android HLS Player Libraries
While ExoPlayer covers most HLS playback needs, several third-party libraries offer alternative approaches or specialized features.
IJKPlayer: A Powerful Alternative
IJKPlayer is a popular open-source media player based on FFmpeg. Developed by Bilibili, it offers strong HLS support with optimizations for mobile devices.
Key features:
- Based on FFmpeg, providing extensive format support
- Hardware acceleration on supported devices
- Customizable timeouts and buffering behavior
- Support for various protocols beyond HLS
- Active community and regular updates
Basic implementation:
1// Add dependencies and import statements
2
3IjkMediaPlayer player = new IjkMediaPlayer();
4player.setOption(IjkMediaPlayer.OPT_CATEGORY_PLAYER, "framedrop", 1);
5player.setOption(IjkMediaPlayer.OPT_CATEGORY_FORMAT, "http-detect-range-support", 0);
6player.setOption(IjkMediaPlayer.OPT_CATEGORY_CODEC, "skip_loop_filter", 48);
7
8try {
9 player.setDataSource("https://example.com/playlist.m3u8");
10 player.prepareAsync();
11 player.setOnPreparedListener(IMediaPlayer::start);
12} catch (IOException e) {
13 e.printStackTrace();
14}
15
Video.js in WebView: A Web-Based Approach
For developers already familiar with web technologies, using Video.js within a WebView can provide a flexible HLS playback solution:
1WebView webView = findViewById(R.id.webview);
2webView.getSettings().setJavaScriptEnabled(true);
3webView.setWebChromeClient(new WebChromeClient());
4
5String html = "<!DOCTYPE html><html><head>" +
6 "<meta name='viewport' content='width=device-width, initial-scale=1.0'>" +
7 "<link href='https://vjs.zencdn.net/7.15.4/video-js.css' rel='stylesheet'>" +
8 "<script src='https://vjs.zencdn.net/7.15.4/video.min.js'></script>" +
9 "</head><body>" +
10 "<video-js id='player' class='vjs-default-skin' controls preload='auto' width='100%' height='100%'>" +
11 "<source src='https://example.com/playlist.m3u8' type='application/x-mpegURL'>" +
12 "</video-js>" +
13 "<script>" +
14 "var player = videojs('player');" +
15 "player.play();" +
16 "</script>" +
17 "</body></html>";
18
19webView.loadData(html, "text/html", "UTF-8");
20
Advantages of the WebView approach:
- Consistent playback behavior across different Android versions
- Leverage web-based HLS players with mature features
- Simplify integration if your app already uses web components
- Easy implementation of custom controls via JavaScript
Disadvantages:
- Higher memory usage compared to native solutions
- Potential performance issues on lower-end devices
- Limited integration with Android's media systems
- More complex interface between JavaScript and native code
Library Comparison
Here's a comparison of the major Android HLS player options:
1Feature ExoPlayer MediaPlayer IJKPlayer WebView + Video.js
2-----------------------------------------------------------------------------
3Native Android Yes (Google) Yes (Built-in) No No
4library
5
6HLS support Excellent Basic Very good Good
7quality
8
9Customization Extensive Limited Good Via JavaScript
10
11Performance Excellent Good Very good Moderate
12
13Memory usage Low Lowest Moderate Highest
14
15Advanced Extensive Limited Moderate Via plugins
16features (DRM,
17subtitles)
18
19Maintenance Active (Google) Part of Android Community Active web library
20
21Implementation Moderate Low High Moderate
22complexity
23
24App size impact ~2 MB 0 (built-in) ~8 MB Minimal
25 (with FFmpeg)
26
Finding Android HLS Player GitHub Options
The open-source community provides several valuable GitHub repositories for HLS playback on Android. Here are some of the most active and well-maintained projects:
1. Google's ExoPlayer
GitHub Repository:
google/ExoPlayer
ExoPlayer is the official Google implementation and the most actively maintained Android HLS player on GitHub. It's regularly updated with new features and bug fixes.
Key features:
- Comprehensive HLS support
- Clear documentation and examples
- Regular releases
- Apache 2.0 license
- Extensive community support
Since the transition to Media3, the main repository is now:
GitHub Repository:
androidx/media
2. Bilibili's IJKPlayer
GitHub Repository:
bilibili/ijkplayer
IJKPlayer is a powerful media player based on FFmpeg developed by the popular Chinese video platform Bilibili.
Key features:
- Extensive format support via FFmpeg
- Hardware acceleration
- Cross-platform (Android and iOS)
- LGPL/GPL license
- Active community
3. JWPlayer Android SDK (Commercial with GitHub Examples)
GitHub Repository:
jwplayer/jwplayer-android-best-practice-apps
While the JWPlayer SDK itself is commercial, their GitHub repository provides valuable example code and best practices for implementing professional video experiences on Android.
Key features:
- Enterprise-grade HLS playback
- Analytics and monitoring
- Advertising integration
- Commercial license with GitHub examples
- Professional support
Evaluating GitHub Projects for Your Needs
When selecting an open-source HLS player for your Android project, consider these factors:
- Activity and maintenance: Check the frequency of commits and releases
- Issue resolution speed: See how quickly bugs are addressed
- Documentation quality: Look for clear setup instructions and examples
- License compatibility: Ensure the license works with your project
- Community size: A larger community means more resources and faster bug identification
- Code quality: Review the code organization and architecture
- Size and dependencies: Consider the impact on your app's size
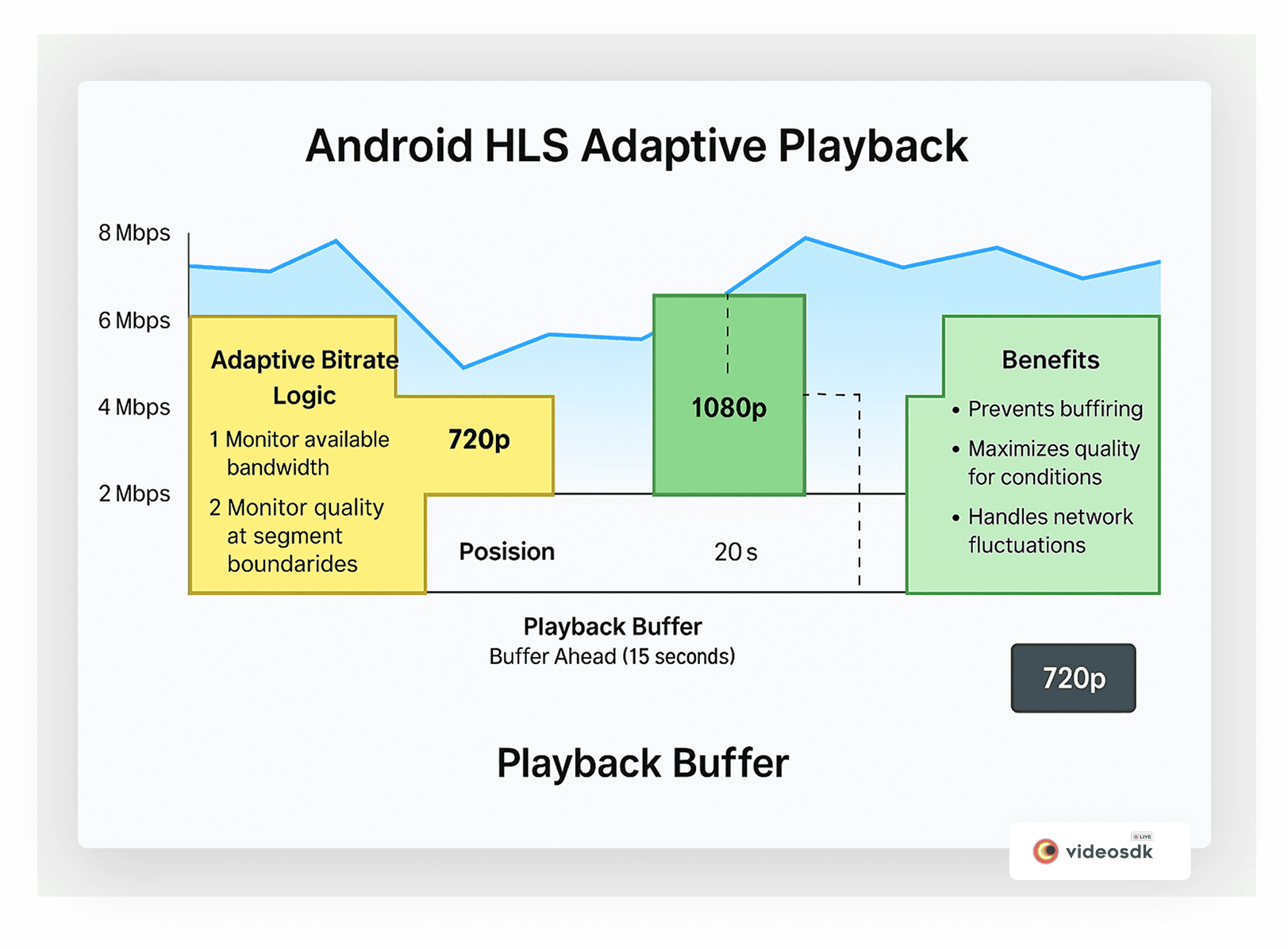
Optimizing HLS Playback on Android
Once you've selected and implemented an HLS player, you'll want to optimize it for the best user experience.
Video Streaming Optimization Guide
Adaptive Bitrate Configuration
For optimal adaptive streaming:
- Prepare multiple quality levels: Create renditions at different resolutions and bitrates.
1# Example FFmpeg command for multiple renditions 2ffmpeg -i input.mp4 \ 3 -vf scale=1920:1080 -c:v h264 -b:v 5000k -c:a aac -b:a 192k high.mp4 \ 4 -vf scale=1280:720 -c:v h264 -b:v 2500k -c:a aac -b:a 128k medium.mp4 \ 5 -vf scale=640:360 -c:v h264 -b:v 1000k -c:a aac -b:a 96k low.mp4 6
- Optimize segment length: 4-6 seconds is typically ideal (balancing quality switching speed vs. overhead)
1# Example HLS segmentation with 4-second segments 2ffmpeg -i input.mp4 -c:v h264 -c:a aac -f hls -hls_time 4 -hls_list_size 0 -hls_segment_filename "segment_%03d.ts" playlist.m3u8 3
- Configure appropriate starting quality: Set initial quality selection based on connection type
1// ExoPlayer example: set initial bitrate constraint 2DefaultTrackSelector trackSelector = new DefaultTrackSelector(context); 3trackSelector.setParameters( 4 trackSelector.buildUponParameters() 5 .setMaxVideoBitrate(getInitialBitrateForNetwork()) 6); 7 8ExoPlayer player = new ExoPlayer.Builder(context) 9 .setTrackSelector(trackSelector) 10 .build(); 11
Buffering Strategies
Effective buffering is crucial for smooth playback:
- Configure buffer sizes appropriately:
1// ExoPlayer example: customize buffering parameters 2LoadControl loadControl = new DefaultLoadControl.Builder() 3 .setBufferDurationsMs( 4 DefaultLoadControl.DEFAULT_MIN_BUFFER_MS, // Min buffer duration 5 30000, // Max buffer duration (30 seconds) 6 DefaultLoadControl.DEFAULT_BUFFER_FOR_PLAYBACK_MS, 7 DefaultLoadControl.DEFAULT_BUFFER_FOR_PLAYBACK_AFTER_REBUFFER_MS) 8 .build(); 9 10ExoPlayer player = new ExoPlayer.Builder(context) 11 .setLoadControl(loadControl) 12 .build(); 13
- Preload content when possible:
1// Preload the next video while the current one is playing 2player.addListener(new Player.Listener() { 3 @Override 4 public void onPositionDiscontinuity(Player.PositionInfo oldPosition, 5 Player.PositionInfo newPosition, 6 int reason) { 7 // Near the end of current video 8 if (player.getDuration() - player.getCurrentPosition() < 10000) { 9 // Prepare the next item in background 10 prepareNextVideo(); 11 } 12 } 13}); 14
- Handle network transitions gracefully:
1// Register a network callback to handle network changes 2ConnectivityManager connectivityManager = 3 (ConnectivityManager) context.getSystemService(Context.CONNECTIVITY_SERVICE); 4 5ConnectivityManager.NetworkCallback networkCallback = new ConnectivityManager.NetworkCallback() { 6 @Override 7 public void onCapabilitiesChanged(Network network, 8 NetworkCapabilities capabilities) { 9 // Adjust buffer strategy based on network capability 10 boolean isUnmetered = 11 capabilities.hasCapability(NetworkCapabilities.NET_CAPABILITY_NOT_METERED); 12 updateBufferStrategy(isUnmetered); 13 } 14}; 15 16NetworkRequest request = new NetworkRequest.Builder() 17 .addCapability(NetworkCapabilities.NET_CAPABILITY_INTERNET) 18 .build(); 19 20connectivityManager.registerNetworkCallback(request, networkCallback); 21
Error Handling and Monitoring
Robust error handling improves the user experience:
- Implement comprehensive error handling:
1player.addListener(new Player.Listener() { 2 @Override 3 public void onPlayerError(PlaybackException error) { 4 // Log error for analytics 5 logPlaybackError(error); 6 7 // Attempt recovery based on error type 8 if (error.errorCode == PlaybackException.ERROR_CODE_IO_NETWORK_CONNECTION_FAILED) { 9 // Show user-friendly message 10 showErrorMessage("Network connection lost. Retrying..."); 11 12 // Retry playback after delay 13 handler.postDelayed(() -> { 14 resetPlayer(); 15 prepareAndPlay(currentMediaItem); 16 }, 5000); 17 } else { 18 // Handle other error types 19 showErrorMessage("Playback error: " + getReadableErrorMessage(error)); 20 } 21 } 22}); 23
- Monitor playback quality:
1player.addAnalyticsListener(new AnalyticsListener() { 2 @Override 3 public void onBandwidthEstimate(EventTime eventTime, 4 int totalLoadTimeMs, 5 long totalBytesLoaded, 6 long bitrateEstimate) { 7 // Log bandwidth for analytics 8 logBandwidthSample(bitrateEstimate); 9 10 // Potentially adjust player settings based on bandwidth trends 11 analyzeBandwidthHistory(); 12 } 13 14 @Override 15 public void onDroppedVideoFrames(EventTime eventTime, 16 int droppedFrames, 17 long elapsedMs) { 18 // Log frame drops for performance monitoring 19 logFrameDrops(droppedFrames, elapsedMs); 20 21 // Consider reducing quality if frame drops are excessive 22 if (isFrameDropRateHigh(droppedFrames, elapsedMs)) { 23 reducePlaybackQuality(); 24 } 25 } 26}); 27
Performance Tuning
Optimize overall playback performance:
- Use hardware acceleration when available:
1// ExoPlayer selects hardware acceleration by default when available 2// You can force software rendering if needed: 3DefaultRenderersFactory renderersFactory = new DefaultRenderersFactory(context) 4 .setExtensionRendererMode(DefaultRenderersFactory.EXTENSION_RENDERER_MODE_OFF); 5 6ExoPlayer player = new ExoPlayer.Builder(context) 7 .setRenderersFactory(renderersFactory) 8 .build(); 9
- Optimize for battery life:
1// Release player resources when not visible 2@Override 3protected void onPause() { 4 super.onPause(); 5 if (Util.SDK_INT <= 23) { 6 releasePlayer(); 7 } 8} 9 10@Override 11protected void onStop() { 12 super.onStop(); 13 if (Util.SDK_INT > 23) { 14 releasePlayer(); 15 } 16} 17 18private void releasePlayer() { 19 if (player != null) { 20 player.release(); 21 player = null; 22 } 23} 24
- Consider the device's capabilities:
1private int getMaxSupportedResolution() { 2 // Check device capability to handle different resolutions 3 ActivityManager activityManager = 4 (ActivityManager) context.getSystemService(Context.ACTIVITY_SERVICE); 5 ActivityManager.MemoryInfo memoryInfo = new ActivityManager.MemoryInfo(); 6 activityManager.getMemoryInfo(memoryInfo); 7 8 // Low-end device detection 9 boolean isLowEndDevice = 10 memoryInfo.totalMem < 2L * 1024L * 1024L * 1024L; // < 2GB RAM 11 12 if (isLowEndDevice) { 13 return 720; // Cap at 720p for low-end devices 14 } else { 15 return 1080; // Allow up to 1080p for higher-end devices 16 } 17} 18
Key Takeaways
When implementing HLS playback on Android, remember these key points:
- ExoPlayer is the recommended solution for most Android HLS playback needs due to its comprehensive feature set, active maintenance by Google, and excellent performance
- Consider device and network diversity when configuring adaptive bitrate settings to ensure smooth playback across the Android ecosystem
- Open-source GitHub projects provide valuable resources, with ExoPlayer and IJKPlayer being the most prominent options
- Proper error handling and buffering strategies are critical for a positive user experience
- Optimize for battery life by properly managing player resources
Conclusion
Implementing HLS playback on Android has never been more accessible. With robust native options like ExoPlayer, powerful libraries, and active open-source projects, developers can deliver high-quality video streaming experiences that adapt to the challenging and diverse conditions of mobile networks.
As video continues to dominate mobile content consumption, mastering HLS implementation gives your Android applications a significant advantage. By using the techniques and tools outlined in this guide, you can create video experiences that keep users engaged regardless of their device or network conditions.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ