Choosing the Right Speech Recognition SDK: A Comprehensive Guide
Speech Recognition technology has revolutionized how we interact with machines. From virtual assistants like Siri and Alexa to transcription services and voice-controlled applications, the possibilities are vast. At the heart of these applications lies the speech recognition SDK, a powerful tool that enables developers to seamlessly integrate speech-to-text capabilities into their projects.
This comprehensive guide will delve into the world of Speech Recognition SDKs, exploring key factors to consider when choosing the right one, comparing top SDKs in the market, providing step-by-step integration instructions, and discussing advanced features and future trends. Whether you're building a mobile app, a web application, or an embedded system, this guide will equip you with the knowledge to make informed decisions and leverage the power of speech recognition.
Introduction to Speech Recognition SDKs
A speech recognition SDK (also referred to as a speech-to-text SDK, voice recognition SDK, or ASR SDK) is a software development kit that provides developers with the tools and resources necessary to add speech recognition functionality to their applications. These SDKs typically include libraries, APIs (speech recognition API, voice recognition API), documentation, and sample code, making it easier to integrate speech recognition into various platforms and devices. Common examples include the Microsoft Azure Speech SDK and the Google Cloud Speech-to-Text service.
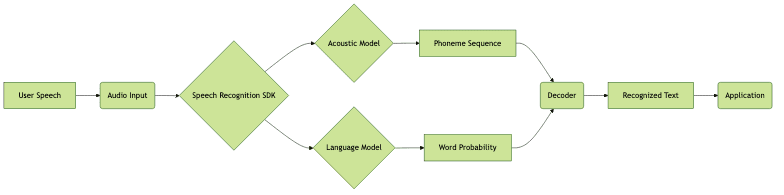
Key Factors to Consider
Choosing the right speech recognition SDK is crucial for the success of your project. Several factors should be considered, including:
- Accuracy: The accuracy of the speech recognition SDK is paramount. Look for SDKs that offer high accuracy rates, especially in noisy environments or with accented speech. Consider the specific use case and the required level of accuracy.
- Language Support: If your application needs to support multiple languages, ensure the speech recognition SDK offers robust multilingual speech recognition SDK support. Check for the availability of language models and the ease of adding new languages.
- Real-time vs. Offline Processing: Determine whether you need real-time speech recognition SDK capabilities for applications like live captioning or voice commands, or whether offline speech recognition SDK is sufficient for tasks like transcribing pre-recorded audio. Consider latency requirements.
- Customization: Some SDKs allow for custom speech recognition SDK development, enabling you to train custom models to improve accuracy for specific vocabularies or accents. This is particularly useful for niche applications or industries. Consider the level of customization offered.
- Pricing: Speech recognition SDK pricing varies significantly between providers. Evaluate the pricing model (e.g., pay-as-you-go, subscription-based) and choose an SDK that aligns with your budget and usage patterns.
- Platform Support: Verify that the speech recognition SDK supports the target platforms for your application, such as iOS speech recognition SDK, Android speech recognition SDK, web browsers (JavaScript speech recognition SDK), or embedded systems (speech recognition SDK for embedded systems).
- Ease of Integration: A well-documented and easy-to-integrate speech recognition SDK can save you significant development time. Look for SDKs with clear speech recognition SDK documentation and ample speech recognition SDK examples and speech recognition SDK tutorial resources. Consider the availability of libraries for your preferred programming languages (Python speech recognition SDK, Java speech recognition SDK, C# speech recognition SDK).
- Features: Look for features like speaker diarization (identifying who is speaking), punctuation insertion, and noise cancellation. Also evaluate the capability of the SDK to integrate with NLP SDK for downstream natural language processing tasks. Consider the speech recognition SDK features offered.
Top Speech Recognition SDKs Compared
Several excellent speech recognition SDKs are available, each with its strengths and weaknesses. Here's a comparison of some of the top speech recognition SDKs to help you compare speech recognition SDKs:
VideoSDK
VideoSDK offers a state-of-the-art speech recognition SDK featuring ultra-fast, accurate AI-powered transcription with support for over 100 languages and real-time live captioning-all with latency under 300 ms. Its hybrid on-device and cloud architecture delivers enterprise-grade security, speaker identification, and easy integration, making it ideal for scalable, real-time speech applications.
Microsoft Azure Speech SDK
The Microsoft Azure Speech SDK is a cloud-based service that offers a wide range of speech recognition capabilities, including speech-to-text, text-to-speech, and speech translation. It supports a variety of languages and offers high accuracy rates. It is known for its strong enterprise features and integrations with other Azure services.
Python
1import azure.cognitiveservices.speech as speechsdk
2
3speech_config = speechsdk.SpeechConfig(subscription="YOUR_SUBSCRIPTION_KEY", region="YOUR_REGION")
4audio_config = speechsdk.AudioConfig(filename="audio.wav")
5
6speech_recognizer = speechsdk.SpeechRecognizer(speech_config=speech_config, audio_config=audio_config)
7
8print("Recognizing...")
9
10result = speech_recognizer.recognize_once_async().get()
11
12if result.reason == speechsdk.ResultReason.RecognizedSpeech:
13 print("Recognized: {}".format(result.text))
14elif result.reason == speechsdk.ResultReason.NoMatch:
15 print("No speech could be recognized: {}".format(result.no_match_details))
16elif result.reason == speechsdk.ResultReason.Canceled:
17 cancellation_details = result.cancellation_details
18 print("Speech Recognition Canceled: {}".format(cancellation_details.reason))
19 if cancellation_details.reason == speechsdk.CancellationReason.Error:
20 print("Error details: {}".format(cancellation_details.error_details))
21
Google Cloud Speech-to-Text
Google Cloud Speech-to-Text is another powerful cloud-based speech-to-text SDK that leverages Google's advanced machine learning algorithms. It offers high accuracy, supports a wide range of languages, and provides features like automatic punctuation and speaker diarization. It also integrates seamlessly with other Google Cloud services.
Python
1from google.cloud import speech
2
3def transcribe_file(speech_file):
4 """Transcribe the given audio file."""
5 client = speech.SpeechClient()
6
7 with open(speech_file, "rb") as audio_file:
8 content = audio_file.read()
9
10 audio = speech.RecognitionAudio(content=content)
11 config = speech.RecognitionConfig(
12 encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16,
13 sample_rate_hertz=16000,
14 language_code="en-US",
15 )
16
17 response = client.recognize(config=config, audio=audio)
18
19 for result in response.results:
20 print("Transcript: {}".format(result.alternatives[0].transcript))
21
22transcribe_file("audio.wav")
23
Amazon Transcribe
Amazon Transcribe is a speech-to-text SDK offered by Amazon Web Services (AWS). It provides accurate and scalable speech recognition capabilities for a variety of use cases, including transcription of audio and video files, real-time transcription, and call center analytics. It integrates well with other AWS services. The tool allows you to create a custom speech recognition SDK.
AssemblyAI
AssemblyAI provides a speech recognition SDK designed for developers who need accurate transcription and powerful audio intelligence features. It's known for its ease of use and developer-friendly API. They offer features like entity detection, topic detection, and sentiment analysis alongside the core speech-to-text functionality.
Other Notable SDKs
Other notable speech recognition SDKs include Deepgram, Kaldi (an open-source toolkit), and CMU Sphinx. The best speech recognition SDK for you depends on your specific requirements and budget.
Integrating a Speech Recognition SDK into Your Application
Integrating a speech recognition SDK into your application typically involves the following steps:
Setting Up Your Development Environment
Before you begin, ensure that you have the necessary tools and libraries installed. This may include installing the SDK itself, setting up API keys, and configuring your development environment to work with the SDK. Follow the SDK's speech recognition SDK documentation for specific instructions.
Step-by-Step Integration Guide
- Install the SDK: Use the appropriate package manager or installation method to install the speech recognition SDK in your project. Example:
pip install azure-cognitiveservices-speech
- Authenticate: Obtain API keys or credentials from the speech recognition API provider and configure your application to authenticate with the service.
- Configure Speech Recognition: Create a
SpeechConfig
object (or equivalent) to specify the language, region, and other settings for speech recognition. For example, for Azure this configures language to use, region to connect to, and the Azure subscription information. - Create an Audio Configuration: Configure the audio input source, such as a microphone or an audio file. Use an
AudioConfig
(or equivalent) to specify these settings. You can specify the location of a .wav file, or set it to use the microphone as input. - Create a Speech Recognizer: Instantiate a
SpeechRecognizer
object (or equivalent) using the configuredSpeechConfig
andAudioConfig
objects. - Start Speech Recognition: Call the appropriate method to start speech recognition. This may involve calling
recognize_once_async()
for single utterances orstart_continuous_recognition_async()
for continuous speech recognition. - Handle Results: Implement event handlers or callbacks to process the results of speech recognition. Extract the recognized text from the results and use it in your application.
- Error Handling: Implement error handling to catch and handle any errors that may occur during speech recognition. Check for network connectivity issues, invalid API keys, or other potential problems.
Python
1# This is a generic example and will need adaptation for the specific SDK.
2
3# Replace with your actual SDK import
4#import myspeechsdk as speechsdk
5
6# Replace with your actual API key and region
7API_KEY = "YOUR_API_KEY"
8REGION = "YOUR_REGION"
9
10# Replace with your actual audio file path
11AUDIO_FILE = "audio.wav"
12
13try:
14 # Initialize speech recognition engine
15 #engine = speechsdk.SpeechRecognitionEngine(API_KEY, REGION)
16
17 # Configure audio source
18 #engine.set_audio_source(AUDIO_FILE)
19
20 # Start recognition
21 #result = engine.recognize()
22
23 # Print the result
24 #print(f"Recognized text: {result.text}")
25 print("Please note: This is a placeholder for actual code integration")
26
27except Exception as e:
28 print(f"Error during speech recognition: {e}")
29
Handling Errors and Debugging
When integrating a speech recognition SDK, it's important to implement robust error handling to gracefully handle potential issues. Common errors include network connectivity problems, invalid API keys, and incorrect audio formats. Use logging and debugging tools to identify and resolve these issues. Check for API rate limits and handle them gracefully.
Optimizing Performance
To optimize the performance of your speech recognition SDK integration, consider the following tips:
- Use appropriate audio formats: Choose audio formats that are optimized for speech recognition, such as WAV or FLAC.
- Reduce background noise: Minimize background noise in the audio input to improve accuracy.
- Use appropriate sample rates: Use appropriate sample rates for speech recognition, typically 16 kHz or 44.1 kHz.
- Cache results: Cache frequently accessed speech recognition results to reduce latency and improve performance.
Advanced Features and Customization
Many speech recognition SDKs offer advanced features and customization options to enhance the accuracy and functionality of your applications.
Custom Speech Models
Custom speech models allow you to train the speech recognition SDK on specific vocabularies or accents, improving accuracy for niche applications or industries. This is particularly useful for applications that involve specialized terminology or require recognition of unique accents. This option can greatly improve the accuracy of speech recognition SDKs.
Language Support and Localization
Ensure that the speech recognition SDK supports the languages and regions you need to target. Some SDKs offer advanced localization features, such as automatic language detection and regional accent adaptation. If you are looking for a multilingual speech recognition SDK, pay attention to which locales the SDK has strong models for.
Real-time vs. Offline Processing
Choose the appropriate processing mode based on your application's requirements. Real-time speech recognition SDK is suitable for applications that require immediate transcription or voice commands, while offline speech recognition SDK is ideal for transcribing pre-recorded audio files. Pay attention to the limitations of speech recognition SDKs, such as latency in real-time processing.
Future Trends in Speech Recognition SDKs
The future of speech recognition SDKs is promising, with ongoing advancements in machine learning and artificial intelligence. Some key trends include:
- Improved Accuracy: Continued improvements in machine learning algorithms are leading to more accurate and robust speech recognition capabilities.
- Enhanced Customization: SDKs are offering more advanced customization options, allowing developers to fine-tune speech recognition models for specific use cases.
- Edge Computing: The rise of edge computing is enabling on-device speech recognition SDK for applications that require low latency or operate in offline environments (offline speech recognition SDK).
- Integration with AI: Speech recognition SDKs are increasingly being integrated with other AI technologies, such as natural language processing (NLP) and machine translation, to create more intelligent and versatile applications.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ