Understanding Pipecat and the Need for Alternatives
What is Pipecat?
Pipecat is a command-line tool designed to pipe data between processes, similar to how Unix pipes work. It aims to provide a simple and efficient way to redirect standard input/output streams, allowing for chained operations and data manipulation. It's often used for tasks like logging, data transformation, and process orchestration.
Limitations of Pipecat
While Pipecat offers basic piping functionality, it has limitations. Its performance can degrade with large datasets or complex pipelines. It may lack advanced features such as error handling, data transformation capabilities, or robust support for different data formats. Additionally, Pipecat might not be suitable for high-concurrency scenarios or distributed systems due to its relatively simple architecture. Furthermore, issues with cross-platform compatibility or maintenance can also arise, making users seek alternatives.
Why You Might Need an Alternative
Several reasons might drive the need for a Pipecat alternative. Scalability issues with large data volumes, lack of advanced data processing features, limited error handling, or the need for more robust concurrency support are common motivations. Users might also require better cross-platform compatibility or find that Pipecat lacks integration with other tools in their workflow. The need for more sophisticated inter-process communication mechanisms or enhanced security features can also prompt the search for alternatives to Pipecat.
Top 5 Pipecat Alternatives
VideoSDK
Videosdk offers highly customizable UI kits and prebuilt templates for rapid integration.
- Features such as chat, polls, Q&A, whiteboard, cloud recording, adaptive audio/video quality, and interactive live streaming.
- Support for large-scale events (up to millions of participants), cross-platform compat
Alternative 1: GNU Parallel
- GNU Parallel is a command-line tool that allows you to execute commands in parallel. It's especially useful when you need to process multiple inputs independently and quickly. It significantly speeds up tasks that would otherwise be performed sequentially, making it a powerful Pipecat alternative.
Alternative 2: Apache Kafka
Apache Kafka is a distributed streaming platform capable of handling real-time data feeds. It's designed for high-throughput, fault-tolerant data pipelines. Kafka is a much more robust and scalable solution compared to Pipecat, especially for handling large data streams in a distributed environment. Kafka is well suited as a message queue alternative.
python
1from kafka import KafkaProducer
2
3producer = KafkaProducer(bootstrap_servers='localhost:9092')
4producer.send('my-topic', b'my message')
5producer.flush()
6
This Python snippet shows how to send a message to a Kafka topic using the Kafka Python client.
RabbitMQ
RabbitMQ is a message broker that implements the Advanced Message Queuing Protocol (AMQP). It provides a reliable and flexible platform for message exchange between applications. Compared to Pipecat, RabbitMQ offers more sophisticated message routing, queuing, and delivery guarantees.
python
1import pika
2
3connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
4channel = connection.channel()
5channel.queue_declare(queue='hello')
6channel.basic_publish(exchange='', routing_key='hello', body='Hello World!')
7connection.close()
8
This Python code sends a "Hello World!" message to a RabbitMQ queue.
ZeroMQ
ZeroMQ (also known as ØMQ or 0MQ) is a high-performance asynchronous messaging library. It provides a socket-based API for various messaging patterns, including publish-subscribe, request-reply, and pipeline. ZeroMQ is ideal for building scalable and distributed applications where low latency and high throughput are critical.
python
1import zmq
2
3context = zmq.Context()
4socket = context.socket(zmq.PUB)
5socket.bind("tcp://*:5555")
6
7while True:
8 socket.send_string("Hello, world!")
9
This Python example creates a ZeroMQ publisher socket and sends messages.
Celery
Celery is a distributed task queue. It's used to asynchronously execute tasks outside the main application thread. Celery is a robust and scalable solution for handling background tasks and long-running processes. It is a good alternative for standard input/output redirection alternatives and process communication alternatives.
python
1from celery import Celery
2
3app = Celery('tasks', broker='redis://localhost:6379/0')
4
5@app.task
6def add(x, y):
7 return x + y
8
9result = add.delay(4, 4)
10print(result.get())
11
This Python code defines a Celery task
add
and executes it asynchronously.Choosing the Right Pipecat Alternative: A Comparative Analysis
Key Features to Consider
When selecting a Pipecat alternative, consider features like scalability, performance, data transformation capabilities, error handling, concurrency support, cross-platform compatibility, security, and integration with your existing infrastructure. Also, assess the ease of use, documentation quality, and community support for each tool.
Comparison Table: Top 5 Alternatives
Feature | GNU Parallel | Apache Kafka | RabbitMQ | ZeroMQ | Celery |
---|---|---|---|---|---|
Scalability | Limited | High | Medium | High | High |
Performance | Good | Excellent | Good | Excellent | Good |
Data Transform | Basic | Limited | None | None | Limited |
Error Handling | Basic | Robust | Robust | Basic | Robust |
Concurrency | High | High | Medium | High | High |
Cross-Platform | Yes | Yes | Yes | Yes | Yes |
Security | Basic | Medium | Medium | Basic | Medium |
Ease of Use | Medium | Medium | Medium | Medium | Medium |
Factors Influencing Your Choice
The best Pipecat alternative depends on your specific needs. If you require simple parallel execution of commands, GNU Parallel might suffice. For high-throughput data streaming, Apache Kafka is a strong contender. RabbitMQ is suitable for reliable message queuing. ZeroMQ is ideal for building low-latency distributed applications. Celery excels at handling asynchronous tasks. Consider your data volume, performance requirements, and integration needs to make an informed decision. For efficient data transfer methods, consider alternatives that suits your specific needs.
Advanced Techniques for Data Piping and Inter-Process Communication
Using Named Pipes (FIFOs)
Named pipes, also known as FIFOs (First-In, First-Out), are a form of inter-process communication that allows unrelated processes to exchange data. Unlike regular pipes, named pipes exist as files in the file system, enabling communication between processes running independently. This is a Unix pipe alternative that is more advanced than basic pipes.
python
1import os
2import time
3
4fifo_path = '/tmp/my_fifo'
5
6# Create the FIFO if it doesn't exist
7if not os.path.exists(fifo_path):
8 os.mkfifo(fifo_path)
9
10# Producer process
11with open(fifo_path, 'w') as fifo:
12 message = "Hello from producer!"
13 fifo.write(message)
14 print(f"Producer sent: {message}")
15
16# Consumer process (in a separate terminal)
17# with open(fifo_path, 'r') as fifo:
18# message = fifo.read()
19# print(f"Consumer received: {message}")
20
The python example provides one part of the producer process, to use FIFOs, you need to run the producer process in one terminal and the consumer process (commented out) in another. Ensure to adjust permissions as needed.
Message Queues for Robust Communication
Message queues provide a robust and reliable mechanism for inter-process communication. They decouple the sender and receiver, ensuring that messages are delivered even if the receiver is temporarily unavailable. Message queues support various messaging patterns, including point-to-point and publish-subscribe. Kafka and RabbitMQ are examples of message queue systems.
python
1import redis
2
3r = redis.Redis(host='localhost', port=6379, db=0)
4queue_name = 'my_queue'
5
6# Producer
7r.lpush(queue_name, 'Message 1')
8r.lpush(queue_name, 'Message 2')
9
10# Consumer
11message1 = r.rpop(queue_name)
12message2 = r.rpop(queue_name)
13
14if message1:
15 print(f"Received: {message1.decode('utf-8')}")
16if message2:
17 print(f"Received: {message2.decode('utf-8')}")
18
This example uses Redis as a simple message queue.
Other IPC Mechanisms
Besides named pipes and message queues, other inter-process communication (IPC) mechanisms include shared memory, sockets, and signals. Shared memory allows processes to directly access a common memory region, enabling fast data exchange. Sockets provide a versatile interface for network communication. Signals are used to notify processes of specific events. These inter-process communication tools each have trade offs.
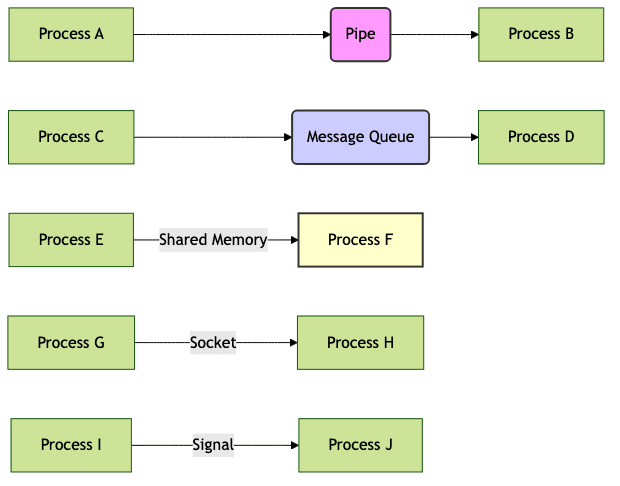
Security Considerations when Using Piping and Inter-Process Communication
Protecting Against Injection Attacks
When using piping and IPC, it's crucial to protect against injection attacks. Malicious actors might inject commands or data into the pipeline to compromise the system. Input validation and sanitization are essential to prevent such attacks. Properly escape or filter any data that passes between processes to ensure only safe data is used. Avoid constructing commands dynamically from user-provided input.
Data Integrity and Authentication
Ensuring data integrity and authentication is paramount in secure IPC. Use cryptographic techniques such as hashing and digital signatures to verify the authenticity and integrity of messages exchanged between processes. This helps prevent tampering and ensures that data originates from a trusted source. Employ secure communication protocols to protect data in transit.
Access Control and Authorization
Implement strict access control and authorization mechanisms to limit which processes can communicate with each other. Use appropriate permissions and authentication to verify the identity of processes attempting to establish communication. Enforce the principle of least privilege to grant processes only the necessary permissions.
Conclusion
Choosing the right Pipecat alternative depends on your specific requirements. Consider factors like scalability, performance, and security. Advanced techniques such as named pipes and message queues offer robust solutions for inter-process communication. Always prioritize security to protect against potential threats. By carefully evaluating your needs and implementing appropriate security measures, you can build efficient and secure data pipelines.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ