Introduction: The Rise of Live Voice Chat
In today's fast-paced digital world, instant communication is not just a luxury; it's a necessity. Live voice chat has emerged as a critical component of online interactions, offering a seamless and immediate way to connect with others. Whether it's for customer support, online gaming, or collaborative work, the ability to have real-time voice chat is transforming how we communicate and interact online. The demand for live audio chat and robust voice communication software is steadily increasing as businesses and individuals alike seek more engaging and efficient communication methods.
What is Live Voice Chat?
Live voice chat is a technology that enables users to communicate with each other in real-time using their voices over the internet. It utilizes Voice over Internet Protocol (VoIP) or technologies like WebRTC to transmit audio data, providing a dynamic and interactive communication experience. Unlike traditional phone calls, online voice chat solutions are often integrated directly into websites and applications, offering a convenient and accessible way to connect.
Why is Live Voice Chat Important?
Live voice chat offers numerous benefits over text-based communication, including faster response times, improved clarity, and a more personal connection. For businesses, this translates to enhanced customer satisfaction and improved operational efficiency. For individuals, it means more engaging social interactions and collaborative experiences. The importance of instant voice chat is further amplified by the growing remote work culture, where real-time communication tools are essential for maintaining productivity and team cohesion. This technology has revolutionized how we approach voice chat for business.
Types of Live Voice Chat
The landscape of live voice chat solutions is diverse, with various types of platforms and technologies catering to different needs and use cases. Understanding the different types of voice chat software available is crucial for choosing the right solution for your specific requirements.
Web-Based vs. App-Based Live Voice Chat
Web-based voice chat operates directly within a web browser, eliminating the need for users to download and install separate applications. This makes it highly accessible and convenient, as users can initiate live voice chat sessions with just a few clicks. WebRTC technology often powers these solutions, offering excellent audio quality and low latency. On the other hand, live voice chat apps offer a more dedicated and feature-rich experience, often providing better performance and integration with mobile devices. However, they require users to download and install the application, which can be a barrier to entry.
Peer-to-Peer vs. Server-Based Live Voice Chat
In peer-to-peer (P2P) voice chat, audio data is transmitted directly between users' devices without passing through a central server. This can result in lower latency and reduced bandwidth consumption, but it also presents scalability and security challenges. P2P solutions are typically suitable for small-group conversations. In contrast, server-based voice chat routes audio data through a central server, which manages connections, enforces security policies, and handles scalability. This approach is more robust and reliable for larger groups and offers better control over the communication environment.
Open Source vs. Proprietary Live Voice Chat Solutions
Open-source voice chat solutions offer developers the flexibility to customize and extend the platform to meet their specific needs. They also provide greater transparency and control over security. However, they often require more technical expertise to set up and maintain. Proprietary live voice chat platforms, on the other hand, are commercially licensed and typically offer a more user-friendly experience, along with dedicated support and maintenance. They may also include advanced features and integrations that are not available in open-source solutions. Selecting between the two depends on your team's technical capabilities and the amount of customization needed.
Choosing the Right Live Voice Chat Platform
Selecting the appropriate live voice chat platform requires careful consideration of your specific needs, budget, and technical capabilities. A variety of live voice chat platforms are available, each with its own strengths and weaknesses. Comparing live voice chat software across different vendors will significantly help determine the best fit.
Key Features to Consider
When evaluating live voice chat platforms, several key features should be taken into account:
- Audio Quality: Ensure the platform delivers clear and crisp audio quality, even under varying network conditions. Look for support for advanced audio codecs and noise cancellation features.
- Latency: Low latency is crucial for real-time communication. A platform with minimal delay will provide a more natural and responsive conversation experience.
- Group Call Support: If you need to support group conversations, ensure the platform can handle multiple participants without performance degradation. The number of concurrent users supported is a key metric.
- Screen Sharing: The ability to share screens can be invaluable for collaboration and presentations.
- Recording: The ability to record voice chat sessions can be useful for training, documentation, and compliance purposes.
- Moderation Tools: For larger groups, moderation tools are essential for managing participants and preventing disruptive behavior.
- Cross-Platform Compatibility: Choose a platform that supports the devices and operating systems your users are likely to use.
Scalability and Integration Capabilities
Scalability is a critical factor, especially if you anticipate a growing user base. The platform should be able to handle increasing traffic and user load without compromising performance. Consider how easily the live voice chat system can be integrated with existing platforms, such as CRM systems, help desk software, or collaboration tools. A well-designed live voice chat API can significantly simplify the integration process.
Security and Privacy Considerations
Security and privacy are paramount when implementing live voice chat. Ensure the platform employs robust encryption protocols to protect audio data in transit and at rest. Look for compliance with relevant data privacy regulations, such as GDPR and HIPAA. Transparency regarding data handling practices is also crucial. Analyze how the platform handles user authentication and authorization to prevent unauthorized access to voice chat sessions.
Implementing Live Voice Chat: A Technical Overview
Implementing live voice chat involves setting up the necessary infrastructure, integrating the platform with your existing systems, and addressing scalability and performance considerations. Below is a technical walkthrough.
Setting Up a Basic Live Voice Chat System
Here's an example using WebRTC, a powerful technology for real-time communication:
1// Client-side code (JavaScript)
2const peerConnection = new RTCPeerConnection();
3
4// Handle incoming media streams
5peerConnection.ontrack = (event) => {
6 const remoteStream = event.streams[0];
7 // Display the remote stream in a video element
8 const remoteVideo = document.getElementById('remoteVideo');
9 remoteVideo.srcObject = remoteStream;
10};
11
12// Get local media stream (audio and video)
13navigator.mediaDevices.getUserMedia({ audio: true, video: false })
14 .then((stream) => {
15 // Add local stream to the peer connection
16 stream.getTracks().forEach(track => peerConnection.addTrack(track, stream));
17 // Display the local stream in a video element (optional)
18 const localVideo = document.getElementById('localVideo');
19 localVideo.srcObject = stream;
20 })
21 .catch((error) => {
22 console.error('Error getting user media:', error);
23 });
24
25// Signaling (SDP exchange - handled by a server)
26// ... (code for creating and exchanging SDP offers and answers)
27
This code snippet showcases the fundamental aspects of initiating a peer connection, acquiring local media, and managing remote media streams using WebRTC. Signaling, crucial for negotiating the connection between peers, requires a server-side component to facilitate the exchange of Session Description Protocol (SDP) offers and answers.
Integrating Live Voice Chat with Existing Platforms
Most live voice chat platforms offer APIs that allow developers to seamlessly integrate them with existing applications and services. These APIs typically provide methods for creating voice chat rooms, managing participants, sending and receiving audio data, and handling events. Conceptual API integration example:
1# Python API integration example (conceptual)
2import requests
3
4API_KEY = "YOUR_API_KEY"
5BASE_URL = "https://api.example-voice-chat.com"
6
7def create_room(room_name):
8 headers = {"Authorization": f"Bearer {API_KEY}"}
9 data = {"name": room_name}
10 response = requests.post(f"{BASE_URL}/rooms", headers=headers, json=data)
11 return response.json()
12
13def add_user_to_room(room_id, user_id):
14 headers = {"Authorization": f"Bearer {API_KEY}"}
15 data = {"user_id": user_id}
16 response = requests.post(f"{BASE_URL}/rooms/{room_id}/users", headers=headers, json=data)
17 return response.json()
18
19# Example usage
20room = create_room("Developers Chat")
21add_user_to_room(room["id"], "user123")
22
This Python code demonstrates a hypothetical API integration. Replace placeholders like
YOUR_API_KEY
and https://api.example-voice-chat.com
with real values from your chosen provider. This illustrates creating a room and adding a user using API calls. Ensure you handle error cases and authentication as specified in the API documentation.Handling Scalability and Performance Issues
As your user base grows, you'll need to address scalability and performance issues. Consider using a content delivery network (CDN) to distribute audio data and reduce latency. Optimize your server infrastructure to handle increased traffic. Implement load balancing to distribute requests across multiple servers. Monitor your system performance closely and identify potential bottlenecks. Utilizing technologies such as WebSockets for persistent connections and implementing efficient audio encoding techniques can significantly improve performance and reduce bandwidth consumption. For server-based solutions, consider auto-scaling capabilities offered by cloud providers to dynamically adjust resources based on demand. Implementing proper error handling and logging mechanisms is critical for diagnosing and resolving performance issues quickly.
Live Voice Chat Applications Across Industries
Live voice chat is transforming industries across the board, offering innovative solutions for communication and collaboration.
Customer Service and Support
Live voice chat enables businesses to provide instant and personalized support to their customers. It allows support agents to quickly address customer inquiries, resolve issues, and build stronger relationships. Voice chat for customer service results in increased customer satisfaction and improved brand loyalty. The immediacy and personal touch of voice communication can often resolve complex issues more effectively than email or text-based chat.
E-Learning and Online Education
Live voice chat enhances the learning experience by enabling real-time interaction between students and instructors. It facilitates discussions, Q&A sessions, and collaborative projects. Voice chat creates a more engaging and interactive learning environment, leading to improved student outcomes. Virtual classrooms benefit greatly from the ability to have spontaneous voice-based conversations, mirroring the dynamics of a physical classroom.
Gaming and Entertainment
Live voice chat is an essential component of online gaming, allowing players to communicate and coordinate strategies in real-time. It enhances the immersive experience and fosters teamwork. Voice chat for gaming provides a competitive edge and makes online gaming more enjoyable. Integration with streaming platforms allows gamers to share their gameplay and commentary with a wider audience, further enhancing the social and entertainment aspects of gaming.
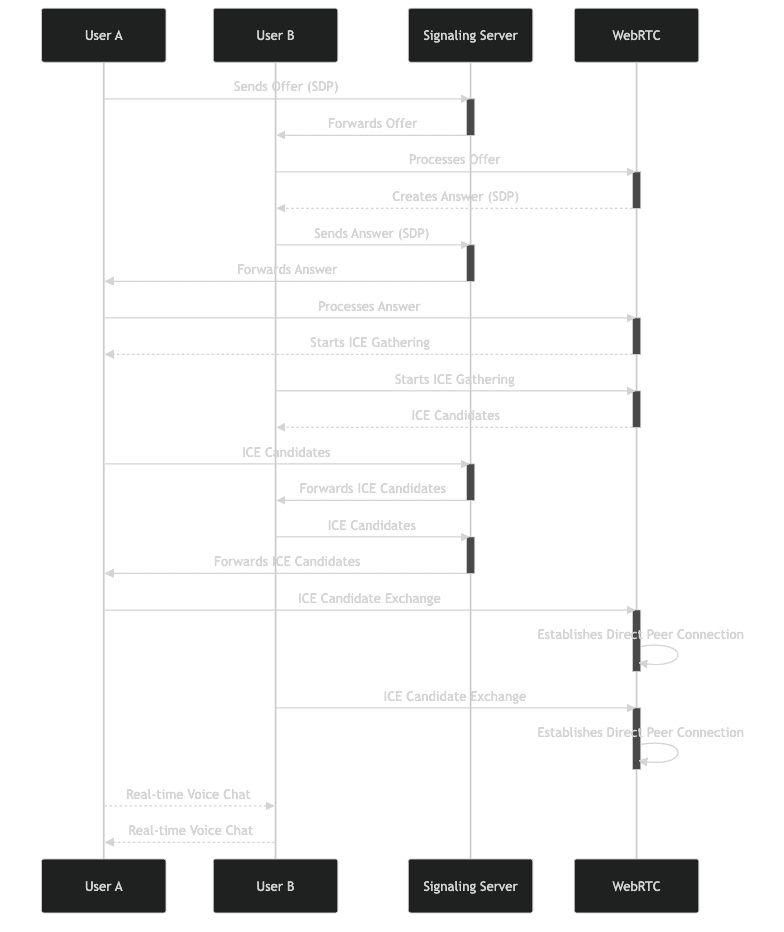
The Future of Live Voice Chat
The future of live voice chat is bright, with ongoing advancements in technology and expanding use cases.
Advancements in WebRTC and VoIP Technology
WebRTC and VoIP technologies are constantly evolving, offering improved audio quality, lower latency, and enhanced security. Future advancements will likely focus on optimizing performance for mobile devices and low-bandwidth environments. The integration of new audio codecs and noise cancellation algorithms will further enhance the user experience. Innovations in network protocols will enable more reliable and scalable live voice chat solutions.
Integration with AI and Machine Learning
AI and machine learning are poised to revolutionize live voice chat. AI-powered noise cancellation can eliminate background noise and improve audio clarity. Machine learning algorithms can analyze voice data to detect sentiment, identify speakers, and provide real-time translation. AI-driven chatbots can assist with customer support, routing calls to the appropriate agents and providing automated responses to common inquiries. The integration of AI and machine learning will make live voice chat more intelligent, efficient, and user-friendly.
Conclusion: The Power of Real-Time Communication
Live voice chat is a powerful tool that can transform the way we communicate and collaborate. Whether it's for customer service, online education, gaming, or enterprise communication, the ability to connect with others in real-time using voice offers numerous benefits. By choosing the right platform, implementing it effectively, and staying abreast of future trends, developers can leverage the power of real-time voice chat to create innovative and engaging experiences. The future of communication is undeniably interactive and immediate, making it more important than ever for developers to understand live voice chat.
- Learn more about WebRTC:
To understand the underlying technology powering many live voice chat solutions, explore the official WebRTC website.
- Explore the possibilities of VoIP:
Delve into the broader context of Voice over Internet Protocol (VoIP) technologies and their impact on communication.
- Discover more about API integrations:
Learn how APIs facilitate the seamless connection between different software systems, enhancing functionality.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ