What is WebTorrent WebRTC?
WebTorrent is a streaming torrent client that works in the browser and on Node.js. It allows you to download and share files quickly and efficiently using the BitTorrent protocol. WebRTC (Web Real-Time Communication) is a technology that enables peer-to-peer communication in web applications, allowing for real-time data transfer without the need for intermediaries.
When combined, WebTorrent and WebRTC provide a powerful solution for video streaming. WebTorrent leverages WebRTC to facilitate direct, peer-to-peer connections between users, enabling efficient and decentralized video streaming. This means that users can stream videos directly from each other, reducing the load on central servers and improving the streaming experience.
Benefits of Using WebTorrent WebRTC in Video Streaming
Efficiency
WebTorrent WebRTC allows for direct data transfer between peers, which means faster download and streaming speeds. As more peers join the network, the available bandwidth for streaming increases, leading to a more efficient distribution of video content.
Decentralization
One of the main advantages of using WebTorrent with WebRTC is decentralization. Unlike traditional video streaming services that rely on centralized servers, WebTorrent enables a distributed network of peers to share video content. This reduces the reliance on central servers and makes the system more robust and scalable.
Real-time Data Transfer
WebRTC facilitates real-time communication between peers. This makes it possible to stream video content with minimal latency, providing a smooth and uninterrupted viewing experience. Real-time data transfer is especially important for applications that require live streaming, such as video conferencing or live events.
By leveraging the strengths of both WebTorrent and WebRTC, developers can create video streaming applications that are efficient, scalable, and resilient. In the following sections, we'll dive into the specifics of setting up a WebTorrent WebRTC video streaming application using JS/Node.js.
Let`s Start to Build WebTorrent WebRTC Video Streaming Application
In this section, we'll guide you through the initial setup and development of a WebTorrent WebRTC video streaming application using JS/Node.js. We'll cover creating a new WebTorrent app, installing necessary libraries, structuring your project, and understanding the app architecture.
Create a New WebTorrent App
Setting Up the Development Environment
To get started, ensure you have Node.js and npm (Node Package Manager) installed on your machine. You can download and install them from the
official Node.js website
.Initializing a New Node.js Project
Open your terminal and create a new directory for your project. Navigate into this directory and initialize a new Node.js project by running:
bash
1mkdir webtorrent-webrtc-app
2cd webtorrent-webrtc-app
3npm init -y
This command creates a
package.json
file, which will manage your project's dependencies and scripts.Install WebTorrent
[a] Installing WebTorrent Library
Next, you'll need to install the WebTorrent library. Run the following command in your terminal:
bash
1npm install webtorrent
This command installs the WebTorrent library and adds it to your project's dependencies in
package.json
.[b] Basic Usage Example
To verify the installation, create an
index.js
file in your project directory and add the following code:JavaScript
1const WebTorrent = require('webtorrent');
2
3const client = new WebTorrent();
4
5client.on('error', (err) => {
6 console.error('Error: ', err.message);
7});
8
9// Example torrent file or magnet link
10const torrentId = 'https://webtorrent.io/torrents/sintel.torrent';
11
12client.add(torrentId, (torrent) => {
13 console.log('Client is downloading:', torrent.infoHash);
14
15 torrent.files.forEach((file) => {
16 file.appendTo('body');
17 });
18});
Run the script using:
bash
1node index.js
This script initializes a WebTorrent client, adds a torrent, and appends the file content to the body of an HTML document.
Structure of the Project
Overview of Project Files and Directories
A well-organized project structure is crucial for maintainability and scalability. Here's a suggested structure for your WebTorrent WebRTC app:
1webtorrent-webrtc-app/
2│
3├── public/
4│ ├── index.html
5│ ├── styles.css
6│ └── app.js
7│
8├── src/
9│ ├── index.js
10│ ├── client.js
11│ └── server.js
12│
13├── package.json
14└── README.md
public/
: Contains static files like HTML, CSS, and client-side JavaScript.src/
: Contains server-side JavaScript files and application logic.package.json
: Manages project dependencies and scripts.README.md
: Provides information about the project.
WebTorrent WebRTC App Architecture
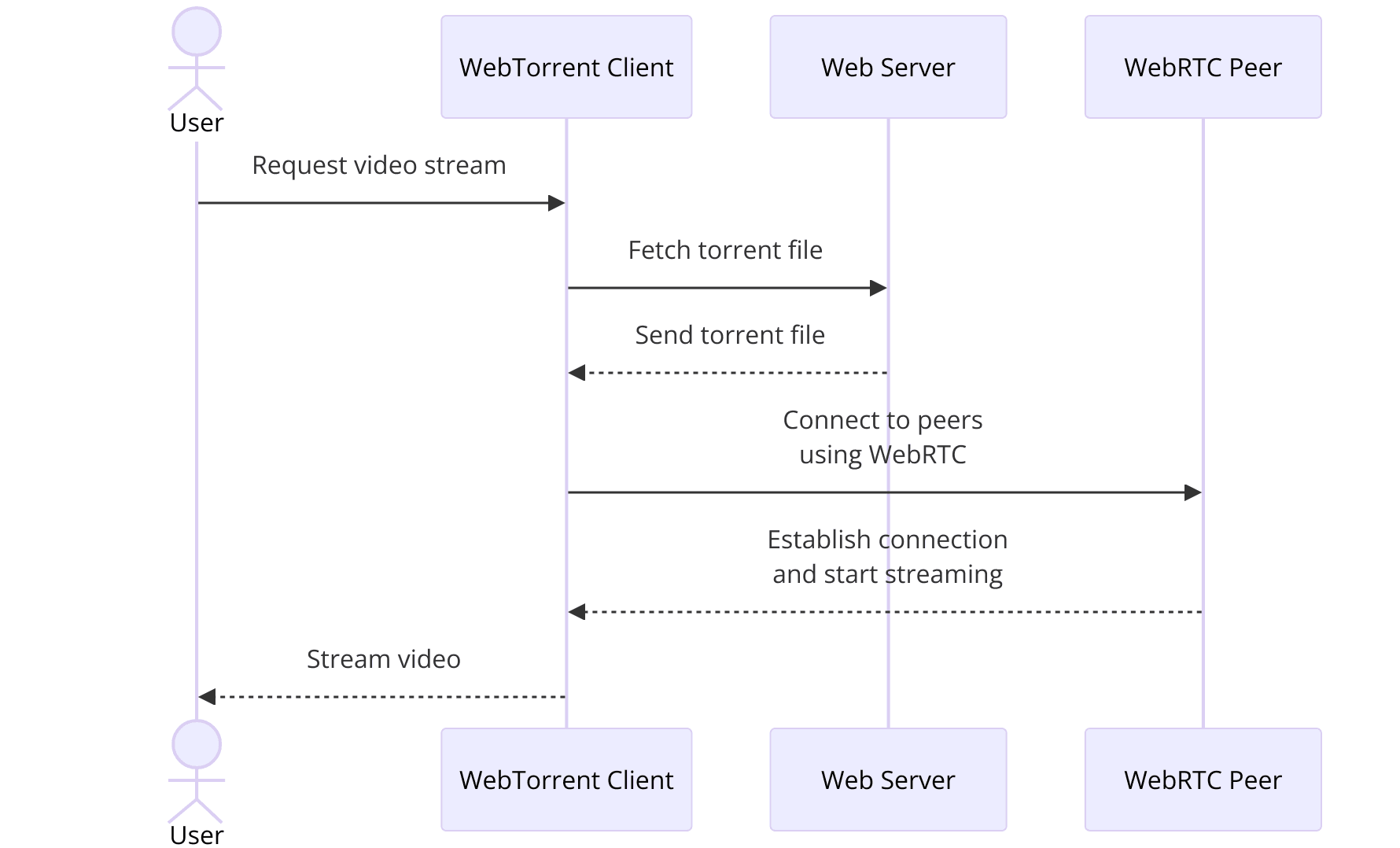
Explanation of the App's Architecture
Our WebTorrent WebRTC app will follow a client-server architecture. The server, implemented with Node.js, will manage WebRTC signaling and WebTorrent seeding. The client will handle the user interface and establish WebRTC connections with other peers.
Interaction Between Client and Server
- Client: Users interact with the client application through a web interface. The client initializes WebRTC connections and connects to the WebTorrent network to download and stream video content.
- Server: The server facilitates WebRTC signaling, manages peer connections, and seeds the initial video content using WebTorrent.
By setting up this architecture, we ensure that our application can efficiently handle multiple users and provide a seamless video streaming experience.
In the next section, we'll delve into the specifics of setting up
index.js
to initialize our WebTorrent client and server components.Step 1: Get Started with index.js
In this section, we will set up the foundational code for our WebTorrent WebRTC application. We will initialize a Node.js project, import the necessary libraries, and set up a basic WebTorrent client in
index.js
.Setting Up index.js
[a] Initializing a Node.js Project
Before diving into the code, ensure your project is properly initialized. If you haven't done so already, run the following commands in your project directory:
bash
1mkdir webtorrent-webrtc-app
2cd webtorrent-webrtc-app
3npm init -y
This creates a
package.json
file that will manage your project's dependencies and configurations.[b] Importing Necessary Libraries
In your project directory, create a new file named
index.js
. This file will serve as the entry point for your Node.js application. Start by importing the required libraries. We will use WebTorrent
for torrent management and http
for creating a basic server.JavaScript
1// index.js
2
3const WebTorrent = require('webtorrent');
4const http = require('http');
5const fs = require('fs');
6const path = require('path');
7
8// Create a WebTorrent client instance
9const client = new WebTorrent();
10
11// Error handling
12client.on('error', (err) => {
13 console.error('Error: ', err.message);
14});
15
16// Basic HTTP server setup
17const server = http.createServer((req, res) => {
18 if (req.url === '/') {
19 fs.readFile(path.join(__dirname, 'public', 'index.html'), (err, content) => {
20 if (err) throw err;
21 res.writeHead(200, { 'Content-Type': 'text/html' });
22 res.end(content, 'utf8');
23 });
24 }
25});
26
27// Server listens on port 3000
28const PORT = process.env.PORT || 3000;
29server.listen(PORT, () => {
30 console.log(`Server running on port ${PORT}`);
31});
[c] Basic Setup for a WebTorrent Client
Now, let’s add some code to
index.js
to set up a basic WebTorrent client that will download and stream a torrent file.JavaScript
1// Example torrent file or magnet link
2const torrentId = 'https://webtorrent.io/torrents/sintel.torrent';
3
4client.add(torrentId, (torrent) => {
5 console.log('Client is downloading:', torrent.infoHash);
6
7 // Stream the files contained in the torrent
8 torrent.files.forEach((file) => {
9 file.getBuffer((err, buffer) => {
10 if (err) throw err;
11 console.log(`Downloaded ${file.name}`);
12 });
13 });
14});
In this snippet, we specify a torrent file URL and use the WebTorrent client to add and download the torrent. The
add
method accepts a torrent ID (which can be a magnet link, torrent file URL, or info hash) and a callback function that executes when the torrent starts downloading. The callback function logs the torrent's info hash and iterates over the files in the torrent, streaming each one to a buffer.[d] Running the Script
To run your script, ensure you have the necessary directories and files in place. Create a
public
directory and an index.html
file inside it:HTML
1<!-- public/index.html -->
2
3<!DOCTYPE html>
4<html lang="en">
5<head>
6 <meta charset="UTF-8">
7 <meta name="viewport" content="width=device-width, initial-scale=1.0">
8 <title>WebTorrent WebRTC App</title>
9</head>
10<body>
11 <h1>Welcome to WebTorrent WebRTC App</h1>
12 <p>Streaming Video...</p>
13</body>
14</html>
Now, start your Node.js server by running:
bash
1node index.js
Open your browser and navigate to
http://localhost:3000
. You should see a welcome message indicating that the server is running and the WebTorrent client is ready to stream video.With the basic setup in place, you now have a foundation to build upon. In the next section, we will design the user interface and wireframe all the components necessary for our WebTorrent WebRTC application.
Step 2: Wireframe All the Components
In this section, we will design the user interface (UI) for our WebTorrent WebRTC application. We'll create the necessary HTML and CSS to structure the layout and style of our application. Additionally, we'll set up client-server communication to handle WebRTC connections.
Designing the UI
[a] Overview of UI Components
Our application will have a simple interface consisting of a video player, controls, and a section for displaying participants. Here’s a breakdown of the UI components:
- Video Player: For streaming the video.
- Controls: For playing, pausing, and seeking the video.
- Participants Section: For displaying other peers connected to the stream.
[b] HTML Structure
Create an
index.html
file inside the public
directory with the following content:HTML
1<!-- public/index.html -->
2
3<!DOCTYPE html>
4<html lang="en">
5<head>
6 <meta charset="UTF-8">
7 <meta name="viewport" content="width=device-width, initial-scale=1.0">
8 <title>WebTorrent WebRTC App</title>
9 <link rel="stylesheet" href="styles.css">
10</head>
11<body>
12 <div class="container">
13 <h1>WebTorrent WebRTC Video Streaming</h1>
14 <div class="video-container">
15 <video id="videoPlayer" controls></video>
16 </div>
17 <div class="controls">
18 <button id="playPauseBtn">Play/Pause</button>
19 <button id="fullScreenBtn">Fullscreen</button>
20 </div>
21 <div class="participants">
22 <h2>Participants</h2>
23 <ul id="participantsList"></ul>
24 </div>
25 </div>
26 <script src="app.js"></script>
27</body>
28</html>
This HTML file sets up a basic structure with a video player, controls, and a participants list.
[c] CSS Styling
Create a
styles.css
file inside the public
directory to style the UI components:CSS
1/* public/styles.css */
2
3body {
4 font-family: Arial, sans-serif;
5 background-color: #f0f0f0;
6 color: #333;
7 margin: 0;
8 padding: 0;
9 display: flex;
10 justify-content: center;
11 align-items: center;
12 height: 100vh;
13}
14
15.container {
16 max-width: 800px;
17 margin: 0 auto;
18 padding: 20px;
19 background: #fff;
20 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
21 border-radius: 8px;
22}
23
24h1 {
25 text-align: center;
26}
27
28.video-container {
29 position: relative;
30 width: 100%;
31 padding-bottom: 56.25%; /* 16:9 aspect ratio */
32 height: 0;
33 margin-bottom: 20px;
34}
35
36video {
37 position: absolute;
38 top: 0;
39 left: 0;
40 width: 100%;
41 height: 100%;
42 border-radius: 8px;
43}
44
45.controls {
46 display: flex;
47 justify-content: center;
48 gap: 10px;
49 margin-bottom: 20px;
50}
51
52button {
53 padding: 10px 20px;
54 font-size: 16px;
55 border: none;
56 border-radius: 4px;
57 cursor: pointer;
58}
59
60button:hover {
61 background-color: #ddd;
62}
63
64.participants {
65 text-align: center;
66}
67
68.participants ul {
69 list-style: none;
70 padding: 0;
71}
72
73.participants li {
74 padding: 5px 0;
75}
This CSS file styles the video player, controls, and participants list to create a clean and user-friendly interface.
Client-Server Communication
[a] Setting Up WebRTC Connections
We need to establish WebRTC connections between peers for video streaming. In the
public
directory, create a file named app.js
to handle client-side logic:JavaScript
1// public/app.js
2
3// Initialize WebTorrent client
4const client = new WebTorrent();
5
6const videoPlayer = document.getElementById('videoPlayer');
7const playPauseBtn = document.getElementById('playPauseBtn');
8const fullScreenBtn = document.getElementById('fullScreenBtn');
9
10// Example torrent file or magnet link
11const torrentId = 'https://webtorrent.io/torrents/sintel.torrent';
12
13client.add(torrentId, (torrent) => {
14 const file = torrent.files.find(file => file.name.endsWith('.mp4'));
15 file.renderTo(videoPlayer);
16
17 playPauseBtn.addEventListener('click', () => {
18 if (videoPlayer.paused) {
19 videoPlayer.play();
20 } else {
21 videoPlayer.pause();
22 }
23 });
24
25 fullScreenBtn.addEventListener('click', () => {
26 if (videoPlayer.requestFullscreen) {
27 videoPlayer.requestFullscreen();
28 } else if (videoPlayer.mozRequestFullScreen) { /* Firefox */
29 videoPlayer.mozRequestFullScreen();
30 } else if (videoPlayer.webkitRequestFullscreen) { /* Chrome, Safari & Opera */
31 videoPlayer.webkitRequestFullscreen();
32 } else if (videoPlayer.msRequestFullscreen) { /* IE/Edge */
33 videoPlayer.msRequestFullscreen();
34 }
35 });
36});
37
38// Handle participants (for demonstration purposes)
39const participantsList = document.getElementById('participantsList');
40const peers = ['Peer 1', 'Peer 2', 'Peer 3'];
41peers.forEach(peer => {
42 const li = document.createElement('li');
43 li.textContent = peer;
44 participantsList.appendChild(li);
45});
This script initializes a WebTorrent client, adds a torrent, and renders the video to the video player element. It also sets up event listeners for the play/pause and fullscreen buttons and populates a sample participants list.
With the UI components and client-side logic in place, we have a basic structure for our WebTorrent WebRTC application. In the next section, we will implement the join screen to handle user input and initialize WebRTC connections.
Step 3: Implement Join Screen
In this section, we'll create a join screen for our WebTorrent WebRTC application. The join screen will allow users to enter a room and connect with other participants for video streaming. We'll add the necessary HTML, CSS, and JavaScript to handle user input and initialize WebRTC connections.
Creating a Join Screen
[a] HTML Structure for the Join Screen
Update your
index.html
file to include the join screen. We'll add a form that allows users to enter a room name and join the session.HTML
1<!-- public/index.html -->
2
3<!DOCTYPE html>
4<html lang="en">
5<head>
6 <meta charset="UTF-8">
7 <meta name="viewport" content="width=device-width, initial-scale=1.0">
8 <title>WebTorrent WebRTC App</title>
9 <link rel="stylesheet" href="styles.css">
10</head>
11<body>
12 <div class="container">
13 <h1>WebTorrent WebRTC Video Streaming</h1>
14
15 <div id="joinScreen">
16 <h2>Join a Room</h2>
17 <form id="joinForm">
18 <input type="text" id="roomInput" placeholder="Enter Room Name" required>
19 <button type="submit">Join</button>
20 </form>
21 </div>
22
23 <div id="videoScreen" style="display: none;">
24 <div class="video-container">
25 <video id="videoPlayer" controls></video>
26 </div>
27 <div class="controls">
28 <button id="playPauseBtn">Play/Pause</button>
29 <button id="fullScreenBtn">Fullscreen</button>
30 </div>
31 <div class="participants">
32 <h2>Participants</h2>
33 <ul id="participantsList"></ul>
34 </div>
35 </div>
36 </div>
37 <script src="app.js"></script>
38</body>
39</html>
This HTML adds a
joinScreen
div with a form for entering the room name. When the user submits the form, they will be redirected to the videoScreen
div.[b] CSS Styling for a User-Friendly Interface
Update your
styles.css
file to style the join screen and the video screen.CSS
1/* public/styles.css */
2
3body {
4 font-family: Arial, sans-serif;
5 background-color: #f0f0f0;
6 color: #333;
7 margin: 0;
8 padding: 0;
9 display: flex;
10 justify-content: center;
11 align-items: center;
12 height: 100vh;
13}
14
15.container {
16 max-width: 800px;
17 margin: 0 auto;
18 padding: 20px;
19 background: #fff;
20 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
21 border-radius: 8px;
22}
23
24h1, h2 {
25 text-align: center;
26}
27
28#joinScreen {
29 display: flex;
30 flex-direction: column;
31 align-items: center;
32}
33
34#joinForm {
35 display: flex;
36 flex-direction: column;
37 align-items: center;
38}
39
40#roomInput {
41 padding: 10px;
42 font-size: 16px;
43 margin-bottom: 10px;
44 width: 200px;
45 border: 1px solid #ccc;
46 border-radius: 4px;
47}
48
49#joinForm button {
50 padding: 10px 20px;
51 font-size: 16px;
52 border: none;
53 border-radius: 4px;
54 cursor: pointer;
55 background-color: #007bff;
56 color: #fff;
57}
58
59#joinForm button:hover {
60 background-color: #0056b3;
61}
62
63.video-container {
64 position: relative;
65 width: 100%;
66 padding-bottom: 56.25%; /* 16:9 aspect ratio */
67 height: 0;
68 margin-bottom: 20px;
69}
70
71video {
72 position: absolute;
73 top: 0;
74 left: 0;
75 width: 100%;
76 height: 100%;
77 border-radius: 8px;
78}
79
80.controls {
81 display: flex;
82 justify-content: center;
83 gap: 10px;
84 margin-bottom: 20px;
85}
86
87button {
88 padding: 10px 20px;
89 font-size: 16px;
90 border: none;
91 border-radius: 4px;
92 cursor: pointer;
93}
94
95button:hover {
96 background-color: #ddd;
97}
98
99.participants {
100 text-align: center;
101}
102
103.participants ul {
104 list-style: none;
105 padding: 0;
106}
107
108.participants li {
109 padding: 5px 0;
110}
This CSS styles the join screen and the video screen to ensure a user-friendly interface.
[c] JavaScript to Handle User Input
Update your
app.js
file to handle form submission and switch between screens.JavaScript
1// public/app.js
2
3// Initialize WebTorrent client
4const client = new WebTorrent();
5
6const videoPlayer = document.getElementById('videoPlayer');
7const playPauseBtn = document.getElementById('playPauseBtn');
8const fullScreenBtn = document.getElementById('fullScreenBtn');
9const joinScreen = document.getElementById('joinScreen');
10const videoScreen = document.getElementById('videoScreen');
11const joinForm = document.getElementById('joinForm');
12const roomInput = document.getElementById('roomInput');
13const participantsList = document.getElementById('participantsList');
14
15// Example torrent file or magnet link
16const torrentId = 'https://webtorrent.io/torrents/sintel.torrent';
17
18// Handle join form submission
19joinForm.addEventListener('submit', (event) => {
20 event.preventDefault();
21 const roomName = roomInput.value.trim();
22 if (roomName) {
23 joinRoom(roomName);
24 }
25});
26
27// Function to join a room
28function joinRoom(roomName) {
29 joinScreen.style.display = 'none';
30 videoScreen.style.display = 'block';
31 console.log(`Joined room: ${roomName}`);
32
33 // Add the torrent and stream the video
34 client.add(torrentId, (torrent) => {
35 const file = torrent.files.find(file => file.name.endsWith('.mp4'));
36 file.renderTo(videoPlayer);
37
38 playPauseBtn.addEventListener('click', () => {
39 if (videoPlayer.paused) {
40 videoPlayer.play();
41 } else {
42 videoPlayer.pause();
43 }
44 });
45
46 fullScreenBtn.addEventListener('click', () => {
47 if (videoPlayer.requestFullscreen) {
48 videoPlayer.requestFullscreen();
49 } else if (videoPlayer.mozRequestFullScreen) { /* Firefox */
50 videoPlayer.mozRequestFullScreen();
51 } else if (videoPlayer.webkitRequestFullscreen) { /* Chrome, Safari & Opera */
52 videoPlayer.webkitRequestFullscreen();
53 } else if (videoPlayer.msRequestFullscreen) { /* IE/Edge */
54 videoPlayer.msRequestFullscreen();
55 }
56 });
57 });
58
59 // Handle participants (for demonstration purposes)
60 const peers = ['Peer 1', 'Peer 2', 'Peer 3'];
61 peers.forEach(peer => {
62 const li = document.createElement('li');
63 li.textContent = peer;
64 participantsList.appendChild(li);
65 });
66}
This script handles the form submission by preventing the default form action and calling the
joinRoom
function with the entered room name. The joinRoom
function hides the join screen, shows the video screen, and initializes the WebTorrent client to stream the video. It also sets up the video controls and populates a sample participants list.With the join screen implemented, users can now enter a room name and connect to the video streaming session. In the next section, we will implement the video controls to enhance the user experience.
Step 4: Implement Controls
In this section, we will enhance our WebTorrent WebRTC application by implementing video controls. These controls will include play/pause functionality, volume control, and fullscreen capabilities. We will also integrate these controls with the WebTorrent streaming functionality.
Developing Video Controls
Play/Pause Functionality
First, let's ensure that the play/pause button works correctly. This functionality has already been partially implemented, but we'll refine it and add more controls.
Update the event listener for the play/pause button in your
app.js
file:JavaScript
1// public/app.js
2
3// Existing code...
4
5playPauseBtn.addEventListener('click', () => {
6 if (videoPlayer.paused) {
7 videoPlayer.play();
8 playPauseBtn.textContent = 'Pause';
9 } else {
10 videoPlayer.pause();
11 playPauseBtn.textContent = 'Play';
12 }
13});
This script updates the button text based on the current state of the video player.
Volume Control
Next, we'll add a volume control slider to adjust the video volume. Update your
index.html
to include the volume control:HTML
1<!-- public/index.html -->
2
3<!-- Existing code... -->
4
5<div class="controls">
6 <button id="playPauseBtn">Play</button>
7 <button id="fullScreenBtn">Fullscreen</button>
8 <input type="range" id="volumeControl" min="0" max="1" step="0.1" value="1">
9</div>
Then, add an event listener for the volume control in
app.js
:JavaScript
1// public/app.js
2
3const volumeControl = document.getElementById('volumeControl');
4
5volumeControl.addEventListener('input', () => {
6 videoPlayer.volume = volumeControl.value;
7});
This script allows users to adjust the video volume using the range input slider.
Fullscreen Control
The fullscreen functionality is already implemented but can be improved for better cross-browser compatibility. Update the event listener for the fullscreen button:
JavaScript
1// public/app.js
2
3fullScreenBtn.addEventListener('click', () => {
4 if (!document.fullscreenElement) {
5 if (videoPlayer.requestFullscreen) {
6 videoPlayer.requestFullscreen();
7 } else if (videoPlayer.mozRequestFullScreen) { /* Firefox */
8 videoPlayer.mozRequestFullScreen();
9 } else if (videoPlayer.webkitRequestFullscreen) { /* Chrome, Safari & Opera */
10 videoPlayer.webkitRequestFullscreen();
11 } else if (videoPlayer.msRequestFullscreen) { /* IE/Edge */
12 videoPlayer.msRequestFullscreen();
13 }
14 } else {
15 if (document.exitFullscreen) {
16 document.exitFullscreen();
17 } else if (document.mozCancelFullScreen) { /* Firefox */
18 document.mozCancelFullScreen();
19 } else if (document.webkitExitFullscreen) { /* Chrome, Safari & Opera */
20 document.webkitExitFullscreen();
21 } else if (document.msExitFullscreen) { /* IE/Edge */
22 document.msExitFullscreen();
23 }
24 }
25});
This script ensures that the fullscreen functionality works across different browsers.
Styling the Controls
Update your
styles.css
file to style the new volume control slider:CSS
1/* public/styles.css */
2
3/* Existing code... */
4
5.controls {
6 display: flex;
7 justify-content: center;
8 gap: 10px;
9 margin-bottom: 20px;
10}
11
12input[type="range"] {
13 width: 150px;
14}
This CSS styles the controls section and the volume control slider.
Integrating Controls with WebTorrent
Ensure the video controls work seamlessly with the WebTorrent streaming functionality. The play/pause, volume, and fullscreen controls are now integrated, enhancing the user experience.
With these controls implemented, users can interact with the video stream more effectively. In the next section, we will implement the participant view to manage multiple video streams and display connected participants.
Step 5: Implement Participant View
In this section, we'll enhance our WebTorrent WebRTC application by implementing a participant view. This feature will allow users to see and manage multiple video streams from different participants. We'll create the necessary HTML, CSS, and JavaScript to handle real-time updates and display connected participants.
Displaying Participants
HTML and CSS for Participant Views
First, let's update the
index.html
file to include a section for displaying participant videos. We'll also update the CSS to style the participant view.Update your
index.html
:HTML
1<!-- public/index.html -->
2
3<!DOCTYPE html>
4<html lang="en">
5<head>
6 <meta charset="UTF-8">
7 <meta name="viewport" content="width=device-width, initial-scale=1.0">
8 <title>WebTorrent WebRTC App</title>
9 <link rel="stylesheet" href="styles.css">
10</head>
11<body>
12 <div class="container">
13 <h1>WebTorrent WebRTC Video Streaming</h1>
14
15 <div id="joinScreen">
16 <h2>Join a Room</h2>
17 <form id="joinForm">
18 <input type="text" id="roomInput" placeholder="Enter Room Name" required>
19 <button type="submit">Join</button>
20 </form>
21 </div>
22
23 <div id="videoScreen" style="display: none;">
24 <div class="video-container">
25 <video id="videoPlayer" controls></video>
26 </div>
27 <div class="controls">
28 <button id="playPauseBtn">Play</button>
29 <button id="fullScreenBtn">Fullscreen</button>
30 <input type="range" id="volumeControl" min="0" max="1" step="0.1" value="1">
31 </div>
32 <div class="participants">
33 <h2>Participants</h2>
34 <ul id="participantsList"></ul>
35 <div id="participantVideos"></div>
36 </div>
37 </div>
38 </div>
39 <script src="app.js"></script>
40</body>
41</html>
Add the following styles to your
styles.css
file to style the participant videos:CSS
1/* public/styles.css */
2
3/* Existing code... */
4
5.participants {
6 text-align: center;
7}
8
9.participants ul {
10 list-style: none;
11 padding: 0;
12}
13
14.participants li {
15 padding: 5px 0;
16}
17
18#participantVideos {
19 display: flex;
20 flex-wrap: wrap;
21 justify-content: center;
22 gap: 10px;
23 margin-top: 20px;
24}
25
26.participant-video {
27 width: 200px;
28 height: 150px;
29 background: #000;
30 border-radius: 8px;
31 overflow: hidden;
32}
33
34.participant-video video {
35 width: 100%;
36 height: 100%;
37}
These styles ensure that the participant videos are displayed in a grid layout, making it easy to see all connected participants.
JavaScript for Real-Time Updates
Now, let's update the
app.js
file to handle real-time updates and display participant videos.JavaScript
1// public/app.js
2
3// Existing code...
4
5const participantVideos = document.getElementById('participantVideos');
6
7// Function to add a participant video
8function addParticipantVideo(peerId) {
9 const participantVideoContainer = document.createElement('div');
10 participantVideoContainer.className = 'participant-video';
11 participantVideoContainer.id = `video-${peerId}`;
12
13 const videoElement = document.createElement('video');
14 videoElement.autoplay = true;
15 videoElement.controls = true;
16 participantVideoContainer.appendChild(videoElement);
17
18 participantVideos.appendChild(participantVideoContainer);
19
20 return videoElement;
21}
22
23// Example function to simulate adding participant videos
24function simulateParticipants() {
25 const peers = ['Peer 1', 'Peer 2', 'Peer 3'];
26 peers.forEach((peer, index) => {
27 const videoElement = addParticipantVideo(peer);
28 // For demonstration, we'll use the same video source for all participants
29 videoElement.src = 'https://www.w3schools.com/html/mov_bbb.mp4';
30 });
31}
32
33// Call simulateParticipants for demonstration purposes
34simulateParticipants();
35
36// Function to join a room
37function joinRoom(roomName) {
38 joinScreen.style.display = 'none';
39 videoScreen.style.display = 'block';
40 console.log(`Joined room: ${roomName}`);
41
42 // Add the torrent and stream the video
43 client.add(torrentId, (torrent) => {
44 const file = torrent.files.find(file => file.name.endsWith('.mp4'));
45 file.renderTo(videoPlayer);
46
47 playPauseBtn.addEventListener('click', () => {
48 if (videoPlayer.paused) {
49 videoPlayer.play();
50 playPauseBtn.textContent = 'Pause';
51 } else {
52 videoPlayer.pause();
53 playPauseBtn.textContent = 'Play';
54 }
55 });
56
57 fullScreenBtn.addEventListener('click', () => {
58 if (!document.fullscreenElement) {
59 if (videoPlayer.requestFullscreen) {
60 videoPlayer.requestFullscreen();
61 } else if (videoPlayer.mozRequestFullScreen) { /* Firefox */
62 videoPlayer.mozRequestFullScreen();
63 } else if (videoPlayer.webkitRequestFullscreen) { /* Chrome, Safari & Opera */
64 videoPlayer.webkitRequestFullscreen();
65 } else if (videoPlayer.msRequestFullscreen) { /* IE/Edge */
66 videoPlayer.msRequestFullscreen();
67 }
68 } else {
69 if (document.exitFullscreen) {
70 document.exitFullscreen();
71 } else if (document.mozCancelFullScreen) { /* Firefox */
72 document.mozCancelFullScreen();
73 } else if (document.webkitExitFullscreen) { /* Chrome, Safari & Opera */
74 document.webkitExitFullscreen();
75 } else if (document.msExitFullscreen) { /* IE/Edge */
76 document.msExitFullscreen();
77 }
78 }
79 });
80 });
81
82 // Handle participants (for demonstration purposes)
83 const peers = ['Peer 1', 'Peer 2', 'Peer 3'];
84 peers.forEach(peer => {
85 const li = document.createElement('li');
86 li.textContent = peer;
87 participantsList.appendChild(li);
88 });
89}
90
91// Function to handle new participant joining
92function handleNewParticipant(peerId) {
93 const videoElement = addParticipantVideo(peerId);
94 // Assume we get a stream from the peer
95 // For demonstration, we'll use a sample video source
96 videoElement.src = 'https://www.w3schools.com/html/mov_bbb.mp4';
97}
98
99// Example usage of handleNewParticipant
100handleNewParticipant('Peer 4');
This script adds a function
addParticipantVideo
to create and display a new video element for each participant. The simulateParticipants
function is a placeholder to demonstrate adding participant videos. The joinRoom
function handles joining a room and initializes the WebTorrent client. The handleNewParticipant
function simulates adding a new participant video.By implementing these changes, you now have a participant view that displays videos for all connected participants. This enhances the collaborative aspect of your WebTorrent WebRTC application by allowing users to see and manage multiple video streams in real-time.
In the next section, we will focus on running and testing the complete application to ensure everything works as expected.
Step 6: Run Your Code Now
In this section, we'll focus on running and testing your complete WebTorrent WebRTC application to ensure everything works as expected. We'll cover local testing, debugging common issues, and best practices for deploying your application.
Testing the Application
Before deploying your application, it's essential to test it locally to ensure all functionalities work correctly. Follow these steps to run your application:
Start the Node.js Server
Ensure you are in your project directory and start the Node.js server using the following command:
bash
1 node index.js
You should see a message indicating that the server is running on port 3000:
bash
1 Server running on port 3000
Open the Application in Your Browser
Open your browser and navigate to
http://localhost:3000
. You should see the join screen where you can enter a room name.Join a Room
Enter a room name and click the "Join" button. This action should switch to the video screen, start streaming the video, and display the participant view.
Debugging Common Issues
Here are some common issues you might encounter and how to resolve them:
Server Not Starting
- Ensure you have Node.js and npm installed.
- Check for syntax errors in your
index.js
or other JavaScript files. - Ensure all necessary dependencies are installed by running
npm install
.
Video Not Streaming
- Verify the torrent file or magnet link is correct and accessible.
- Check for errors in the browser console and server logs.
- Ensure your HTML and JavaScript files are correctly linked.
Participants Not Displaying
- Ensure the participant view is correctly implemented and styled.
- Check for errors in the JavaScript logic handling participant connections.
- Simulate participants correctly by calling the
simulateParticipants
function.
Deploying the Application
Deployment Options for Node.js Applications
Once you've tested your application locally, you can deploy it to a live environment. Here are some common deployment options for Node.js applications:
Heroku
- Install the Heroku CLI and log in.
- Create a new Heroku app and deploy your code using Git.
- Configure environment variables and scale your application.
Vercel
- Sign up for a Vercel account and link your Git repository.
- Deploy your Node.js application with automatic builds and deployments.
- Configure custom domains and environment variables.
DigitalOcean
- Create a Droplet (virtual private server) and configure your server environment.
- Deploy your Node.js application manually or using a CI/CD pipeline.
- Manage your server and scale resources as needed.
Best Practices for Production Deployment
- Security: Ensure your application is secure by implementing HTTPS, sanitizing user inputs, and using secure storage for sensitive data.
- Performance: Optimize your application for performance by using a CDN for static assets, enabling caching, and monitoring server load.
- Scalability: Design your application to scale by using microservices, load balancing, and containerization (e.g., Docker).
Conclusion
In this comprehensive guide, we've explored the process of creating a video streaming application using WebTorrent and WebRTC with JS/Node.js. We've covered everything from setting up the development environment and installing the necessary libraries to implementing essential features like video controls and participant views. By leveraging the strengths of both WebTorrent and WebRTC, you can build a robust, decentralized video streaming application that is efficient, scalable, and resilient.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ